728x90
2장
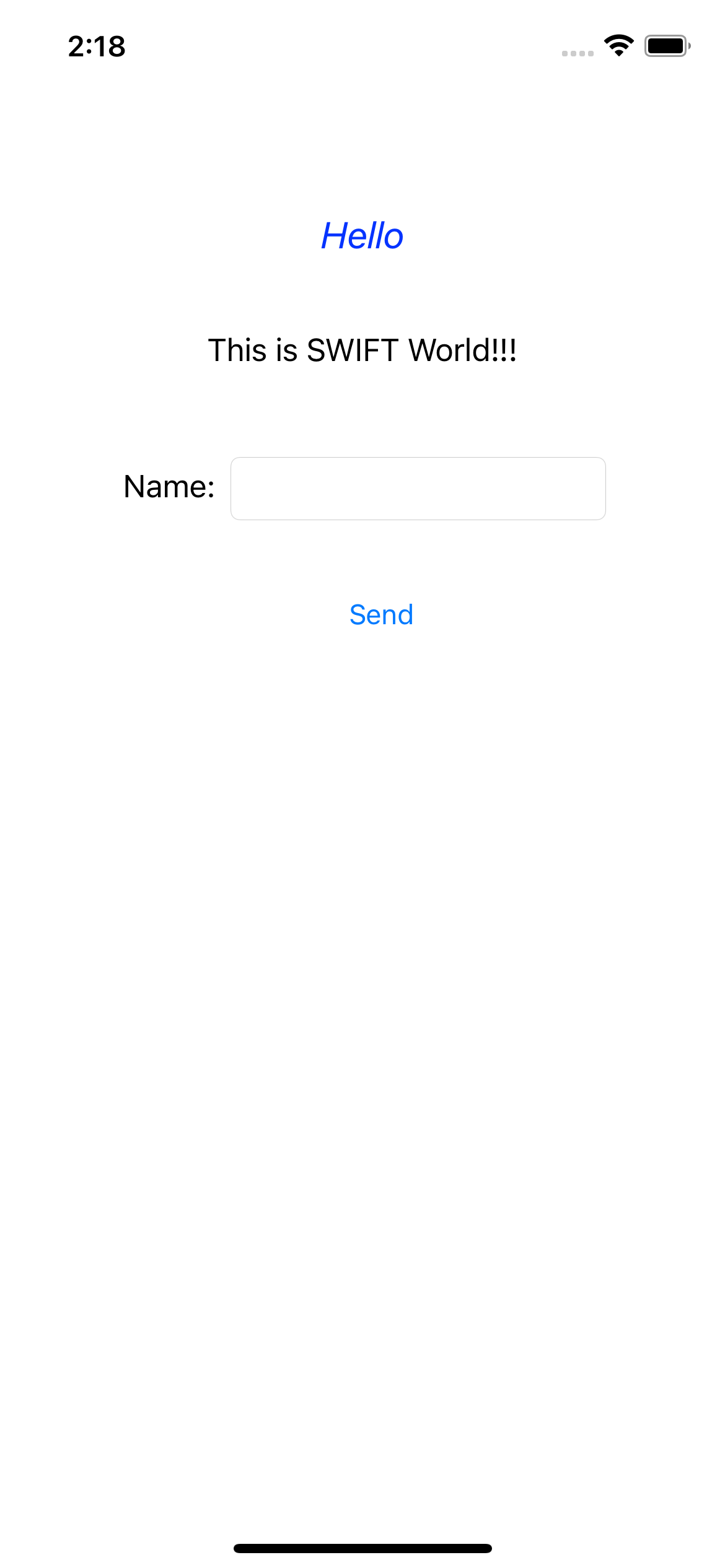
//
// ViewController.swift
// HelloWorld
//
// Created by Ho-Jeong Song on 2021/11/09.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var lblHello: UILabel!
@IBOutlet var txtName: UITextField!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnSend(_ sender: UIButton) {
lblHello.text = "Hello, " + txtName.text!
}
}
연결 관계
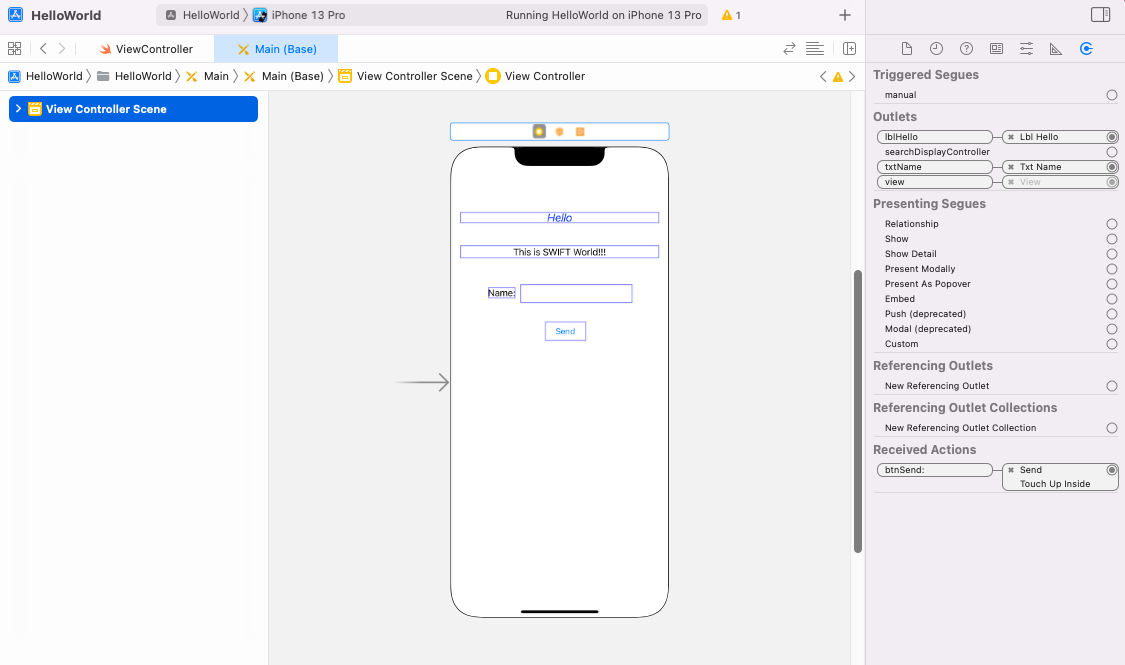
3강 본문 실습
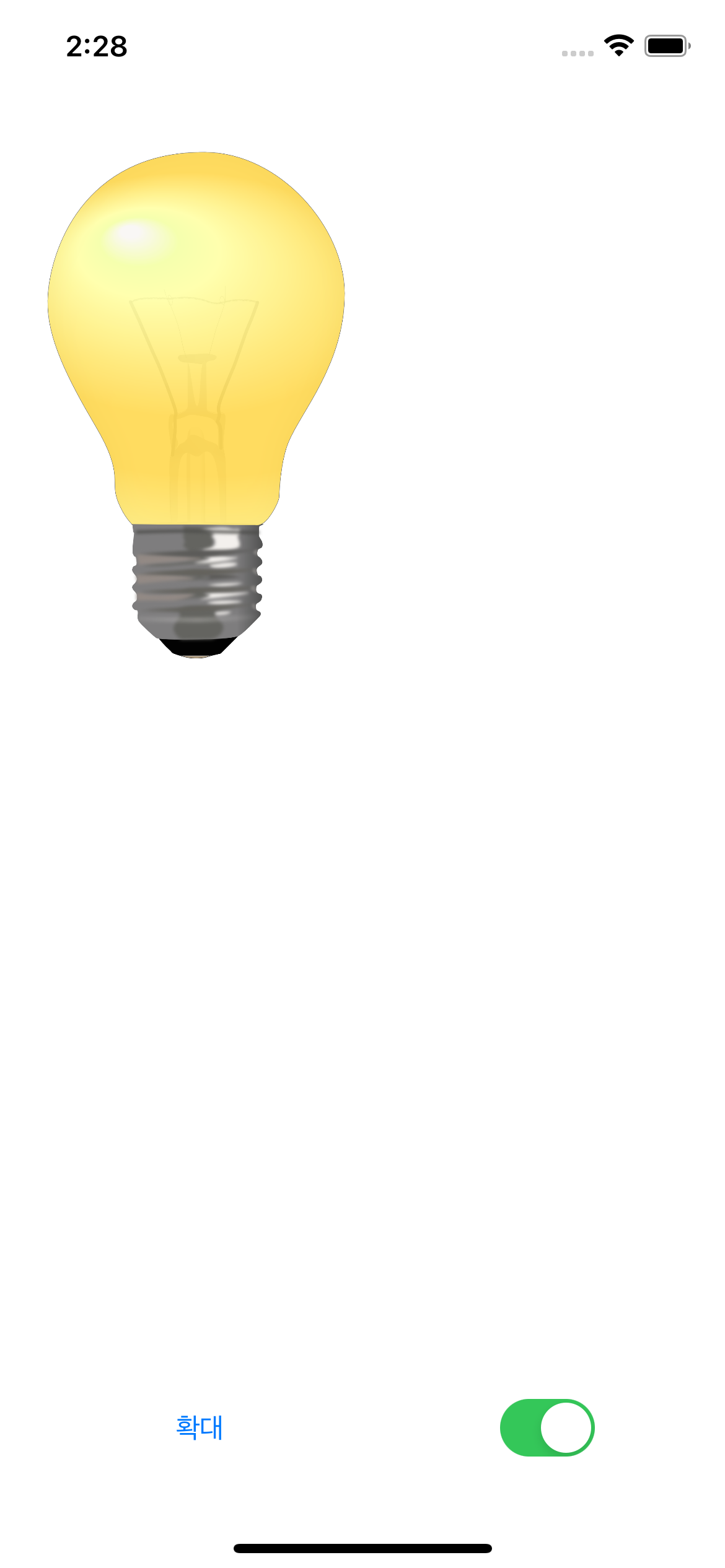
//
// ViewController.swift
// ImageView
//
// Created by Ho-Jeong Song on 2021/11/23.
//
import UIKit
class ViewController: UIViewController {
var isZoom = false
var imgOn: UIImage?
var imgOff: UIImage?
@IBOutlet var imgView: UIImageView!
@IBOutlet var btnResize: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
imgOn = UIImage(named: "lamp_on.png")
imgOff = UIImage(named: "lamp_off.png")
imgView.image = imgOn
}
@IBAction func btnResizeImage(_ sender: UIButton) {
let scale: CGFloat = 2.0
var newWidth: CGFloat, newHeight: CGFloat
if (isZoom) { // true
newWidth = imgView.frame.width / scale
newHeight = imgView.frame.height / scale
btnResize.setTitle("확대", for: .normal)
}
else { // false
newWidth = imgView.frame.width * scale
newHeight = imgView.frame.height * scale
btnResize.setTitle("축소", for: .normal)
}
imgView.frame.size = CGSize(width: newWidth, height: newHeight)
isZoom = !isZoom
}
@IBAction func switchImageOnOff(_ sender: UISwitch) {
if sender.isOn {
imgView.image = imgOn
} else {
imgView.image = imgOff
}
}
}
연결관계

4장 본문 실습 (데이트 타임 픽커)
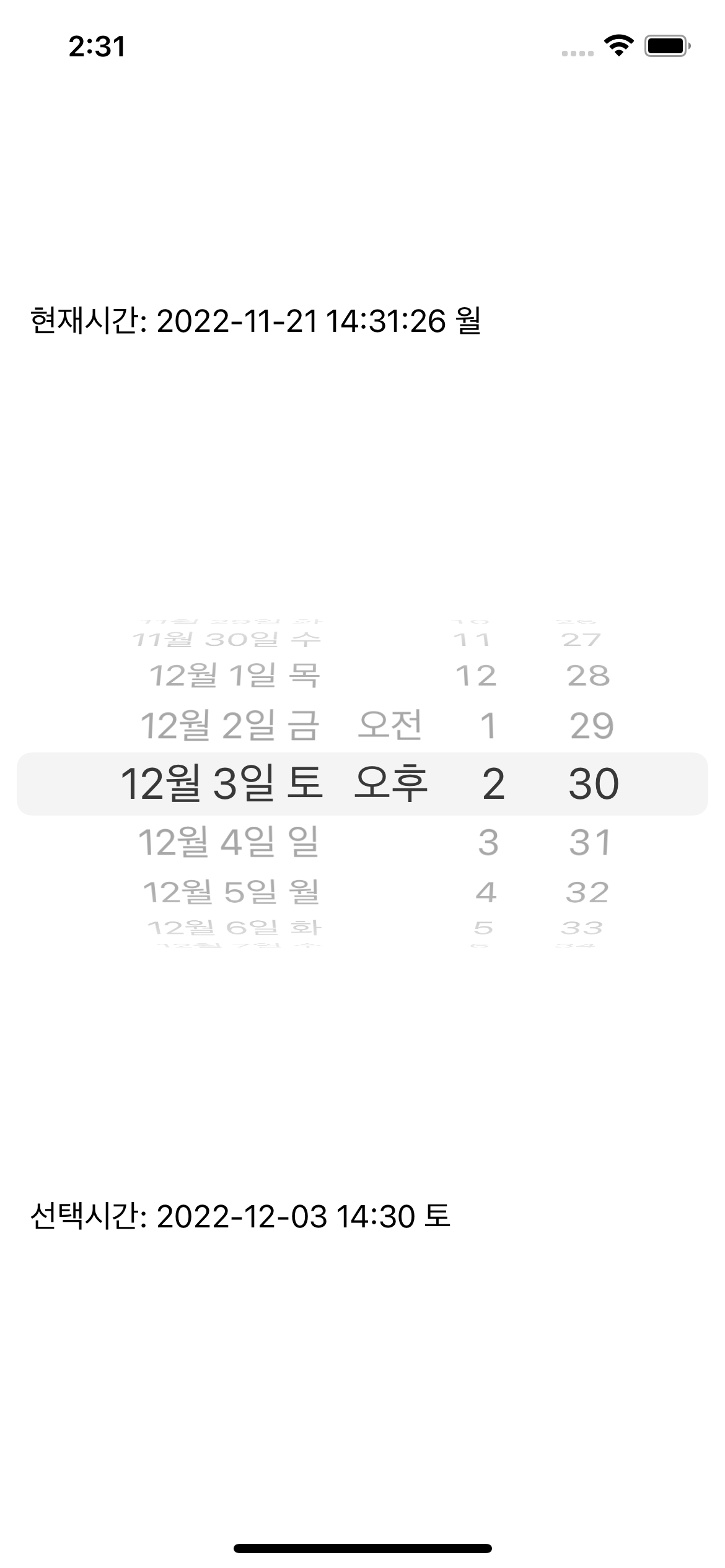
//
// ViewController.swift
// DatePicker
//
// Created by Ho-Jeong Song on 2021/11/24.
//
import UIKit
class ViewController: UIViewController {
let timeSelector: Selector = #selector(ViewController.updateTime)
let interval = 1.0
var count = 0
@IBOutlet var lblCurrentTime: UILabel!
@IBOutlet var lblPickerTime: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
Timer.scheduledTimer(timeInterval: interval, target: self, selector: timeSelector, userInfo: nil, repeats: true)
}
@IBAction func changeDatePicker(_ sender: UIDatePicker) {
let datePickerView = sender
let formatter = DateFormatter()
formatter.dateFormat = "yyyy-MM-dd HH:mm EEE"
lblPickerTime.text = "선택시간: " + formatter.string(from: datePickerView.date)
}
@objc func updateTime() {
// lblCurrentTime.text = String(count)
// count = count + 1
let date = NSDate()
let formatter = DateFormatter()
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss EEE"
lblCurrentTime.text = "현재시간: " + formatter.string(from: date as Date)
}
}
연결 관계
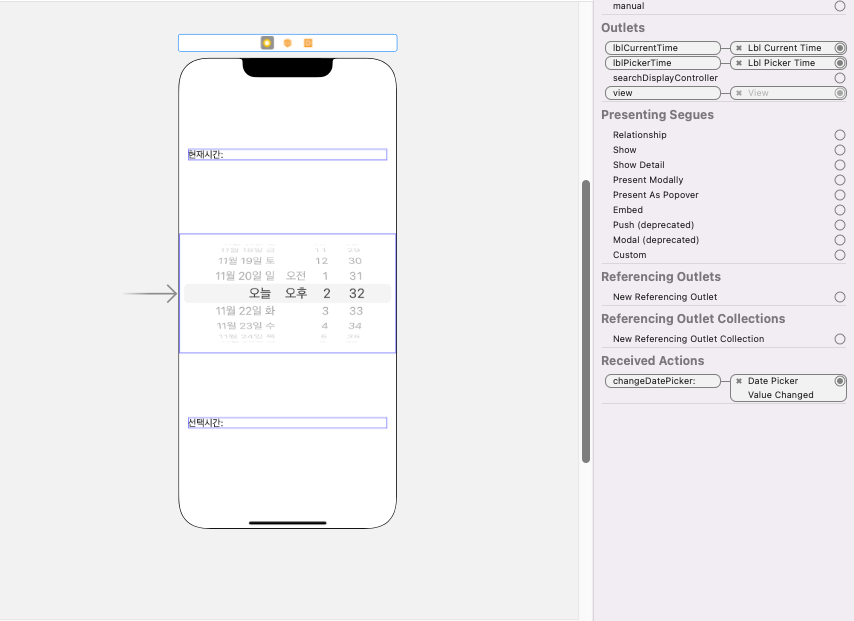
5강 본문 실습 픽커뷰
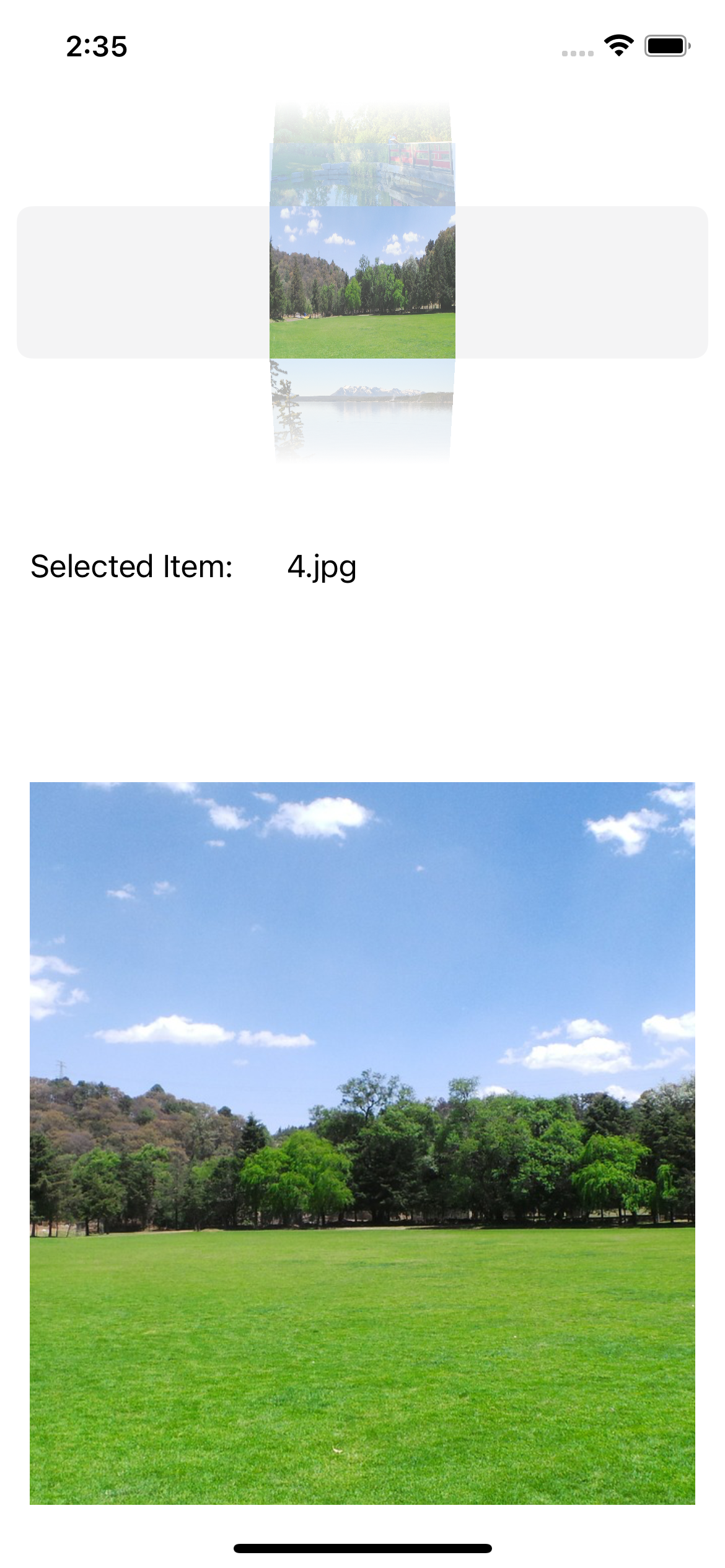
//
// ViewController.swift
// PickerView
//
// Created by Ho-Jeong Song on 2021/11/25.
//
import UIKit
class ViewController: UIViewController, UIPickerViewDelegate, UIPickerViewDataSource {
let MAX_ARRAY_NUM = 10
let PICKER_VIEW_COLUMN = 1
let PICKER_VIEW_HEIGHT:CGFloat = 80
var imageArray = [UIImage?]()
var imageFileName = [ "1.jpg", "2.jpg", "3.jpg", "4.jpg", "5.jpg",
"6.jpg", "7.jpg", "8.jpg", "9.jpg", "10.jpg" ]
@IBOutlet var pickerImage: UIPickerView!
@IBOutlet var lblImageFileName: UILabel!
@IBOutlet var imageView: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
for i in 0 ..< MAX_ARRAY_NUM {
let image = UIImage(named: imageFileName[i])
imageArray.append(image)
}
lblImageFileName.text = imageFileName[0]
imageView.image = imageArray[0]
}
// returns the number of 'columns' to display.
func numberOfComponents(in pickerView: UIPickerView) -> Int {
return PICKER_VIEW_COLUMN
}
// returns height of row for each component.
func pickerView(_ pickerView: UIPickerView, rowHeightForComponent component: Int) -> CGFloat {
return PICKER_VIEW_HEIGHT
}
// returns the # of rows in each component..
func pickerView(_ pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int {
return imageFileName.count
}
// func pickerView(_ pickerView: UIPickerView, titleForRow row: Int, forComponent component: Int) -> String? {
// return imageFileName[row]
// }
func pickerView(_ pickerView: UIPickerView, viewForRow row: Int, forComponent component: Int, reusing view: UIView?) -> UIView {
let imageView = UIImageView(image:imageArray[row])
imageView.frame = CGRect(x: 0, y: 0, width: 100, height: 150)
return imageView
}
func pickerView(_ pickerView: UIPickerView, didSelectRow row: Int, inComponent component: Int) {
lblImageFileName.text = imageFileName[row]
imageView.image = imageArray[row]
}
}
연결 관계
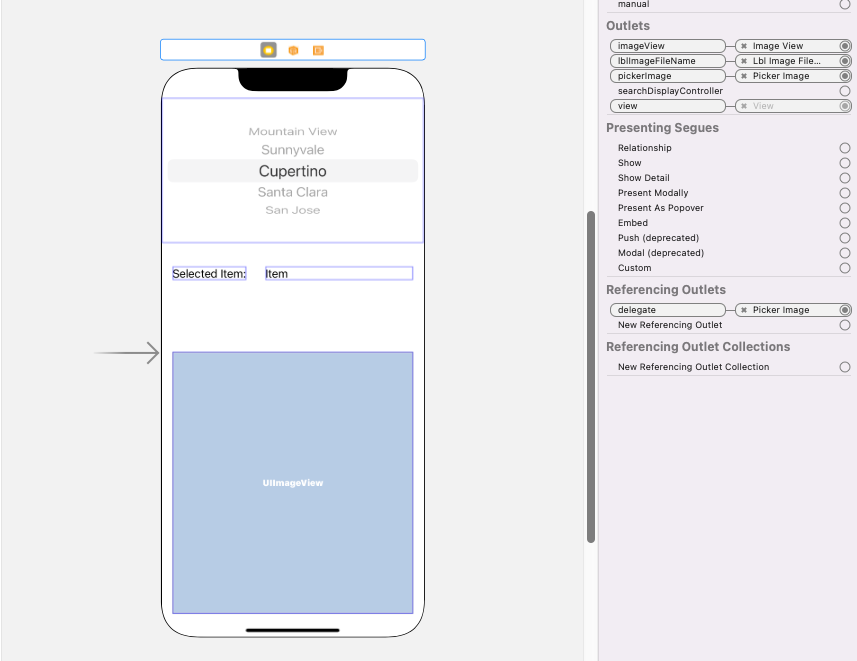
6강 경고창
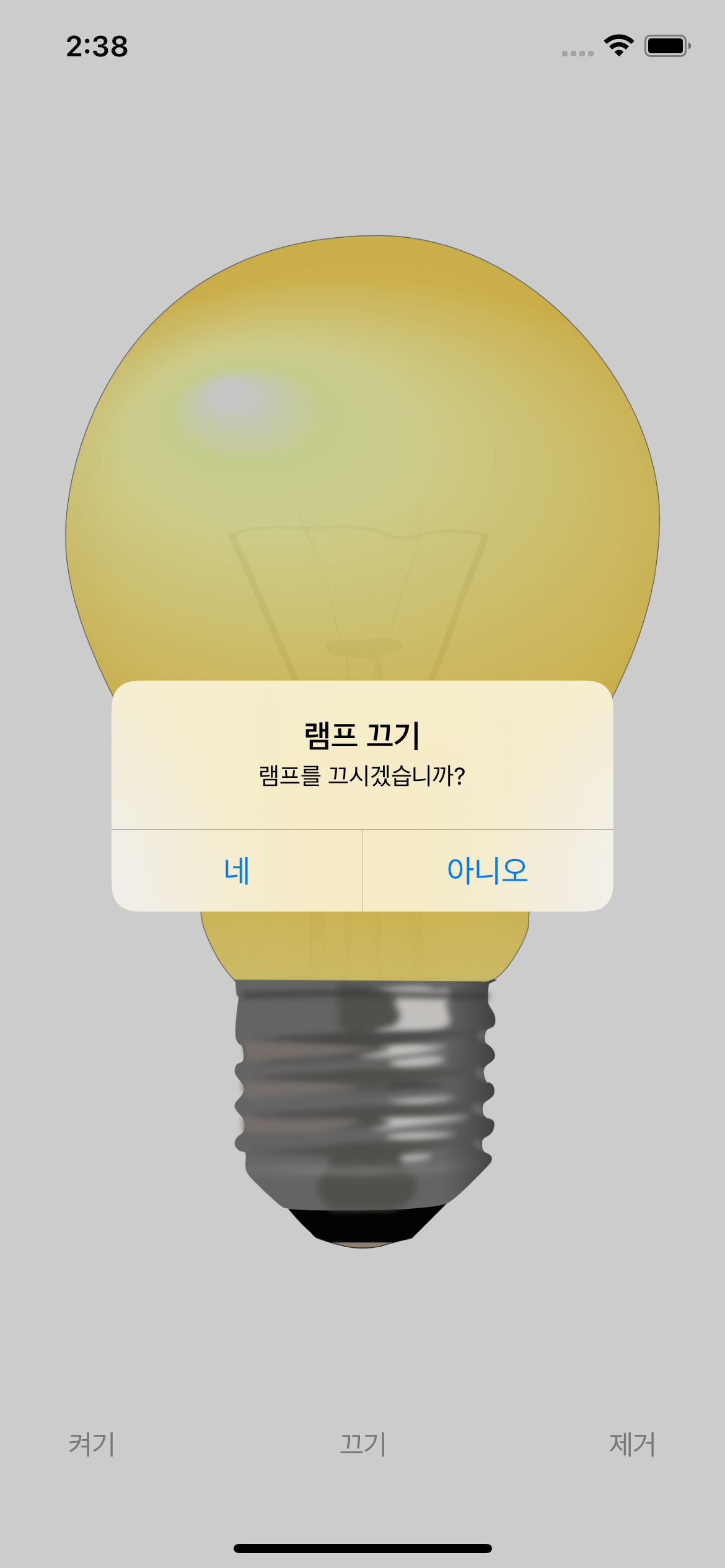
//
// ViewController.swift
// Alert
//
// Created by BeomGeun Lee on
//
import UIKit
class ViewController: UIViewController {
let imgOn = UIImage(named: "lamp-on.png")
let imgOff = UIImage(named: "lamp-off.png")
let imgRemove = UIImage(named: "lamp-remove.png")
var isLampOn = true
@IBOutlet var lampImg: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
lampImg.image = imgOn
}
@IBAction func btnLampOn(_ sender: UIButton) {
if(isLampOn==true) {
let lampOnAlert = UIAlertController(title: "경고", message: "현재 On 상태입니다", preferredStyle: UIAlertController.Style.alert)
let onAction = UIAlertAction(title: "네, 알겠습니다.", style: UIAlertAction.Style.default, handler: nil)
lampOnAlert.addAction(onAction)
present(lampOnAlert, animated: true, completion: nil)
} else {
lampImg.image = imgOn
isLampOn=true
}
}
@IBAction func btnLanpOff(_ sender: UIButton) {
if isLampOn {
let lampOffAlert = UIAlertController(title: "램프 끄기", message: "램프를 끄시겠습니까?", preferredStyle: UIAlertController.Style.alert)
let offAction = UIAlertAction(title: "네", style: UIAlertAction.Style.default, handler: {
ACTION in self.lampImg.image = self.imgOff
self.isLampOn=false
})
let cancelAction = UIAlertAction(title: "아니오", style: UIAlertAction.Style.default, handler: nil)
lampOffAlert.addAction(offAction)
lampOffAlert.addAction(cancelAction)
present(lampOffAlert, animated: true, completion: nil)
}
}
@IBAction func btnLampRemove(_ sender: UIButton) {
let lampRemoveAlert = UIAlertController(title: "램프 제거", message: "램프를 제거하시겠습니까?", preferredStyle: UIAlertController.Style.alert)
let offAction = UIAlertAction(title: "아니오, 끕니다(off).", style: UIAlertAction.Style.default, handler: {
ACTION in self.lampImg.image = self.imgOff
self.isLampOn=false
})
let onAction = UIAlertAction(title: "아니오, 켭니다(on).", style: UIAlertAction.Style.default) {
ACTION in self.lampImg.image = self.imgOn
self.isLampOn=true
}
let removeAction = UIAlertAction(title: "네, 제거합니다.", style: UIAlertAction.Style.destructive, handler: {
ACTION in self.lampImg.image = self.imgRemove
self.isLampOn=false
})
lampRemoveAlert.addAction(offAction)
lampRemoveAlert.addAction(onAction)
lampRemoveAlert.addAction(removeAction)
present(lampRemoveAlert, animated: true, completion: nil)
}
}
연결 관계
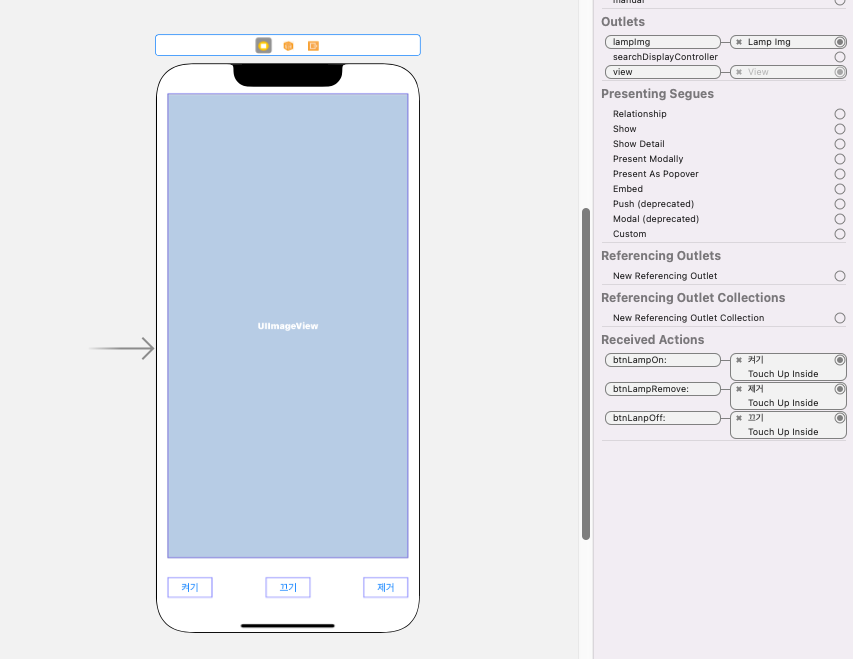
7강 본문실습 내장형 웹브라우저 삽입
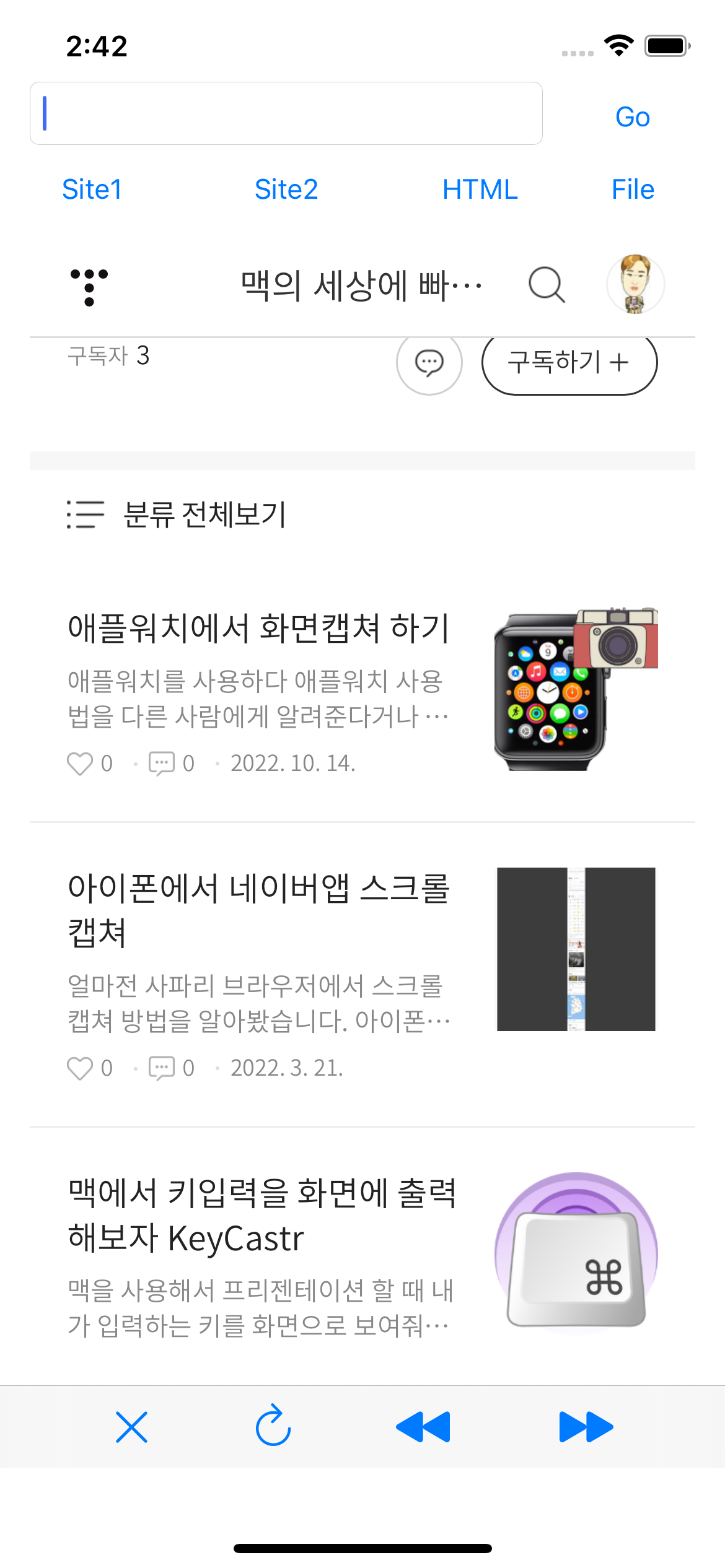
//
// ViewController.swift
// Web
//
// Created by BeomGeun Lee on
//
import UIKit
import WebKit
class ViewController: UIViewController, WKNavigationDelegate {
@IBOutlet var txtUrl: UITextField!
@IBOutlet var myWebView: WKWebView!
@IBOutlet var myActivityIndicator: UIActivityIndicatorView!
func loadWebPage(_ url: String) {
let myUrl = URL(string: url)
let myRequest = URLRequest(url: myUrl!)
myWebView.load(myRequest)
}
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
myWebView.navigationDelegate = self
loadWebPage("http://2sam.net")
}
func webView(_ webView: WKWebView, didCommit navigation: WKNavigation!) {
myActivityIndicator.startAnimating()
myActivityIndicator.isHidden = false
}
func webView(_ webView: WKWebView, didFinish navigation: WKNavigation!) {
myActivityIndicator.stopAnimating()
myActivityIndicator.isHidden = true
}
func webView(_ webView: WKWebView, didFail navigation: WKNavigation!, withError error: Error) {
myActivityIndicator.stopAnimating()
myActivityIndicator.isHidden = true
}
func checkUrl(_ url: String) -> String {
var strUrl = url
let flag = strUrl.hasPrefix("http://")
if !flag {
strUrl = "http://" + strUrl
}
return strUrl
}
@IBAction func btnGotoUrl(_ sender: UIButton) {
let myUrl = checkUrl(txtUrl.text!)
txtUrl.text = ""
loadWebPage(myUrl)
}
@IBAction func btnGoSite1(_ sender: UIButton) {
loadWebPage("http://fallinmac.tistory.com")
}
@IBAction func btnGoSite2(_ sender: UIButton) {
loadWebPage("http://blog.2sam.net")
}
@IBAction func btnLoadHtmlString(_ sender: UIButton) {
let htmlString = "<h1> HTML String </h1><p> String 변수를 이용한 웹페이지 </p> <p><a href=\"http://2sam.net\">2sam</a>으로 이동</p>"
myWebView.loadHTMLString(htmlString, baseURL: nil)
}
@IBAction func btnLoadHtmlFile(_ sender: UIButton) {
let filePath = Bundle.main.path(forResource: "htmlView", ofType: "html")
let myUrl = URL(fileURLWithPath: filePath!)
let myRequest = URLRequest(url: myUrl)
myWebView.load(myRequest)
}
@IBAction func btnStop(_ sender: UIBarButtonItem) {
myWebView.stopLoading()
}
@IBAction func btnReload(_ sender: UIBarButtonItem) {
myWebView.reload()
}
@IBAction func btnGoBack(_ sender: UIBarButtonItem) {
myWebView.goBack()
}
@IBAction func btnGoForward(_ sender: UIBarButtonItem) {
myWebView.goForward()
}
}
연결 관계
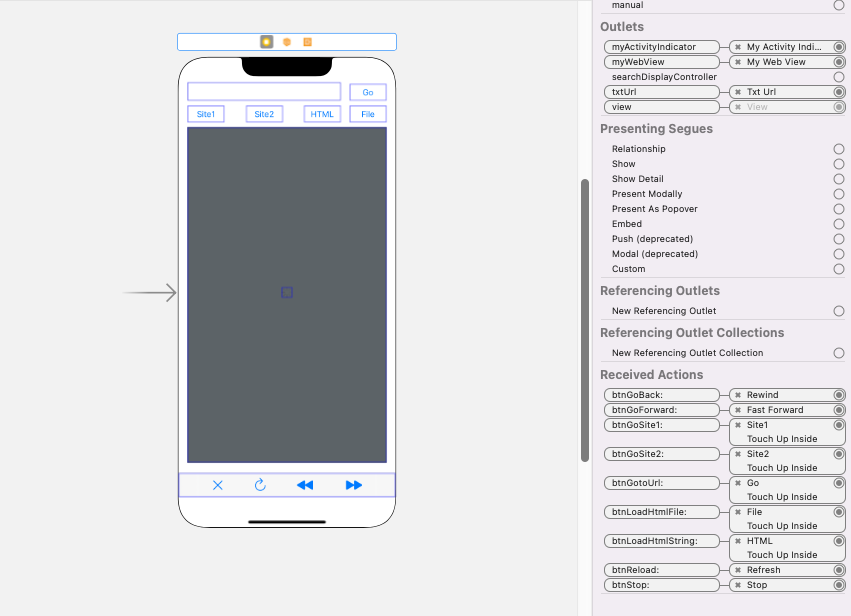
8강 지도 넘어가기
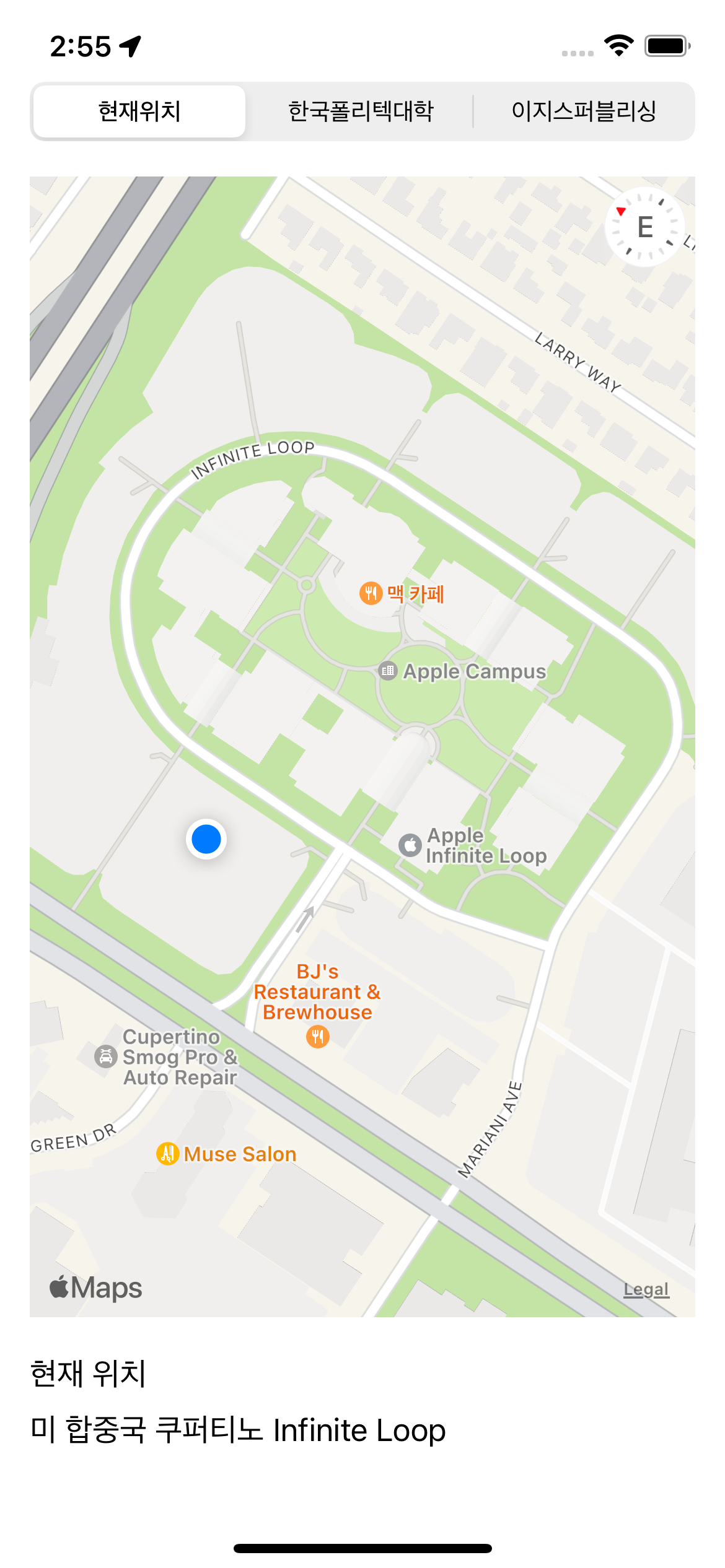
//
// ViewController.swift
// Map
//
// Created by BeomGeun Lee on 2021
//
import UIKit
import MapKit
class ViewController: UIViewController, CLLocationManagerDelegate {
@IBOutlet var myMap: MKMapView!
@IBOutlet var lblLocationInfo1: UILabel!
@IBOutlet var lblLocationInfo2: UILabel!
let locationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
lblLocationInfo1.text = ""
lblLocationInfo2.text = ""
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestWhenInUseAuthorization()
locationManager.startUpdatingLocation()
myMap.showsUserLocation = true
}
func goLocation(latitudeValue: CLLocationDegrees, longitudeValue : CLLocationDegrees, delta span :Double)-> CLLocationCoordinate2D {
let pLocation = CLLocationCoordinate2DMake(latitudeValue, longitudeValue)
let spanValue = MKCoordinateSpan(latitudeDelta: span, longitudeDelta: span)
let pRegion = MKCoordinateRegion(center: pLocation, span: spanValue)
myMap.setRegion(pRegion, animated: true)
return pLocation
}
func setAnnotation(latitudeValue: CLLocationDegrees, longitudeValue : CLLocationDegrees, delta span :Double, title strTitle: String, subtitle strSubtitle:String) {
let annotation = MKPointAnnotation()
annotation.coordinate = goLocation(latitudeValue: latitudeValue, longitudeValue: longitudeValue, delta: span)
annotation.title = strTitle
annotation.subtitle = strSubtitle
myMap.addAnnotation(annotation)
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
let pLocation = locations.last
_ = goLocation(latitudeValue: (pLocation?.coordinate.latitude)!, longitudeValue: (pLocation?.coordinate.longitude)!, delta: 0.01)
CLGeocoder().reverseGeocodeLocation(pLocation!, completionHandler: {
(placemarks, error) -> Void in
let pm = placemarks!.first
let country = pm!.country
var address:String = country!
if pm!.locality != nil {
address += " "
address += pm!.locality!
}
if pm!.thoroughfare != nil {
address += " "
address += pm!.thoroughfare!
}
self.lblLocationInfo1.text = "현재 위치"
self.lblLocationInfo2.text = address
})
locationManager.stopUpdatingLocation()
}
@IBAction func sgChangeLocation(_ sender: UISegmentedControl) {
if sender.selectedSegmentIndex == 0 {
self.lblLocationInfo1.text = ""
self.lblLocationInfo2.text = ""
locationManager.startUpdatingLocation()
} else if sender.selectedSegmentIndex == 1 {
setAnnotation(latitudeValue: 37.751853, longitudeValue: 128.87605740000004, delta: 1, title: "한국폴리텍대학 강릉캠퍼스", subtitle: "강원도 강릉시 남산초교길 121")
self.lblLocationInfo1.text = "보고 계신 위치"
self.lblLocationInfo2.text = "한국폴리텍대학 강릉캠퍼스"
} else if sender.selectedSegmentIndex == 2 {
setAnnotation(latitudeValue: 37.556876, longitudeValue: 126.914066, delta: 0.1, title: "이지스퍼블리싱", subtitle: "서울시 마포구 잔다리로 109 이지스 빌딩")
self.lblLocationInfo1.text = "보고 계신 위치"
self.lblLocationInfo2.text = "이지스퍼블리싱 출판사 "
}
}
}
연결 관계
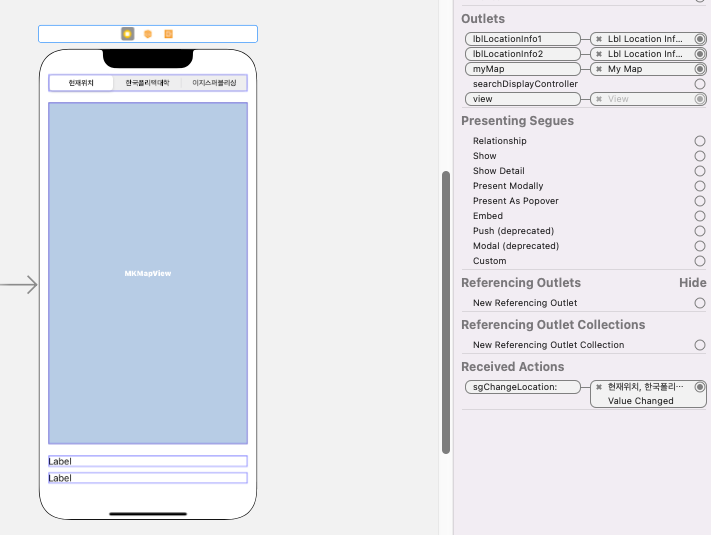

지도 이용시 이렇게 테스트 가능 , 시뮬레이터에서 art 누르면 확대 축소 가능
9강
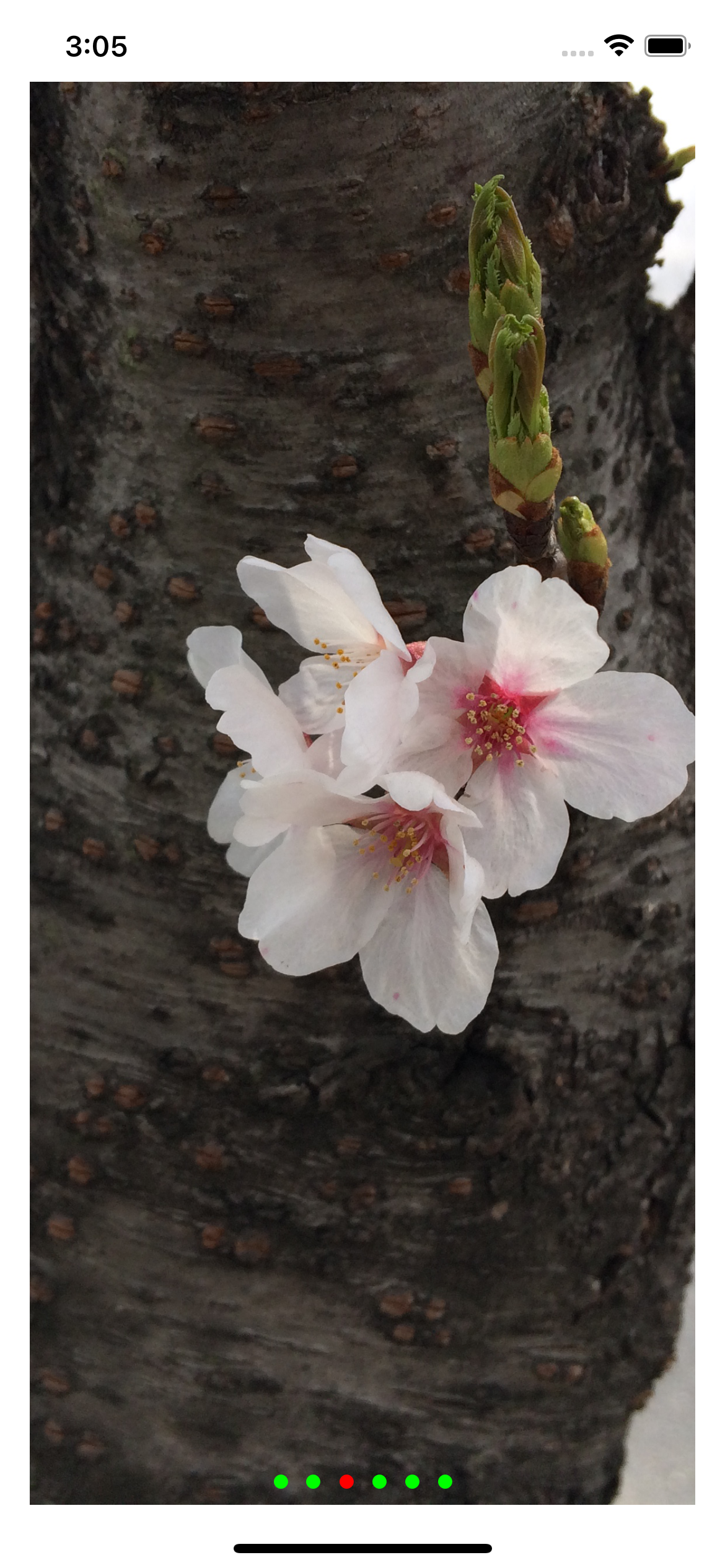
//
// ViewController.swift
// PageControl
//
// Created by Ho-Jeong Song on 2021/11/25.
//
import UIKit
var images = [ "01.png", "02.png", "03.png", "04.png", "05.png", "06.png" ]
class ViewController: UIViewController {
@IBOutlet var imgView: UIImageView!
@IBOutlet var pageControl: UIPageControl!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
pageControl.numberOfPages = images.count
pageControl.currentPage = 0
pageControl.pageIndicatorTintColor = UIColor.green
pageControl.currentPageIndicatorTintColor = UIColor.red
imgView.image = UIImage(named: images[0])
}
@IBAction func pageChange(_ sender: UIPageControl) {
imgView.image = UIImage(named: images[pageControl.currentPage])
}
}
연결관계
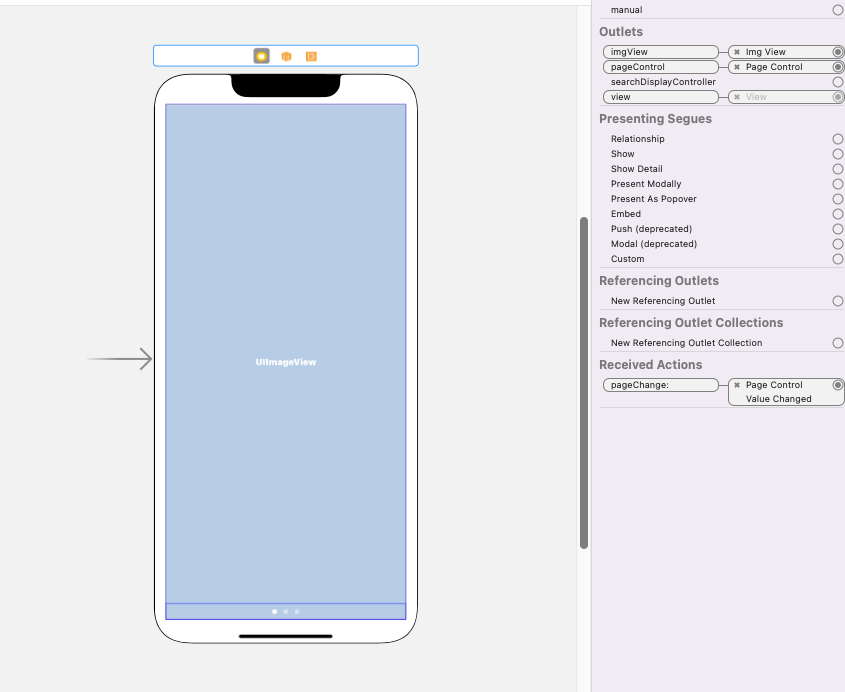
10강

//
// ViewController.swift
// Tab
//
// Created by BeomGeun Lee on 2021.
//
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnMoveImageView(_ sender: UIButton) {
tabBarController?.selectedIndex = 1
}
@IBAction func btnMoveDatePickerView(_ sender: UIButton) {
tabBarController?.selectedIndex = 2
}
}
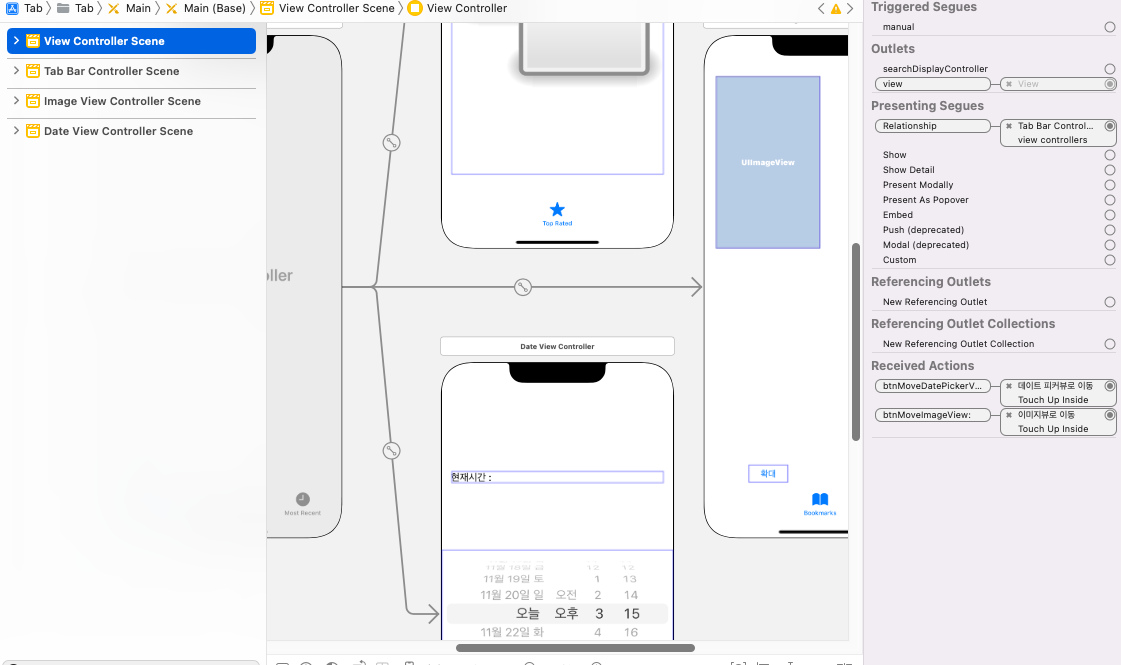
11강

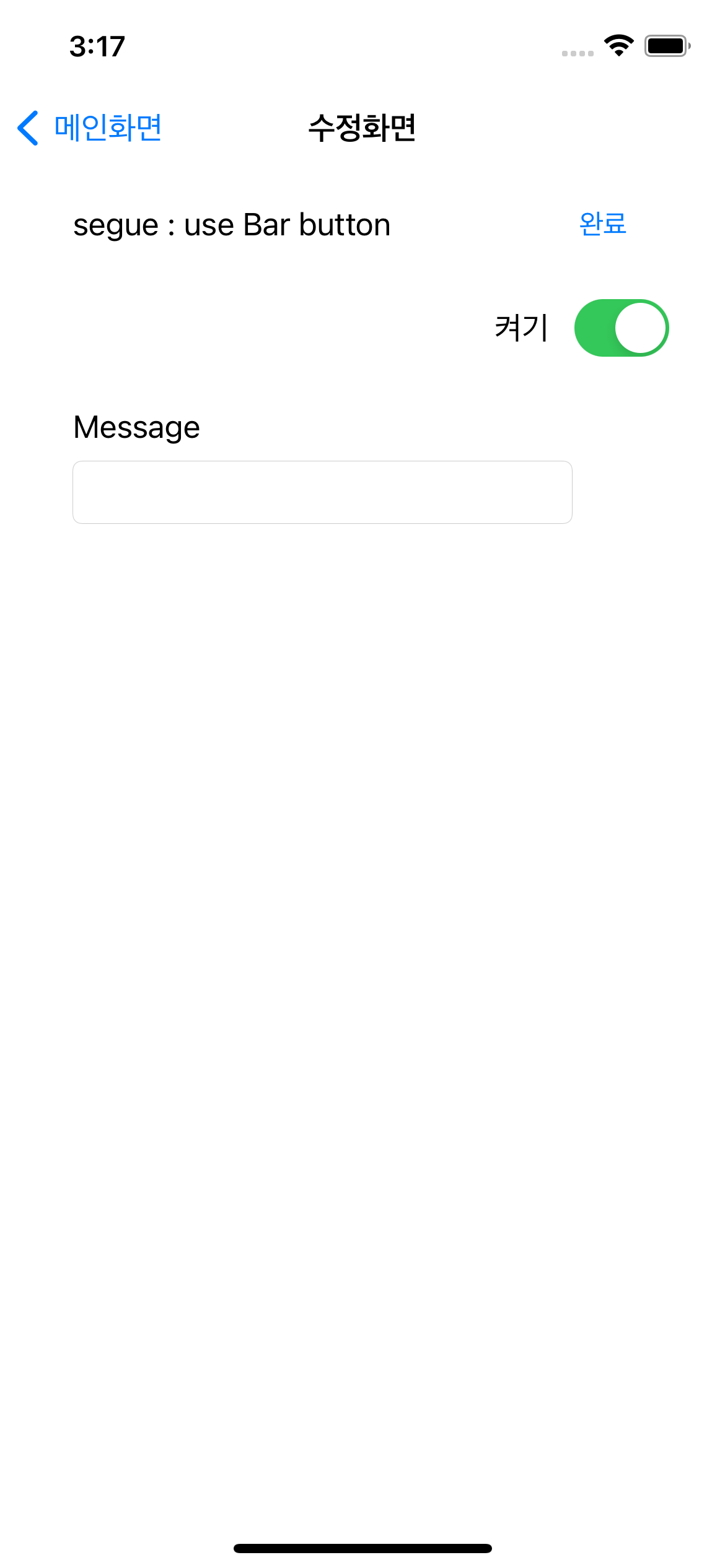
//
// ViewController.swift
// Navigation
//
// Created by BeomGeun Lee on 2021.
//
import UIKit
class ViewController: UIViewController, EditDelegate {
let imgOn = UIImage(named: "lamp_on.png")
let imgOff = UIImage(named: "lamp_off.png")
var isOn = true
@IBOutlet var txMessage: UITextField!
@IBOutlet var imgView: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
imgView.image = imgOn
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
let editViewController = segue.destination as! EditViewController
if segue.identifier == "editButton" {
editViewController.textWayValue = "segue : use button"
} else if segue.identifier == "editBarButton" {
editViewController.textWayValue = "segue : use Bar button"
}
editViewController.textMessage = txMessage.text!
editViewController.isOn = isOn
editViewController.delegate = self
}
func didMessageEditDone(_ controller: EditViewController, message: String) {
txMessage.text = message
}
func didImageOnOffDone(_ controller: EditViewController, isOn: Bool) {
if isOn {
imgView.image = imgOn
self.isOn = true
} else {
imgView.image = imgOff
self.isOn = false
}
}
}
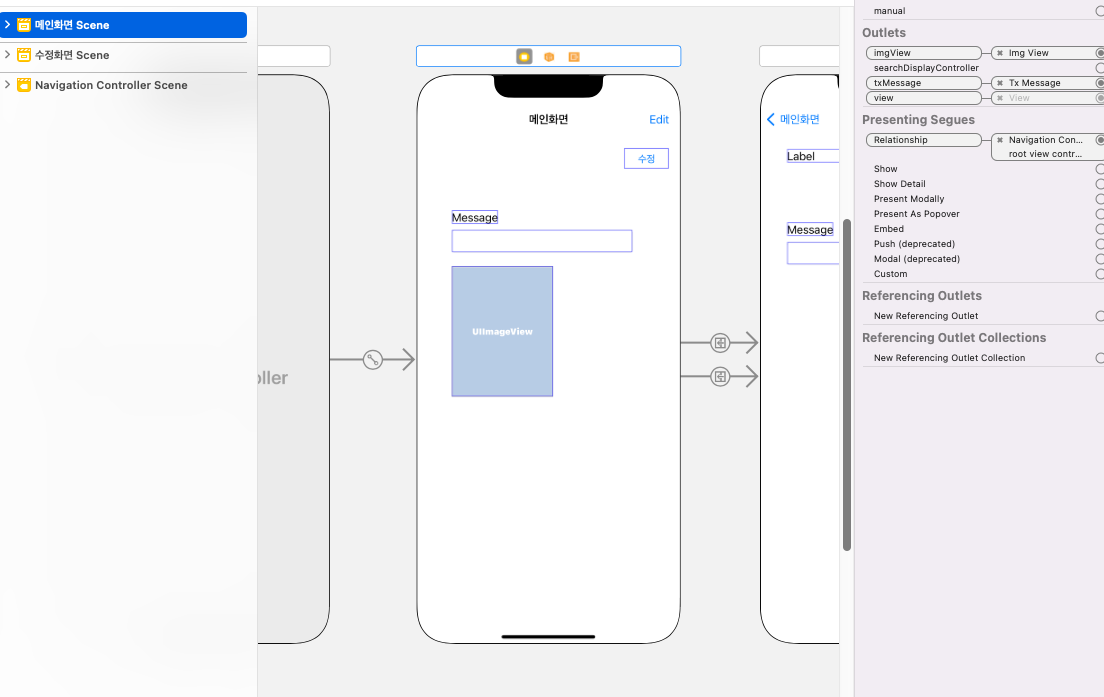
12강
(투두 리스트)테이블
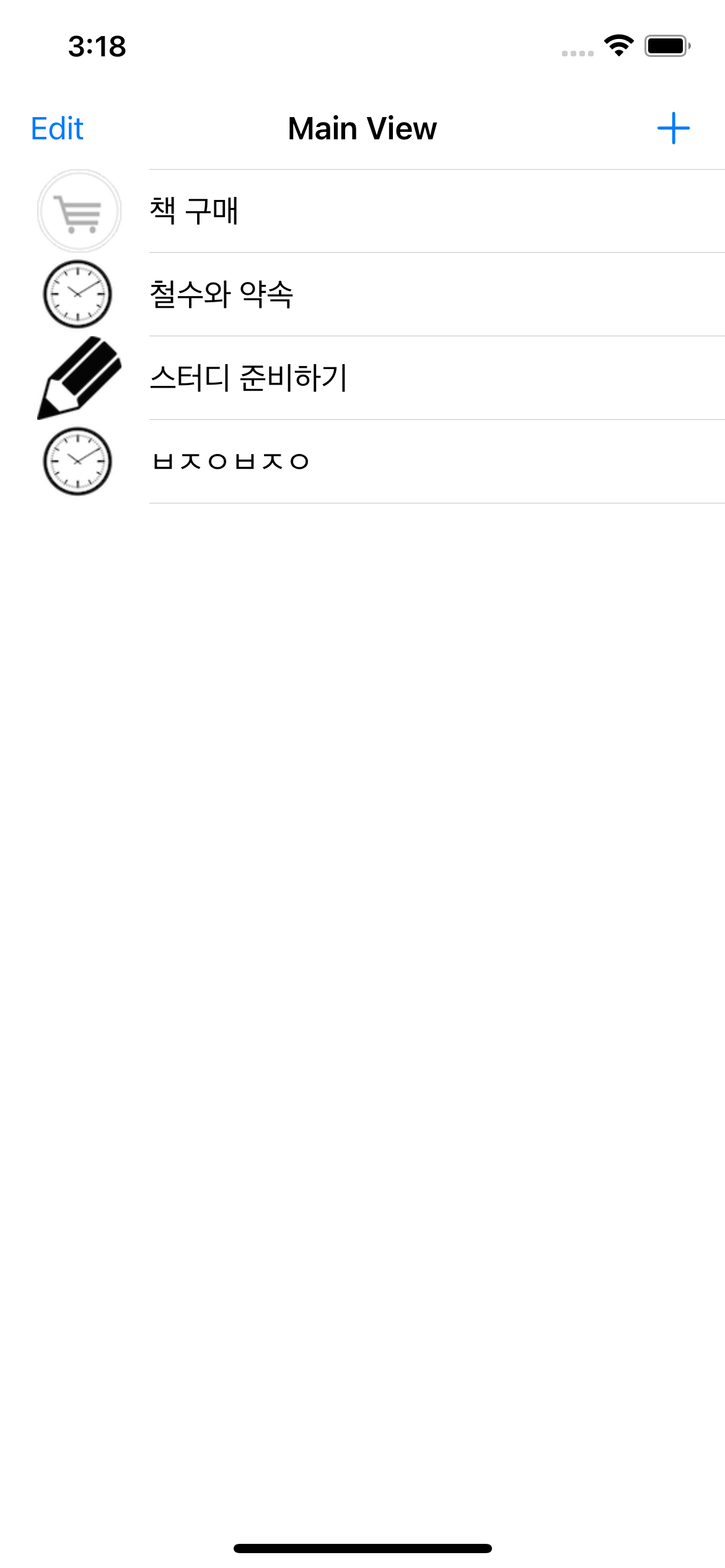
13강(오디오 출력)
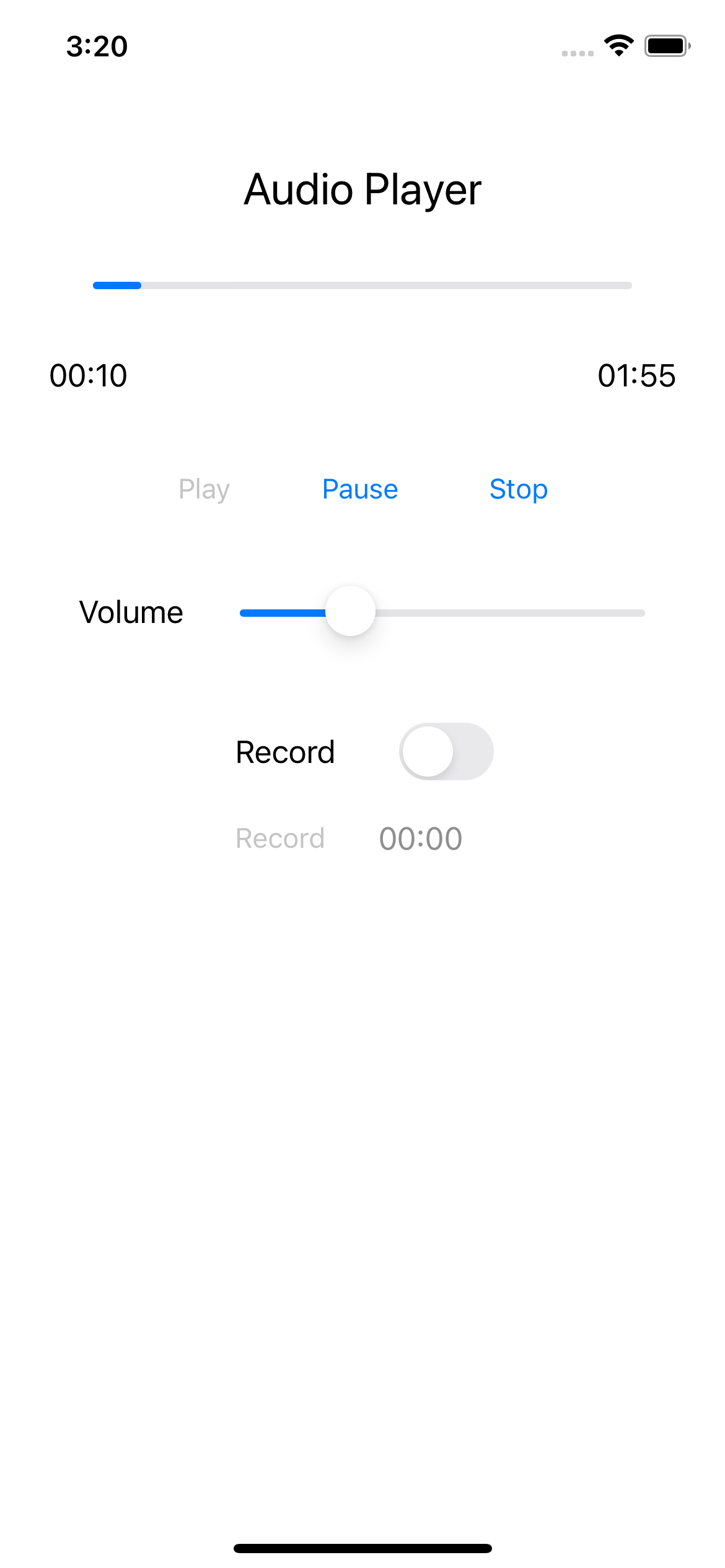
//
// ViewController.swift
// Audio
//
// Created by BeomGeun Lee on 2021.
//
import UIKit
import AVFoundation
class ViewController: UIViewController, AVAudioPlayerDelegate, AVAudioRecorderDelegate {
var audioPlayer : AVAudioPlayer!
var audioFile : URL!
let MAX_VOLUME : Float = 10.0
var progressTimer : Timer!
let timePlayerSelector:Selector = #selector(ViewController.updatePlayTime)
let timeRecordSelector:Selector = #selector(ViewController.updateRecordTime)
@IBOutlet var pvProgressPlay: UIProgressView!
@IBOutlet var lblCurrentTime: UILabel!
@IBOutlet var lblEndTime: UILabel!
@IBOutlet var btnPlay: UIButton!
@IBOutlet var btnPause: UIButton!
@IBOutlet var btnStop: UIButton!
@IBOutlet var slVolume: UISlider!
@IBOutlet var btnRecord: UIButton!
@IBOutlet var lblRecordTime: UILabel!
var audioRecorder : AVAudioRecorder!
var isRecordMode = false
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
selectAudioFile()
if !isRecordMode {
initPlay()
btnRecord.isEnabled = false
lblRecordTime.isEnabled = false
} else {
initRecord()
}
}
func selectAudioFile() {
if !isRecordMode {
audioFile = Bundle.main.url(forResource: "Sicilian_Breeze", withExtension: "mp3")
} else {
let documentDirectory = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)[0]
audioFile = documentDirectory.appendingPathComponent("recordFile.m4a")
}
}
func initRecord() {
let recordSettings = [
AVFormatIDKey : NSNumber(value: kAudioFormatAppleLossless as UInt32),
AVEncoderAudioQualityKey : AVAudioQuality.max.rawValue,
AVEncoderBitRateKey : 320000,
AVNumberOfChannelsKey : 2,
AVSampleRateKey : 44100.0] as [String : Any]
do {
audioRecorder = try AVAudioRecorder(url: audioFile, settings: recordSettings)
} catch let error as NSError {
print("Error-initRecord : \(error)")
}
audioRecorder.delegate = self
slVolume.value = 1.0
audioPlayer.volume = slVolume.value
lblEndTime.text = convertNSTimeInterval2String(0)
lblCurrentTime.text = convertNSTimeInterval2String(0)
setPlayButtons(false, pause: false, stop: false)
let session = AVAudioSession.sharedInstance()
do {
try AVAudioSession.sharedInstance().setCategory(.playAndRecord, mode: .default)
try AVAudioSession.sharedInstance().setActive(true)
} catch let error as NSError {
print(" Error-setCategory : \(error)")
}
do {
try session.setActive(true)
} catch let error as NSError {
print(" Error-setActive : \(error)")
}
}
func initPlay() {
do {
audioPlayer = try AVAudioPlayer(contentsOf: audioFile)
} catch let error as NSError {
print("Error-initPlay : \(error)")
}
slVolume.maximumValue = MAX_VOLUME
slVolume.value = 1.0
pvProgressPlay.progress = 0
audioPlayer.delegate = self
audioPlayer.prepareToPlay()
audioPlayer.volume = slVolume.value
lblEndTime.text = convertNSTimeInterval2String(audioPlayer.duration)
lblCurrentTime.text = convertNSTimeInterval2String(0)
setPlayButtons(true, pause: false, stop: false)
}
func setPlayButtons(_ play:Bool, pause:Bool, stop:Bool) {
btnPlay.isEnabled = play
btnPause.isEnabled = pause
btnStop.isEnabled = stop
}
func convertNSTimeInterval2String(_ time:TimeInterval) -> String {
let min = Int(time/60)
let sec = Int(time.truncatingRemainder(dividingBy: 60))
let strTime = String(format: "%02d:%02d", min, sec)
return strTime
}
@IBAction func btnPlayAudio(_ sender: UIButton) {
audioPlayer.play()
setPlayButtons(false, pause: true, stop: true)
progressTimer = Timer.scheduledTimer(timeInterval: 0.1, target: self, selector: timePlayerSelector, userInfo: nil, repeats: true)
}
@objc func updatePlayTime() {
lblCurrentTime.text = convertNSTimeInterval2String(audioPlayer.currentTime)
pvProgressPlay.progress = Float(audioPlayer.currentTime/audioPlayer.duration)
}
@IBAction func btnPauseAudio(_ sender: UIButton) {
audioPlayer.pause()
setPlayButtons(true, pause: false, stop: true)
}
@IBAction func btnStopAudio(_ sender: UIButton) {
audioPlayer.stop()
audioPlayer.currentTime = 0
lblCurrentTime.text = convertNSTimeInterval2String(0)
setPlayButtons(true, pause: false, stop: false)
progressTimer.invalidate()
}
@IBAction func slChangeVolume(_ sender: UISlider) {
audioPlayer.volume = slVolume.value
}
func audioPlayerDidFinishPlaying(_ player: AVAudioPlayer, successfully flag: Bool) {
progressTimer.invalidate()
setPlayButtons(true, pause: false, stop: false)
}
@IBAction func swRecordMode(_ sender: UISwitch) {
if sender.isOn {
audioPlayer.stop()
audioPlayer.currentTime=0
lblRecordTime!.text = convertNSTimeInterval2String(0)
isRecordMode = true
btnRecord.isEnabled = true
lblRecordTime.isEnabled = true
} else {
isRecordMode = false
btnRecord.isEnabled = false
lblRecordTime.isEnabled = false
lblRecordTime.text = convertNSTimeInterval2String(0)
}
selectAudioFile()
if !isRecordMode {
initPlay()
} else {
initRecord()
}
}
@IBAction func btnRecord(_ sender: UIButton) {
if (sender as AnyObject).titleLabel?.text == "Record" {
audioRecorder.record()
(sender as AnyObject).setTitle("Stop", for: UIControl.State())
progressTimer = Timer.scheduledTimer(timeInterval: 0.1, target: self, selector: timeRecordSelector, userInfo: nil, repeats: true)
} else {
audioRecorder.stop()
progressTimer.invalidate()
(sender as AnyObject).setTitle("Record", for: UIControl.State())
btnPlay.isEnabled = true
initPlay()
}
}
@objc func updateRecordTime() {
lblRecordTime.text = convertNSTimeInterval2String(audioRecorder.currentTime)
}
}
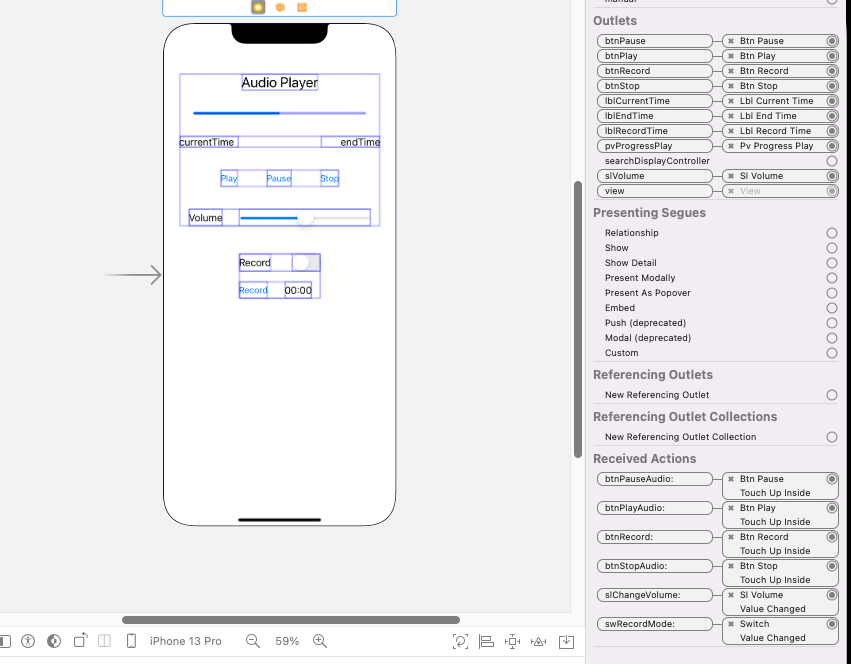
14강
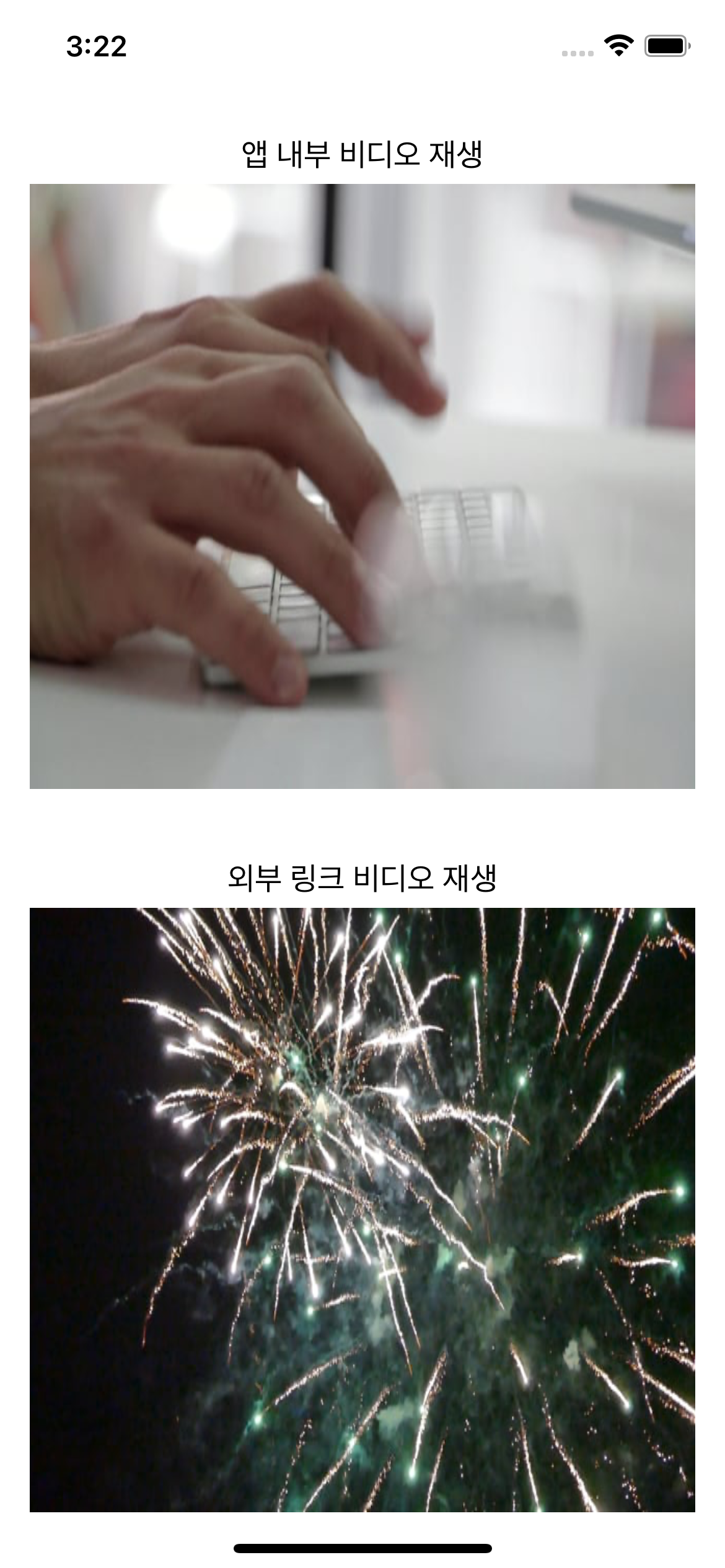
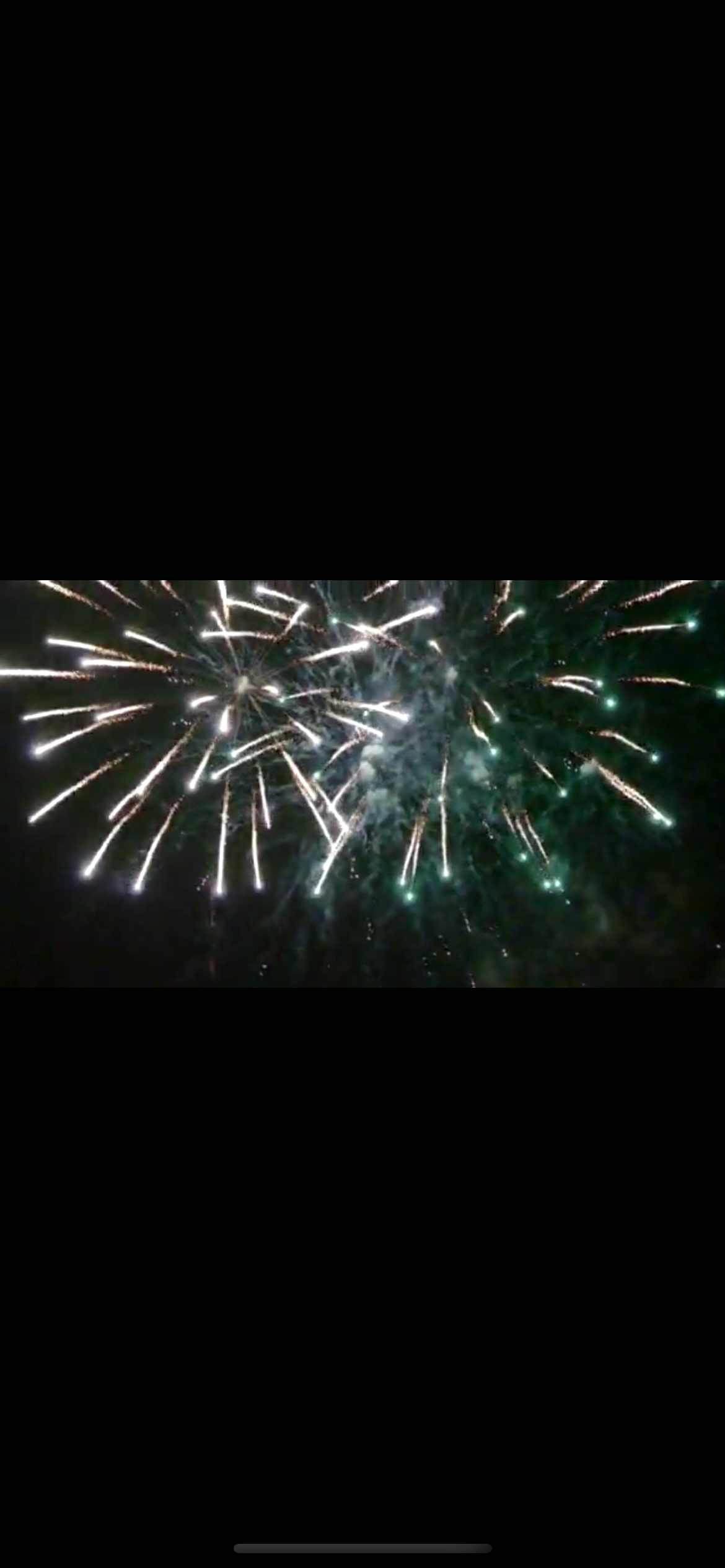
//
// ViewController.swift
// MoviePlayer
//
// Created by Ho-Jeong Song on 2021/11/26.
//
import UIKit
import AVKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnPlayInternalMovie(_ sender: UIButton) {
// 내부 파일 mp4
let filePath:String? = Bundle.main.path(forResource: "FastTyping", ofType: "mp4")
let url = NSURL(fileURLWithPath: filePath!)
playVideo(url: url)
}
@IBAction func btnPlayerExternalMovie(_ sender: UIButton) {
// 외부 파일 mp4
let url = NSURL(string: "https://dl.dropboxusercontent.com/s/e38auz050w2mvud/Fireworks.mp4")!
playVideo(url: url)
}
private func playVideo(url: NSURL) {
let playerController = AVPlayerViewController()
let player = AVPlayer(url: url as URL)
playerController.player = player
self.present(playerController, animated: true) {
player.play()
}
}
}
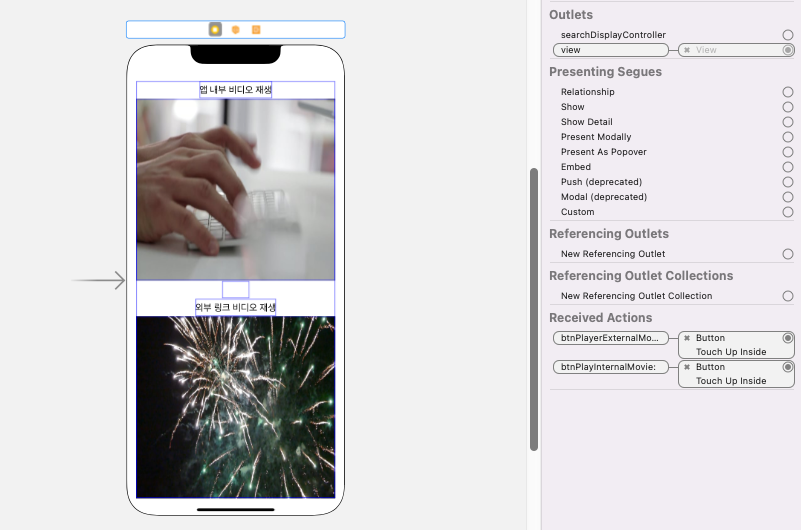
15강
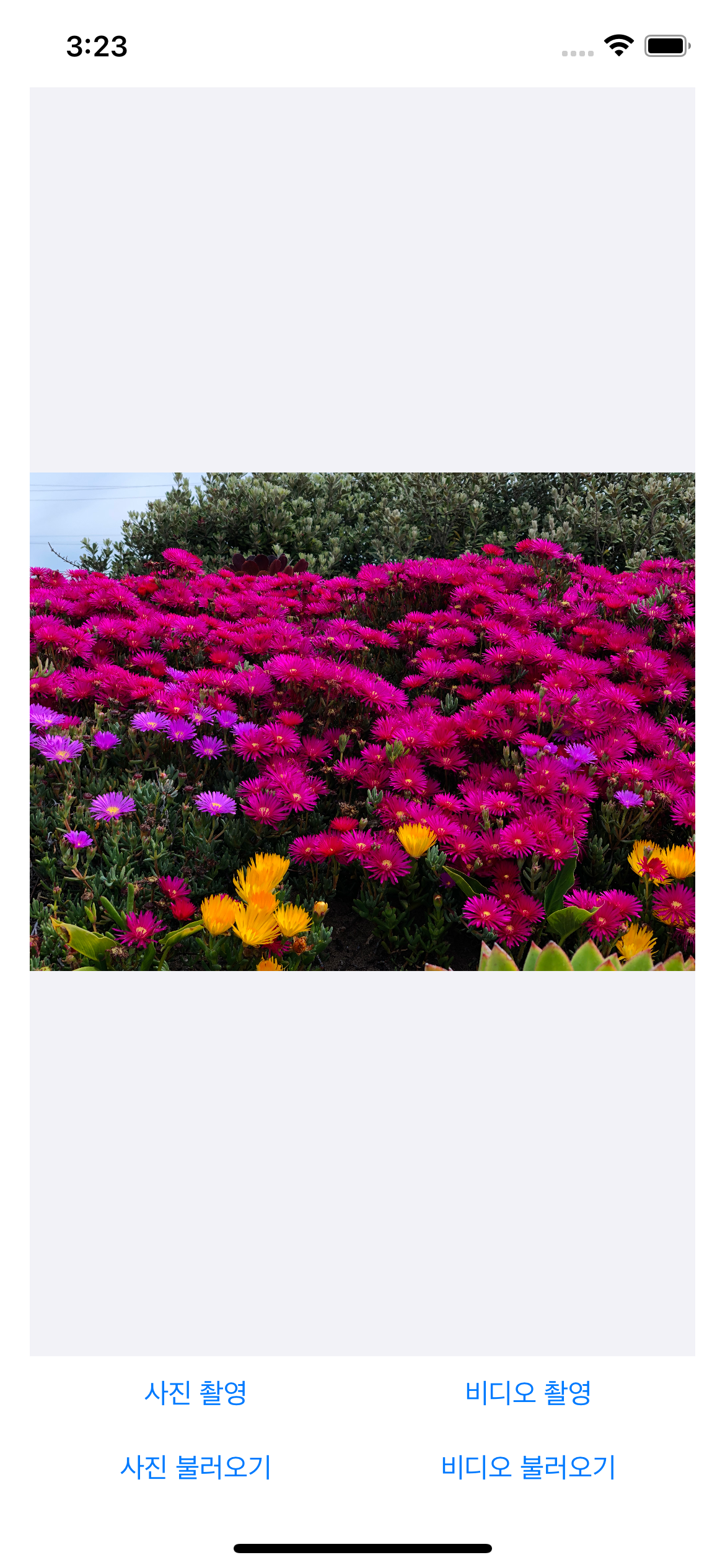
//
// ViewController.swift
// CameraPhotoLibrary
//
// Created by Ho-Jeong Song on 2021/11/27.
//
import UIKit
import MobileCoreServices
class ViewController: UIViewController, UINavigationControllerDelegate, UIImagePickerControllerDelegate {
@IBOutlet var imgView: UIImageView!
let imagePicker: UIImagePickerController! = UIImagePickerController()
var captureImage: UIImage!
var videoURL: URL!
var flagImageSave = false
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnCaptureImageFromCamera(_ sender: UIButton) {
if (UIImagePickerController.isSourceTypeAvailable(.camera)) {
flagImageSave = true
imagePicker.delegate = self
imagePicker.sourceType = .camera
imagePicker.mediaTypes = ["public.image"]
imagePicker.allowsEditing = false
present(imagePicker, animated: true, completion: nil)
}
else {
myAlert("Camera inaccessable", message: "Application cannot access the camera.")
}
}
@IBAction func btnLoadImageFromLibrary(_ sender: UIButton) {
if (UIImagePickerController.isSourceTypeAvailable(.photoLibrary)) {
flagImageSave = false
imagePicker.delegate = self
imagePicker.sourceType = .photoLibrary
imagePicker.mediaTypes = ["public.image"]
imagePicker.allowsEditing = true
present(imagePicker, animated: true, completion: nil)
}
else {
myAlert("Photo album inaccessable", message: "Application cannot access the photo album.")
}
}
@IBAction func btnRecordVideoFromCamera(_ sender: UIButton) {
if (UIImagePickerController.isSourceTypeAvailable(.camera)) {
flagImageSave = true
imagePicker.delegate = self
imagePicker.sourceType = .camera
imagePicker.mediaTypes = ["public.movie"]
imagePicker.allowsEditing = false
present(imagePicker, animated: true, completion: nil)
}
else {
myAlert("Camera inaccessable", message: "Application cannot access the camera.")
}
}
@IBAction func btnLoadVideoFromLibrary(_ sender: UIButton) {
if (UIImagePickerController.isSourceTypeAvailable(.photoLibrary)) {
flagImageSave = false
imagePicker.delegate = self
imagePicker.sourceType = .photoLibrary
imagePicker.mediaTypes = ["public.movie"]
imagePicker.allowsEditing = false
present(imagePicker, animated: true, completion: nil)
}
else {
myAlert("Photo album inaccessable", message: "Application cannot access the photo album.")
}
}
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [UIImagePickerController.InfoKey : Any]) {
let mediaType = info[UIImagePickerController.InfoKey.mediaType] as! NSString
if mediaType.isEqual(to: "public.image" as String) {
captureImage = info[UIImagePickerController.InfoKey.originalImage] as? UIImage
if flagImageSave {
UIImageWriteToSavedPhotosAlbum(captureImage, self, nil, nil)
}
imgView.image = captureImage
}
else if mediaType.isEqual(to: "public.movie" as String) {
if flagImageSave {
videoURL = (info[UIImagePickerController.InfoKey.mediaURL] as! URL)
UISaveVideoAtPathToSavedPhotosAlbum(videoURL.relativePath, self, nil, nil)
}
}
self.dismiss(animated: true, completion: nil)
}
func imagePickerControllerDidCancel(_ picker: UIImagePickerController) {
self.dismiss(animated: true, completion: nil)
}
func myAlert(_ title: String, message: String) {
let alert = UIAlertController(title: title, message: message, preferredStyle: UIAlertController.Style.alert)
let action = UIAlertAction(title: "Ok", style: UIAlertAction.Style.default, handler: nil)
alert.addAction(action)
self.present(alert, animated: true, completion: nil)
}
}

16강
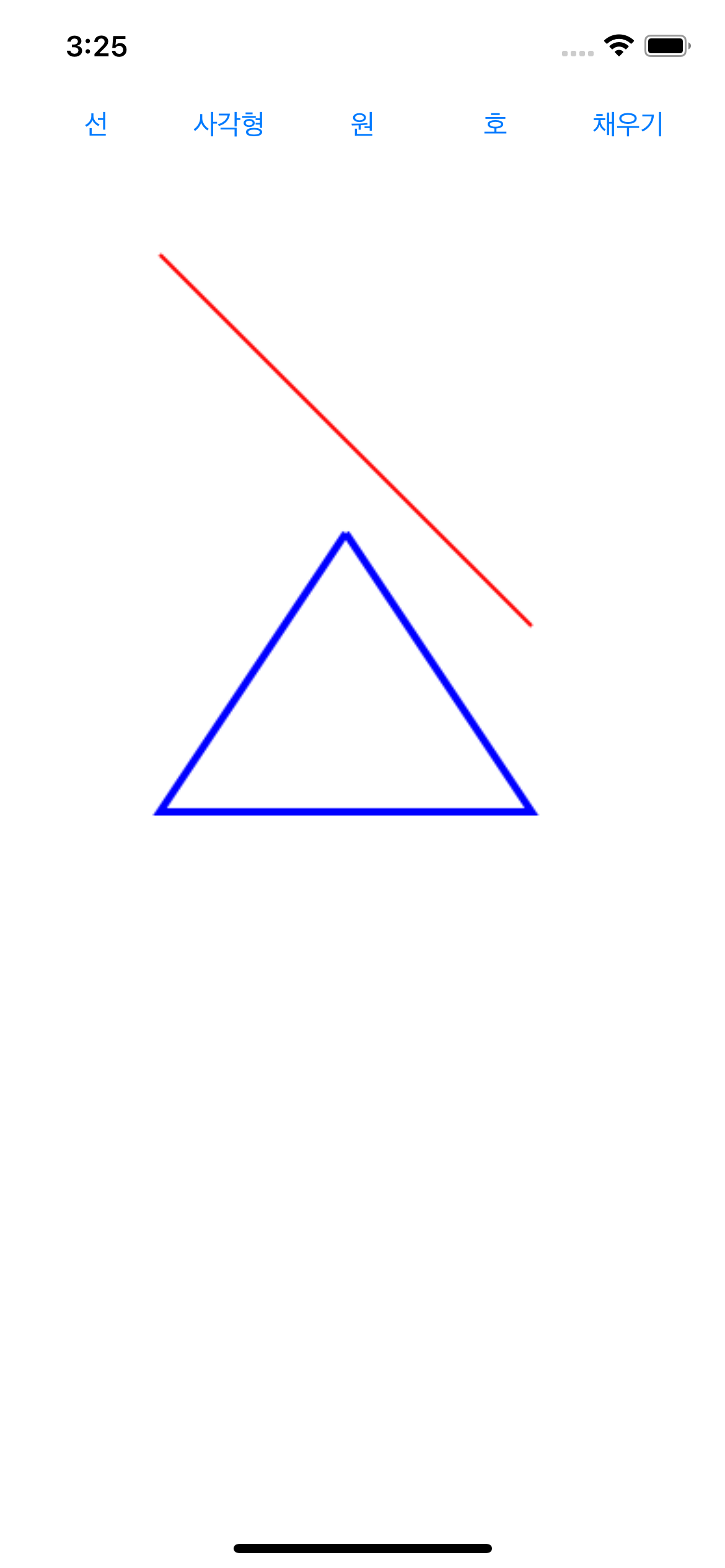
//
// ViewController.swift
// DrawGraphics
//
// Created by Ho-Jeong Song on 2021/12/01.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var imgView: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnDrawLine(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Line
context.setLineWidth(2.0)
context.setStrokeColor(UIColor.red.cgColor)
context.move(to: CGPoint(x: 70, y: 50))
context.addLine(to: CGPoint(x: 270, y: 250))
context.strokePath()
// Draw Triangle
context.setLineWidth(4.0)
context.setStrokeColor(UIColor.blue.cgColor)
context.move(to: CGPoint(x: 170, y: 200))
context.addLine(to: CGPoint(x: 270, y: 350))
context.addLine(to: CGPoint(x: 70, y: 350))
context.addLine(to: CGPoint(x: 170, y: 200))
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
@IBAction func btnDrawRectangle(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Rectangle
context.setLineWidth(2.0)
context.setStrokeColor(UIColor.red.cgColor)
context.addRect(CGRect(x: 70, y: 100, width: 200, height: 200))
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
@IBAction func btnDrawCircle(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Ellipse
context.setLineWidth(2.0)
context.setStrokeColor(UIColor.red.cgColor)
context.addEllipse(in: CGRect(x: 70, y: 50, width: 200, height: 100))
context.strokePath()
// Draw Circle
context.setLineWidth(5.0)
context.setStrokeColor(UIColor.green.cgColor)
context.addEllipse(in: CGRect(x: 70, y: 200, width: 200, height: 200))
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
@IBAction func btnDrawArc(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Arc
context.setLineWidth(5.0)
context.setStrokeColor(UIColor.red.cgColor)
context.move(to: CGPoint(x: 100, y: 50))
context.addArc(tangent1End: CGPoint(x: 250, y:50), tangent2End: CGPoint(x:250, y:200), radius: CGFloat(50))
context.addLine(to: CGPoint(x: 250, y: 200))
context.move(to: CGPoint(x: 100, y: 250))
context.addArc(tangent1End: CGPoint(x: 270, y:250), tangent2End: CGPoint(x:100, y:400), radius: CGFloat(20))
context.addLine(to: CGPoint(x: 100, y: 400))
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
@IBAction func btnDrawFill(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Rectangle
context.setLineWidth(1.0)
context.setStrokeColor(UIColor.red.cgColor)
context.setFillColor(UIColor.red.cgColor)
let rectangel = CGRect(x: 70, y: 50, width: 200, height: 100)
context.addRect(rectangel)
context.fill(rectangel)
context.strokePath()
// Draw Circle
context.setLineWidth(1.0)
context.setStrokeColor(UIColor.blue.cgColor)
context.setFillColor(UIColor.blue.cgColor)
let circle = CGRect(x: 70, y: 200, width: 200, height: 100)
context.addEllipse(in: circle)
context.fillEllipse(in: circle)
context.strokePath()
// Draw Triangle
context.setLineWidth(1.0)
context.setStrokeColor(UIColor.green.cgColor)
context.setFillColor(UIColor.green.cgColor)
context.move(to: CGPoint(x: 170, y: 350))
context.addLine(to: CGPoint(x: 270, y: 450))
context.addLine(to: CGPoint(x: 70, y: 450))
context.addLine(to: CGPoint(x: 170, y: 350))
context.fillPath()
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
}
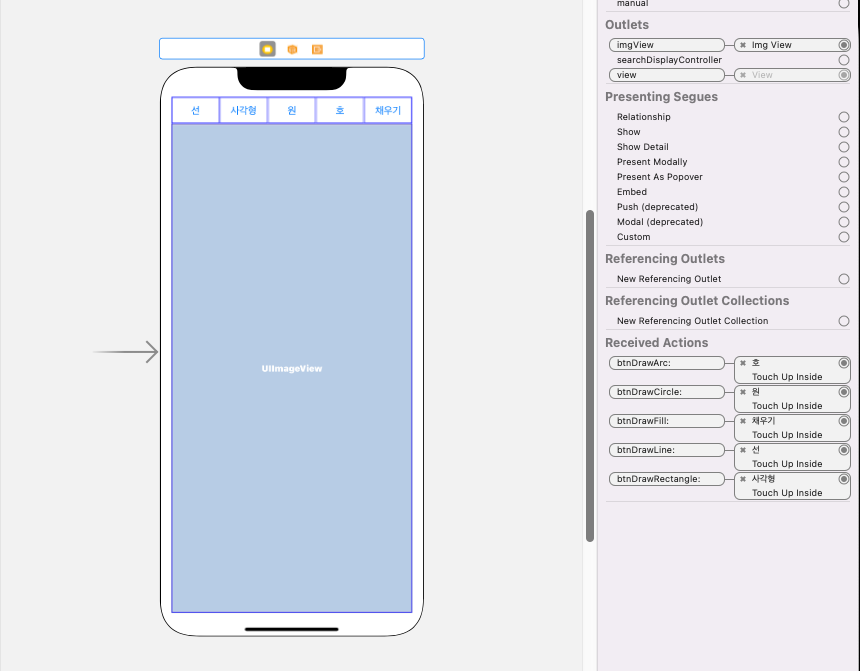
17강
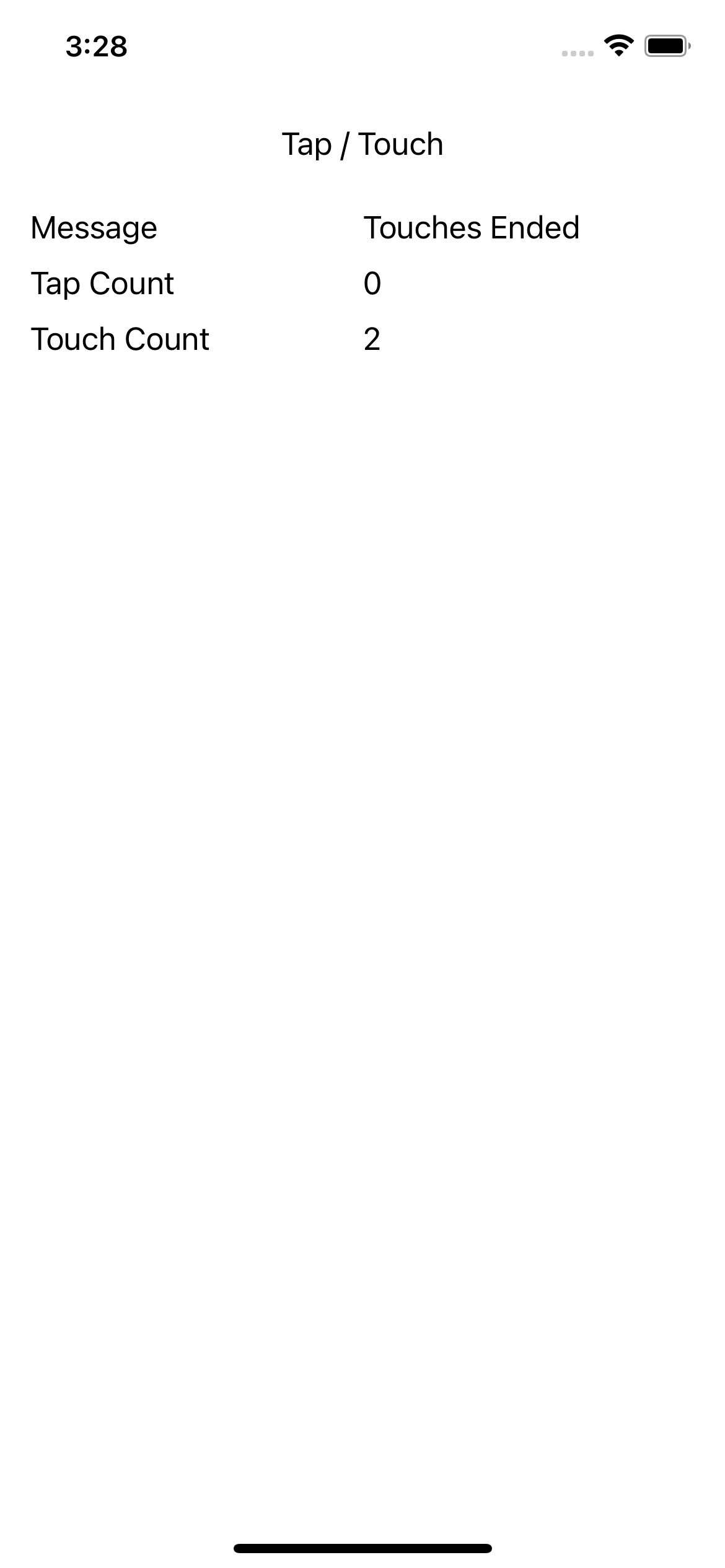
//
// ViewController.swift
// TapTouch
//
// Created by Ho-Jeong Song on 2021/12/01.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var txtMessage: UILabel!
@IBOutlet var txtTapCount: UILabel!
@IBOutlet var txtTouchCount: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
let touch = touches.first! as UITouch
txtMessage.text = "Touches Began"
txtTapCount.text = String(touch.tapCount)
txtTouchCount.text = String(touches.count)
}
override func touchesMoved(_ touches: Set<UITouch>, with event: UIEvent?) {
let touch = touches.first! as UITouch
txtMessage.text = "Touches Moved"
txtTapCount.text = String(touch.tapCount)
txtTouchCount.text = String(touches.count)
}
override func touchesEnded(_ touches: Set<UITouch>, with event: UIEvent?) {
let touch = touches.first! as UITouch
txtMessage.text = "Touches Ended"
txtTapCount.text = String(touch.tapCount)
txtTouchCount.text = String(touches.count)
}
}

18강
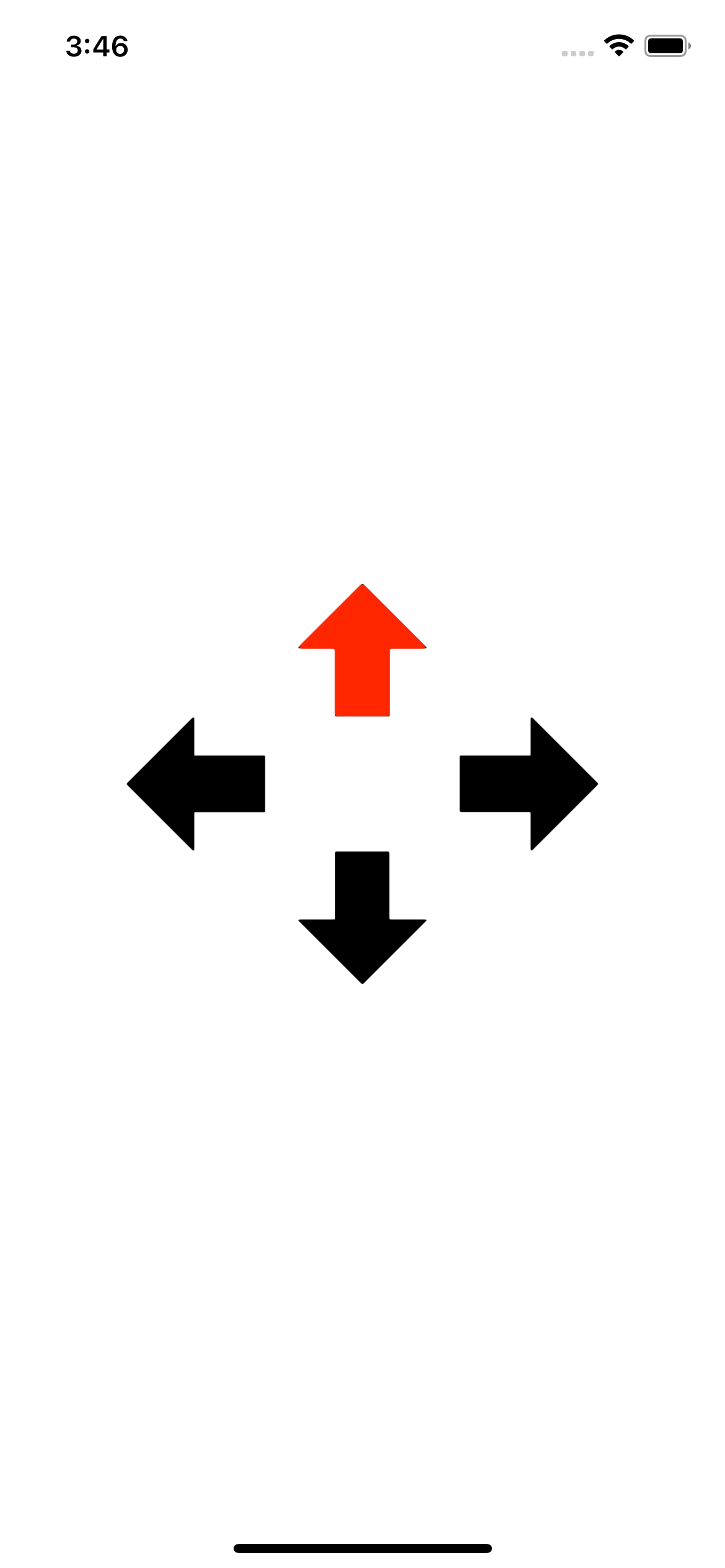
//
// ViewController.swift
// SwipeGesture
//
// Created by Ho-Jeong Song on 2021/12/02.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var imgViewUp: UIImageView!
@IBOutlet var imgViewDown: UIImageView!
@IBOutlet var imgViewLeft: UIImageView!
@IBOutlet var imgViewRight: UIImageView!
var imgLeft = [UIImage]()
var imgRight = [UIImage]()
var imgUp = [UIImage]()
var imgDown = [UIImage]()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
imgUp.append(UIImage(named: "arrow-up-black.png")!)
imgUp.append(UIImage(named: "arrow-up-red.png")!)
imgDown.append(UIImage(named: "arrow-down-black.png")!)
imgDown.append(UIImage(named: "arrow-down-red.png")!)
imgLeft.append(UIImage(named: "arrow-left-black.png")!)
imgLeft.append(UIImage(named: "arrow-left-red.png")!)
imgRight.append(UIImage(named: "arrow-right-black.png")!)
imgRight.append(UIImage(named: "arrow-right-red.png")!)
imgViewUp.image = imgUp[0]
imgViewDown.image = imgDown[0]
imgViewLeft.image = imgLeft[0]
imgViewRight.image = imgRight[0]
let swipeUp = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.respondToSwipeGesture(_:)))
swipeUp.direction = UISwipeGestureRecognizer.Direction.up
self.view.addGestureRecognizer(swipeUp)
let swipeDown = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.respondToSwipeGesture(_:)))
swipeDown.direction = UISwipeGestureRecognizer.Direction.down
self.view.addGestureRecognizer(swipeDown)
let swipeLeft = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.respondToSwipeGesture(_:)))
swipeLeft.direction = UISwipeGestureRecognizer.Direction.left
self.view.addGestureRecognizer(swipeLeft)
let swipeRight = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.respondToSwipeGesture(_:)))
swipeRight.direction = UISwipeGestureRecognizer.Direction.right
self.view.addGestureRecognizer(swipeRight)
}
@objc func respondToSwipeGesture(_ gesture: UIGestureRecognizer) {
if let swipeGesture = gesture as? UISwipeGestureRecognizer {
imgViewUp.image = imgUp[0]
imgViewDown.image = imgDown[0]
imgViewLeft.image = imgLeft[0]
imgViewRight.image = imgRight[0]
switch swipeGesture.direction {
case UISwipeGestureRecognizer.Direction.up:
imgViewUp.image = imgUp[1]
case UISwipeGestureRecognizer.Direction.down:
imgViewDown.image = imgDown[1]
case UISwipeGestureRecognizer.Direction.left:
imgViewLeft.image = imgLeft[1]
case UISwipeGestureRecognizer.Direction.right:
imgViewRight.image = imgRight[1]
default:
break
}
}
}
}

19강

//
// ViewController.swift
// PinchGesture
//
// Created by Ho-Jeong Song on 2021/12/02.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var txtPinch: UILabel!
var initialFontSize:CGFloat!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
let pinch = UIPinchGestureRecognizer(target: self, action: #selector(ViewController.doPinch(_:)))
self.view.addGestureRecognizer(pinch)
}
@objc func doPinch(_ pinch: UIPinchGestureRecognizer) {
if (pinch.state == UIGestureRecognizer.State.began) {
initialFontSize = txtPinch.font.pointSize
} else {
txtPinch.font = txtPinch.font.withSize(initialFontSize * pinch.scale)
}
}
}
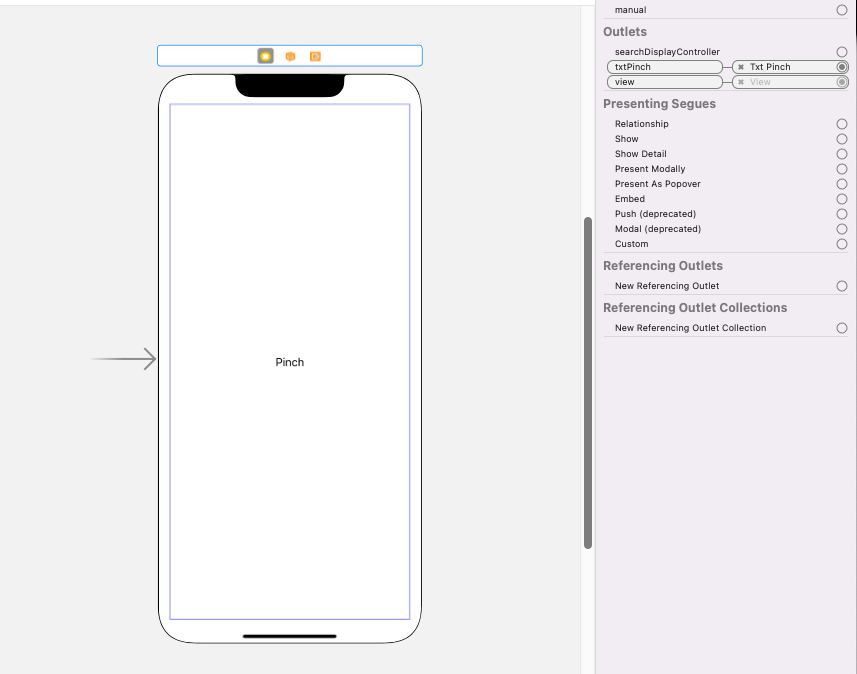
BMI 실습 소스
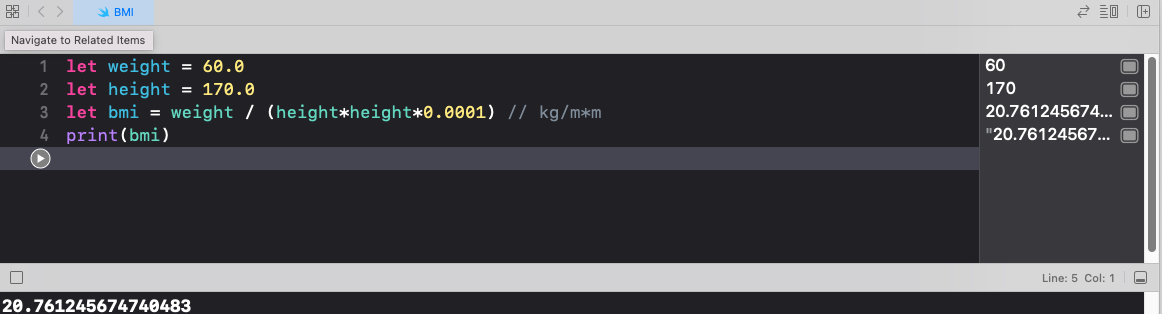
let weight = 60.0
let height = 170.0
let bmi = weight / (height*height*0.0001) // kg/m*m
print(bmi)
import Foundation
let weight = 60.0
let height = 170.0
let bmi = weight / (height*height*0.0001) // kg/m*m
let shortenedBmi = String(format: "%.2f", bmi) // 더블형을 문자열로 바꿔 저장
print(bmi, shortenedBmi)
//20.761245674740483 20.8
import Foundation
class BMI {
var weight : Double
var height : Double
init(weight:Double, height:Double){
self.height = height
self.weight = weight
}
func calcBMI() -> String {
let bmi=weight/(height*height*0.0001)// kg/m*m
let shortenedBmi = String(format: "%.1f", bmi)
var body = ""
if bmi >= 40{
body = "3단계 비만"
} else if bmi >= 30 && bmi < 40 {
body = "2단계 비만"
} else if bmi >= 25 && bmi < 30 {
body = "1단계 비만"
} else if bmi >= 18.5 && bmi < 25 {
body = "정상"
} else {
body = "저체중"
}
return "BMI:\(shortenedBmi), 판정:\(body)"
}
}
var han = BMI(weight:62.5, height:172.3)
print(han.calcBMI())
// BMI를 도출하는 클레스 생성자를 이용하요 값을 넣고 클레스 메소드를 이용하여 판정 결과 추출
//BMI:21.1, 판정:정상
728x90
'APP > iOS' 카테고리의 다른 글
MapKit with SwiftUI 기초부터 심화까지 1 : MapKit 다루기 기본 과정 (0) | 2024.01.08 |
---|---|
iOS23 SkillUpThon 2주차 정리 (0) | 2023.06.22 |
iOS23 SkillUpThon 1주차 정리 (0) | 2023.06.21 |
앱의 생명주기(Life Cycle) (0) | 2023.04.16 |
iOS 프로그래밍 : Event Driven Programming (0) | 2022.11.18 |
728x90
2장
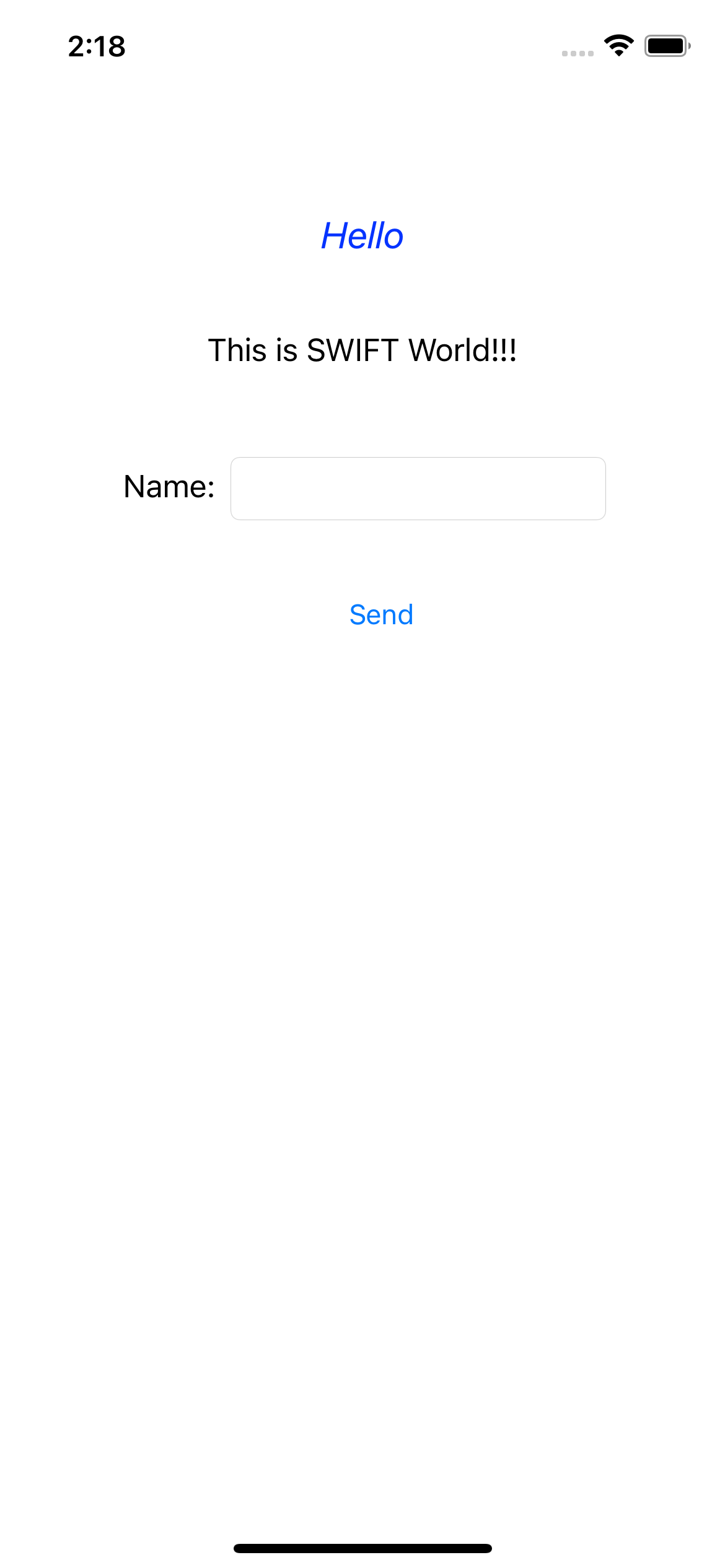
//
// ViewController.swift
// HelloWorld
//
// Created by Ho-Jeong Song on 2021/11/09.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var lblHello: UILabel!
@IBOutlet var txtName: UITextField!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnSend(_ sender: UIButton) {
lblHello.text = "Hello, " + txtName.text!
}
}
연결 관계
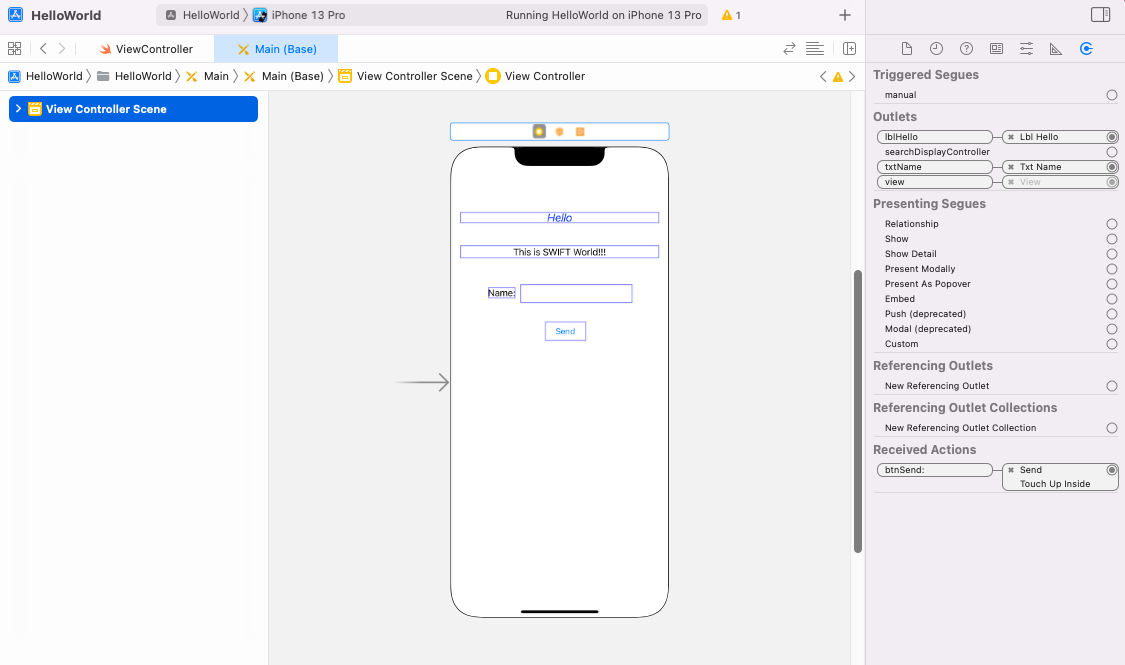
3강 본문 실습
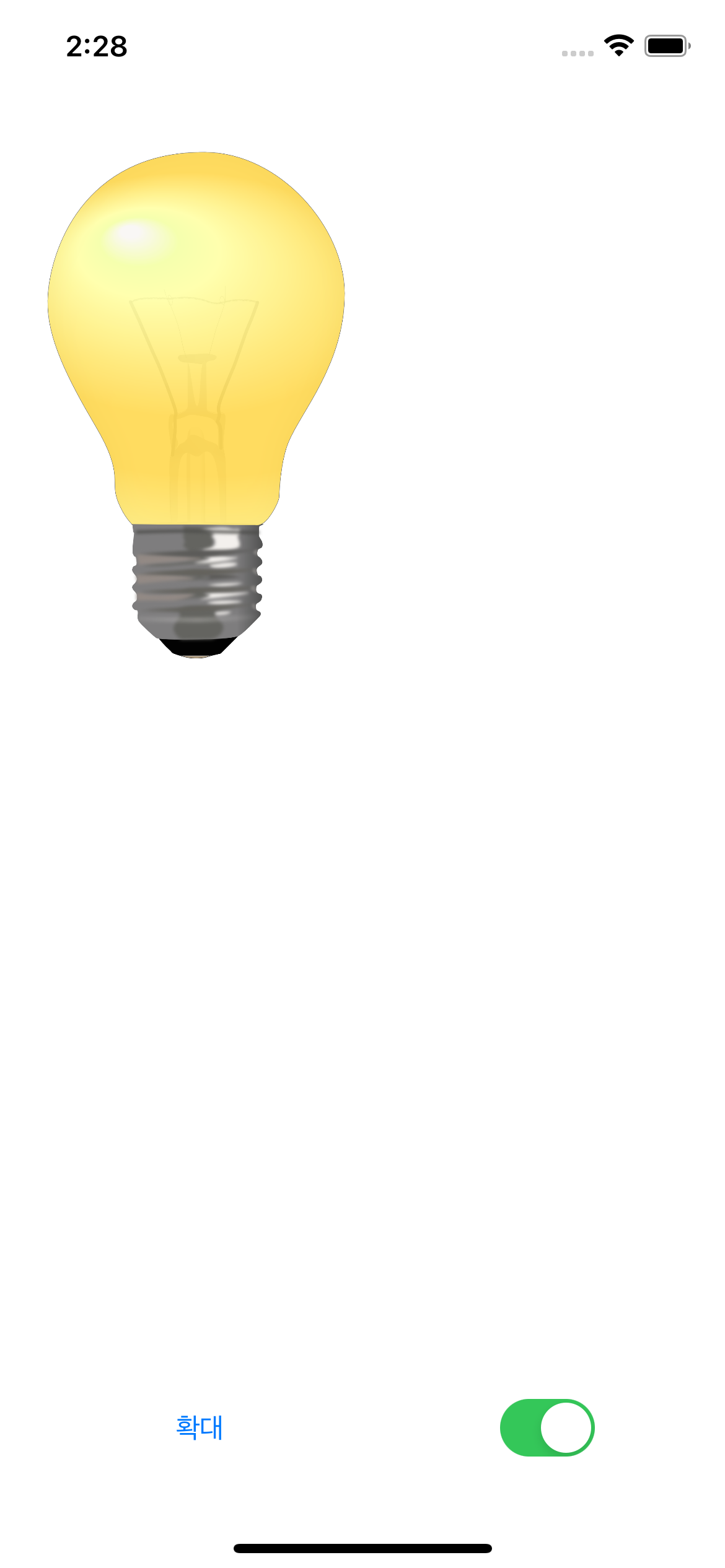
//
// ViewController.swift
// ImageView
//
// Created by Ho-Jeong Song on 2021/11/23.
//
import UIKit
class ViewController: UIViewController {
var isZoom = false
var imgOn: UIImage?
var imgOff: UIImage?
@IBOutlet var imgView: UIImageView!
@IBOutlet var btnResize: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
imgOn = UIImage(named: "lamp_on.png")
imgOff = UIImage(named: "lamp_off.png")
imgView.image = imgOn
}
@IBAction func btnResizeImage(_ sender: UIButton) {
let scale: CGFloat = 2.0
var newWidth: CGFloat, newHeight: CGFloat
if (isZoom) { // true
newWidth = imgView.frame.width / scale
newHeight = imgView.frame.height / scale
btnResize.setTitle("확대", for: .normal)
}
else { // false
newWidth = imgView.frame.width * scale
newHeight = imgView.frame.height * scale
btnResize.setTitle("축소", for: .normal)
}
imgView.frame.size = CGSize(width: newWidth, height: newHeight)
isZoom = !isZoom
}
@IBAction func switchImageOnOff(_ sender: UISwitch) {
if sender.isOn {
imgView.image = imgOn
} else {
imgView.image = imgOff
}
}
}
연결관계

4장 본문 실습 (데이트 타임 픽커)
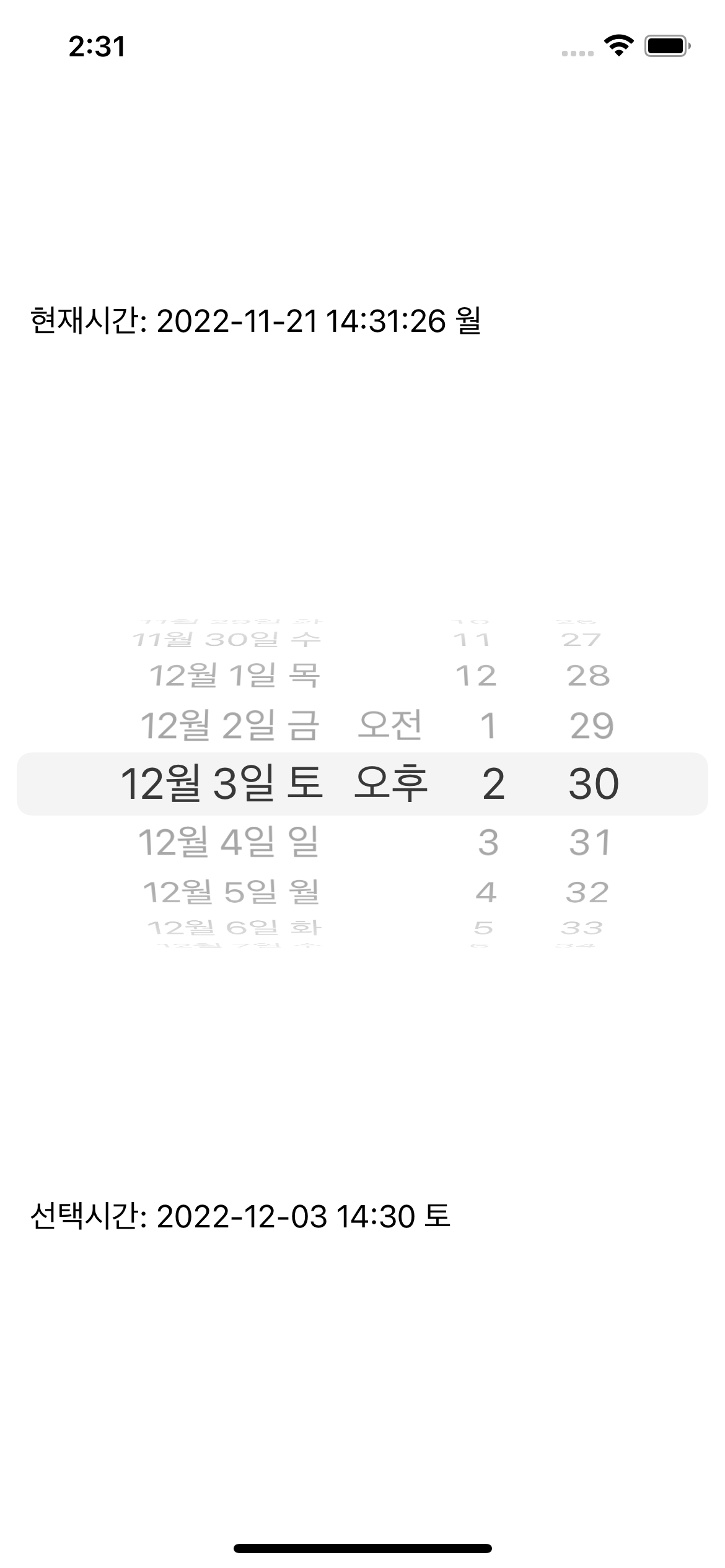
//
// ViewController.swift
// DatePicker
//
// Created by Ho-Jeong Song on 2021/11/24.
//
import UIKit
class ViewController: UIViewController {
let timeSelector: Selector = #selector(ViewController.updateTime)
let interval = 1.0
var count = 0
@IBOutlet var lblCurrentTime: UILabel!
@IBOutlet var lblPickerTime: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
Timer.scheduledTimer(timeInterval: interval, target: self, selector: timeSelector, userInfo: nil, repeats: true)
}
@IBAction func changeDatePicker(_ sender: UIDatePicker) {
let datePickerView = sender
let formatter = DateFormatter()
formatter.dateFormat = "yyyy-MM-dd HH:mm EEE"
lblPickerTime.text = "선택시간: " + formatter.string(from: datePickerView.date)
}
@objc func updateTime() {
// lblCurrentTime.text = String(count)
// count = count + 1
let date = NSDate()
let formatter = DateFormatter()
formatter.dateFormat = "yyyy-MM-dd HH:mm:ss EEE"
lblCurrentTime.text = "현재시간: " + formatter.string(from: date as Date)
}
}
연결 관계
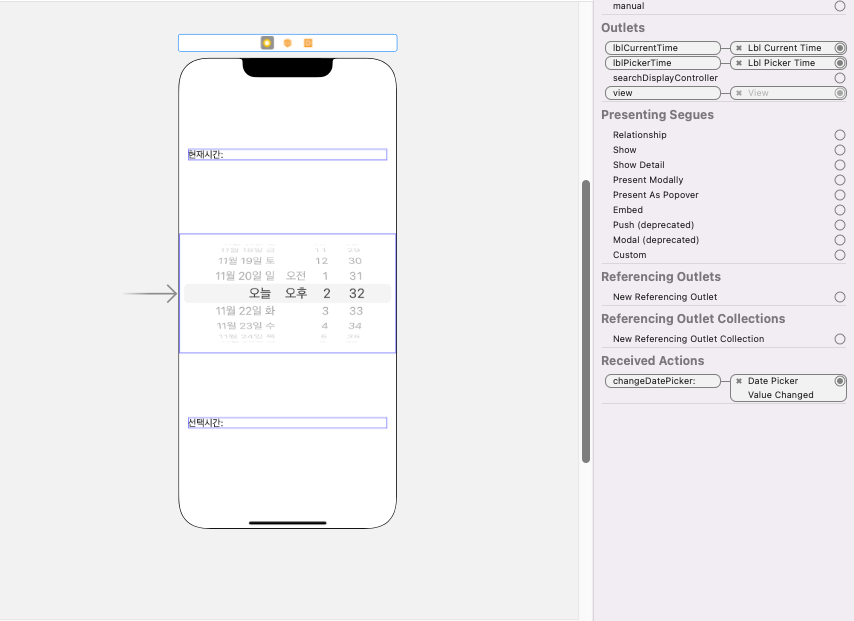
5강 본문 실습 픽커뷰
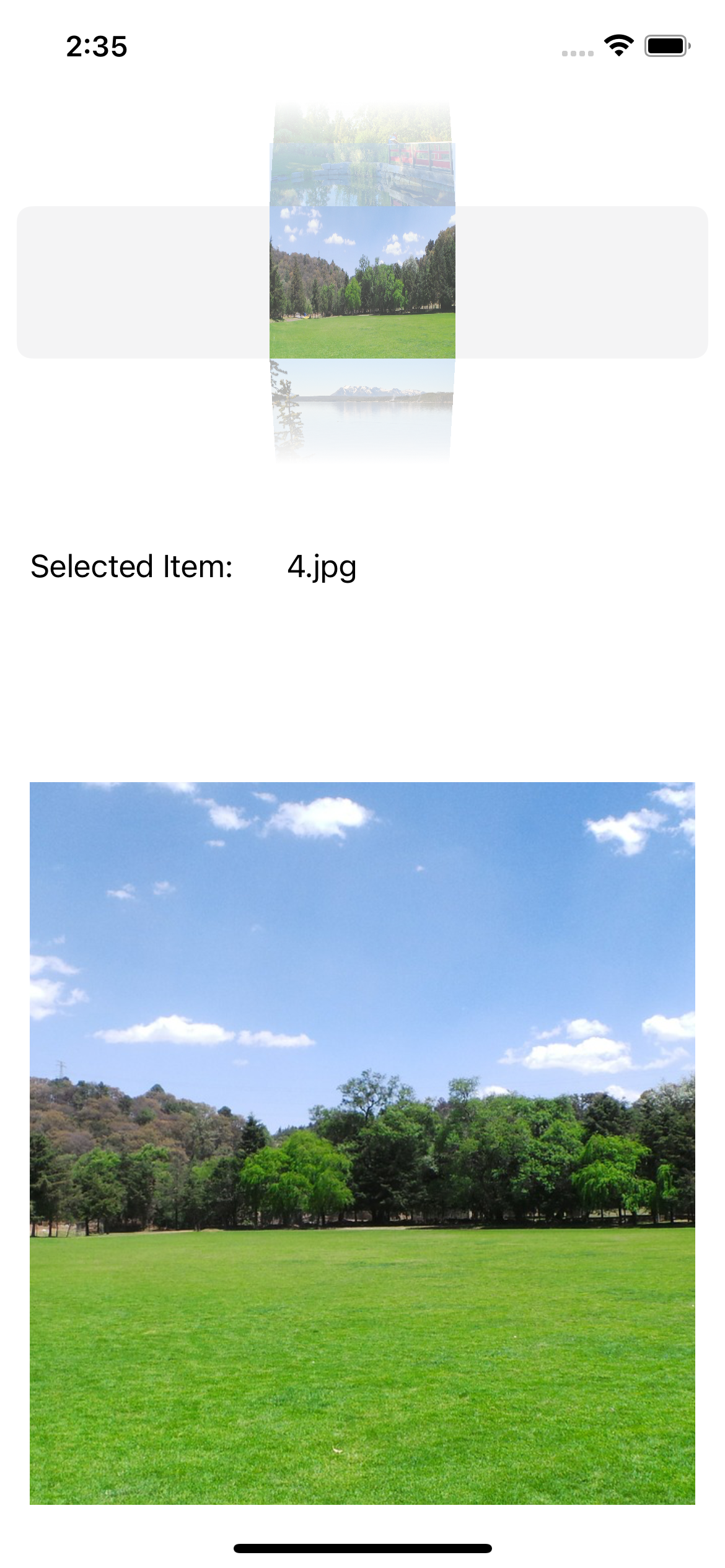
//
// ViewController.swift
// PickerView
//
// Created by Ho-Jeong Song on 2021/11/25.
//
import UIKit
class ViewController: UIViewController, UIPickerViewDelegate, UIPickerViewDataSource {
let MAX_ARRAY_NUM = 10
let PICKER_VIEW_COLUMN = 1
let PICKER_VIEW_HEIGHT:CGFloat = 80
var imageArray = [UIImage?]()
var imageFileName = [ "1.jpg", "2.jpg", "3.jpg", "4.jpg", "5.jpg",
"6.jpg", "7.jpg", "8.jpg", "9.jpg", "10.jpg" ]
@IBOutlet var pickerImage: UIPickerView!
@IBOutlet var lblImageFileName: UILabel!
@IBOutlet var imageView: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
for i in 0 ..< MAX_ARRAY_NUM {
let image = UIImage(named: imageFileName[i])
imageArray.append(image)
}
lblImageFileName.text = imageFileName[0]
imageView.image = imageArray[0]
}
// returns the number of 'columns' to display.
func numberOfComponents(in pickerView: UIPickerView) -> Int {
return PICKER_VIEW_COLUMN
}
// returns height of row for each component.
func pickerView(_ pickerView: UIPickerView, rowHeightForComponent component: Int) -> CGFloat {
return PICKER_VIEW_HEIGHT
}
// returns the # of rows in each component..
func pickerView(_ pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int {
return imageFileName.count
}
// func pickerView(_ pickerView: UIPickerView, titleForRow row: Int, forComponent component: Int) -> String? {
// return imageFileName[row]
// }
func pickerView(_ pickerView: UIPickerView, viewForRow row: Int, forComponent component: Int, reusing view: UIView?) -> UIView {
let imageView = UIImageView(image:imageArray[row])
imageView.frame = CGRect(x: 0, y: 0, width: 100, height: 150)
return imageView
}
func pickerView(_ pickerView: UIPickerView, didSelectRow row: Int, inComponent component: Int) {
lblImageFileName.text = imageFileName[row]
imageView.image = imageArray[row]
}
}
연결 관계
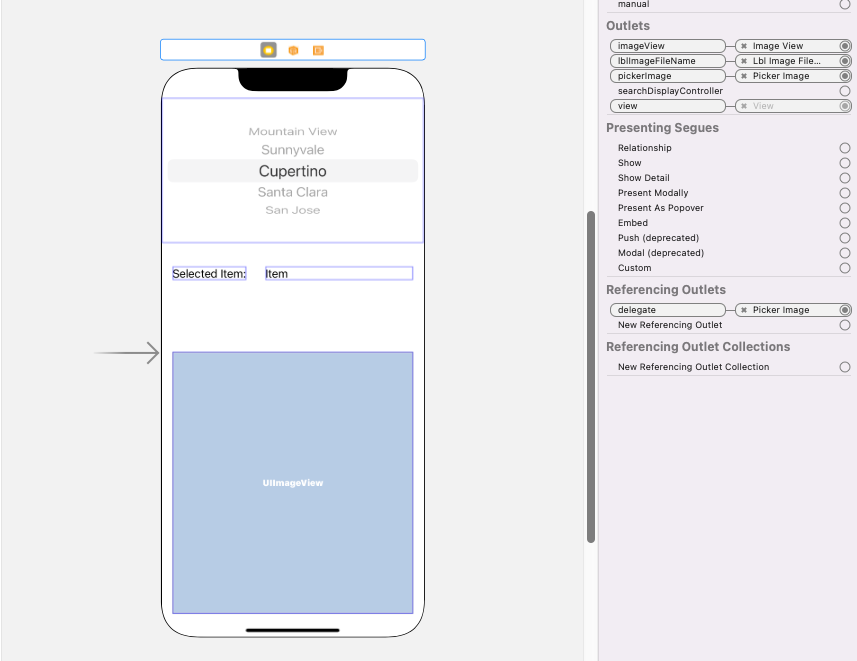
6강 경고창
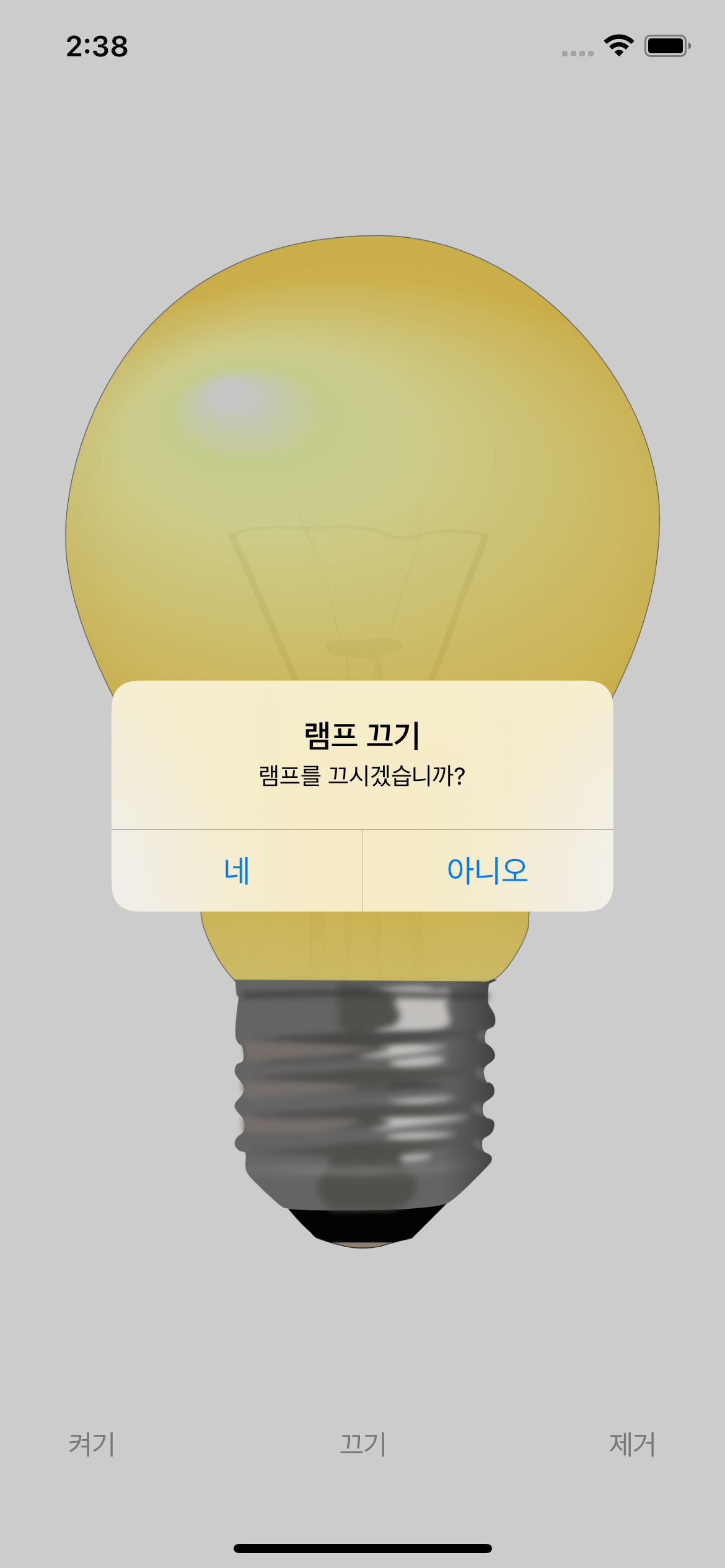
//
// ViewController.swift
// Alert
//
// Created by BeomGeun Lee on
//
import UIKit
class ViewController: UIViewController {
let imgOn = UIImage(named: "lamp-on.png")
let imgOff = UIImage(named: "lamp-off.png")
let imgRemove = UIImage(named: "lamp-remove.png")
var isLampOn = true
@IBOutlet var lampImg: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
lampImg.image = imgOn
}
@IBAction func btnLampOn(_ sender: UIButton) {
if(isLampOn==true) {
let lampOnAlert = UIAlertController(title: "경고", message: "현재 On 상태입니다", preferredStyle: UIAlertController.Style.alert)
let onAction = UIAlertAction(title: "네, 알겠습니다.", style: UIAlertAction.Style.default, handler: nil)
lampOnAlert.addAction(onAction)
present(lampOnAlert, animated: true, completion: nil)
} else {
lampImg.image = imgOn
isLampOn=true
}
}
@IBAction func btnLanpOff(_ sender: UIButton) {
if isLampOn {
let lampOffAlert = UIAlertController(title: "램프 끄기", message: "램프를 끄시겠습니까?", preferredStyle: UIAlertController.Style.alert)
let offAction = UIAlertAction(title: "네", style: UIAlertAction.Style.default, handler: {
ACTION in self.lampImg.image = self.imgOff
self.isLampOn=false
})
let cancelAction = UIAlertAction(title: "아니오", style: UIAlertAction.Style.default, handler: nil)
lampOffAlert.addAction(offAction)
lampOffAlert.addAction(cancelAction)
present(lampOffAlert, animated: true, completion: nil)
}
}
@IBAction func btnLampRemove(_ sender: UIButton) {
let lampRemoveAlert = UIAlertController(title: "램프 제거", message: "램프를 제거하시겠습니까?", preferredStyle: UIAlertController.Style.alert)
let offAction = UIAlertAction(title: "아니오, 끕니다(off).", style: UIAlertAction.Style.default, handler: {
ACTION in self.lampImg.image = self.imgOff
self.isLampOn=false
})
let onAction = UIAlertAction(title: "아니오, 켭니다(on).", style: UIAlertAction.Style.default) {
ACTION in self.lampImg.image = self.imgOn
self.isLampOn=true
}
let removeAction = UIAlertAction(title: "네, 제거합니다.", style: UIAlertAction.Style.destructive, handler: {
ACTION in self.lampImg.image = self.imgRemove
self.isLampOn=false
})
lampRemoveAlert.addAction(offAction)
lampRemoveAlert.addAction(onAction)
lampRemoveAlert.addAction(removeAction)
present(lampRemoveAlert, animated: true, completion: nil)
}
}
연결 관계
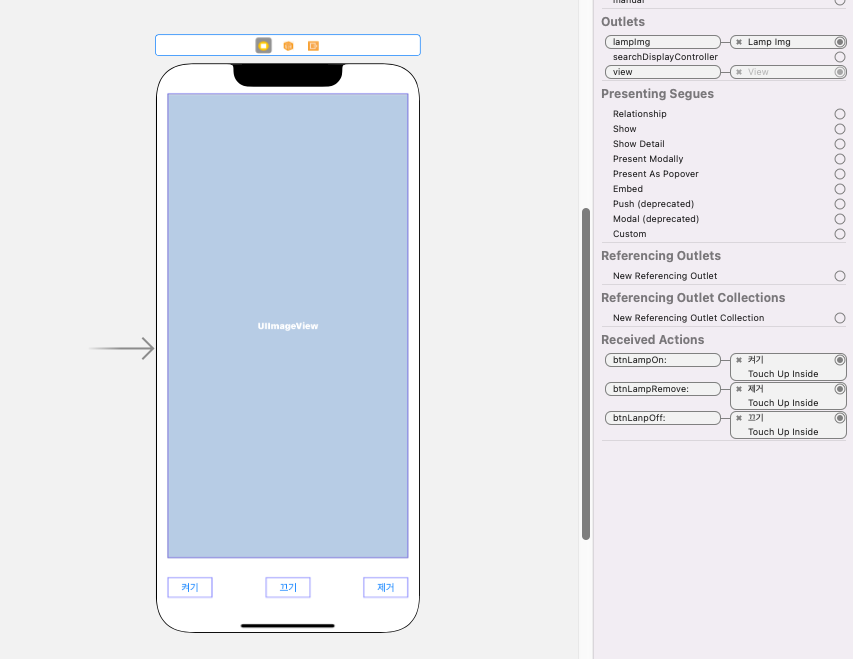
7강 본문실습 내장형 웹브라우저 삽입
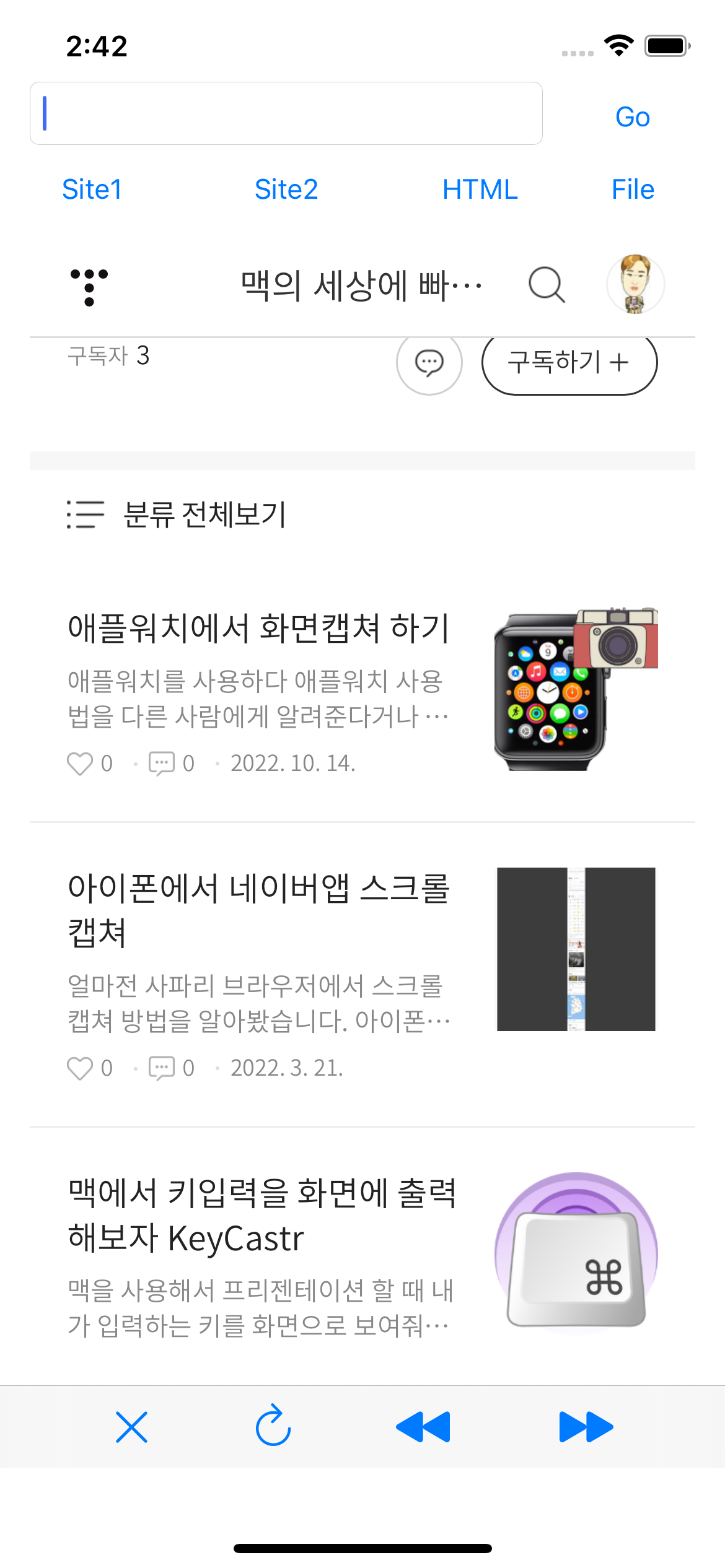
//
// ViewController.swift
// Web
//
// Created by BeomGeun Lee on
//
import UIKit
import WebKit
class ViewController: UIViewController, WKNavigationDelegate {
@IBOutlet var txtUrl: UITextField!
@IBOutlet var myWebView: WKWebView!
@IBOutlet var myActivityIndicator: UIActivityIndicatorView!
func loadWebPage(_ url: String) {
let myUrl = URL(string: url)
let myRequest = URLRequest(url: myUrl!)
myWebView.load(myRequest)
}
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
myWebView.navigationDelegate = self
loadWebPage("http://2sam.net")
}
func webView(_ webView: WKWebView, didCommit navigation: WKNavigation!) {
myActivityIndicator.startAnimating()
myActivityIndicator.isHidden = false
}
func webView(_ webView: WKWebView, didFinish navigation: WKNavigation!) {
myActivityIndicator.stopAnimating()
myActivityIndicator.isHidden = true
}
func webView(_ webView: WKWebView, didFail navigation: WKNavigation!, withError error: Error) {
myActivityIndicator.stopAnimating()
myActivityIndicator.isHidden = true
}
func checkUrl(_ url: String) -> String {
var strUrl = url
let flag = strUrl.hasPrefix("http://")
if !flag {
strUrl = "http://" + strUrl
}
return strUrl
}
@IBAction func btnGotoUrl(_ sender: UIButton) {
let myUrl = checkUrl(txtUrl.text!)
txtUrl.text = ""
loadWebPage(myUrl)
}
@IBAction func btnGoSite1(_ sender: UIButton) {
loadWebPage("http://fallinmac.tistory.com")
}
@IBAction func btnGoSite2(_ sender: UIButton) {
loadWebPage("http://blog.2sam.net")
}
@IBAction func btnLoadHtmlString(_ sender: UIButton) {
let htmlString = "<h1> HTML String </h1><p> String 변수를 이용한 웹페이지 </p> <p><a href=\"http://2sam.net\">2sam</a>으로 이동</p>"
myWebView.loadHTMLString(htmlString, baseURL: nil)
}
@IBAction func btnLoadHtmlFile(_ sender: UIButton) {
let filePath = Bundle.main.path(forResource: "htmlView", ofType: "html")
let myUrl = URL(fileURLWithPath: filePath!)
let myRequest = URLRequest(url: myUrl)
myWebView.load(myRequest)
}
@IBAction func btnStop(_ sender: UIBarButtonItem) {
myWebView.stopLoading()
}
@IBAction func btnReload(_ sender: UIBarButtonItem) {
myWebView.reload()
}
@IBAction func btnGoBack(_ sender: UIBarButtonItem) {
myWebView.goBack()
}
@IBAction func btnGoForward(_ sender: UIBarButtonItem) {
myWebView.goForward()
}
}
연결 관계
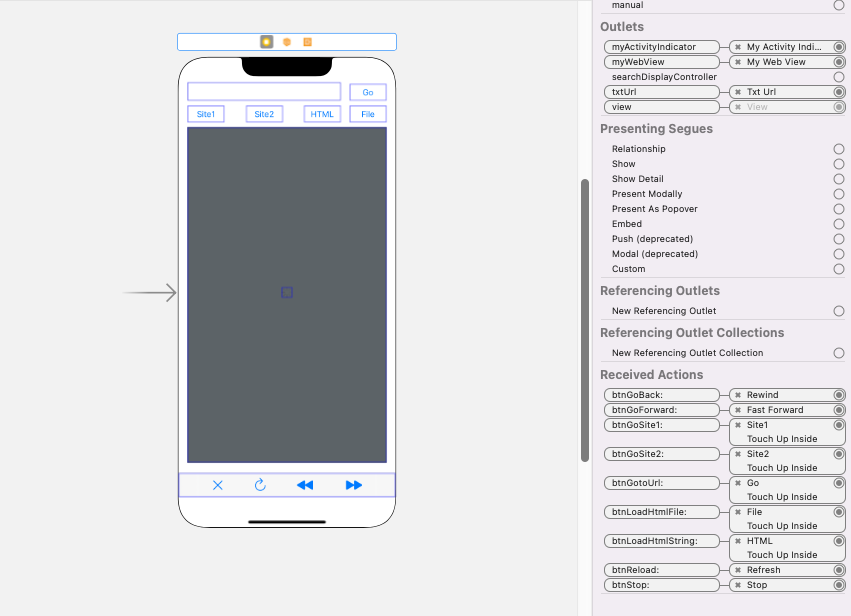
8강 지도 넘어가기
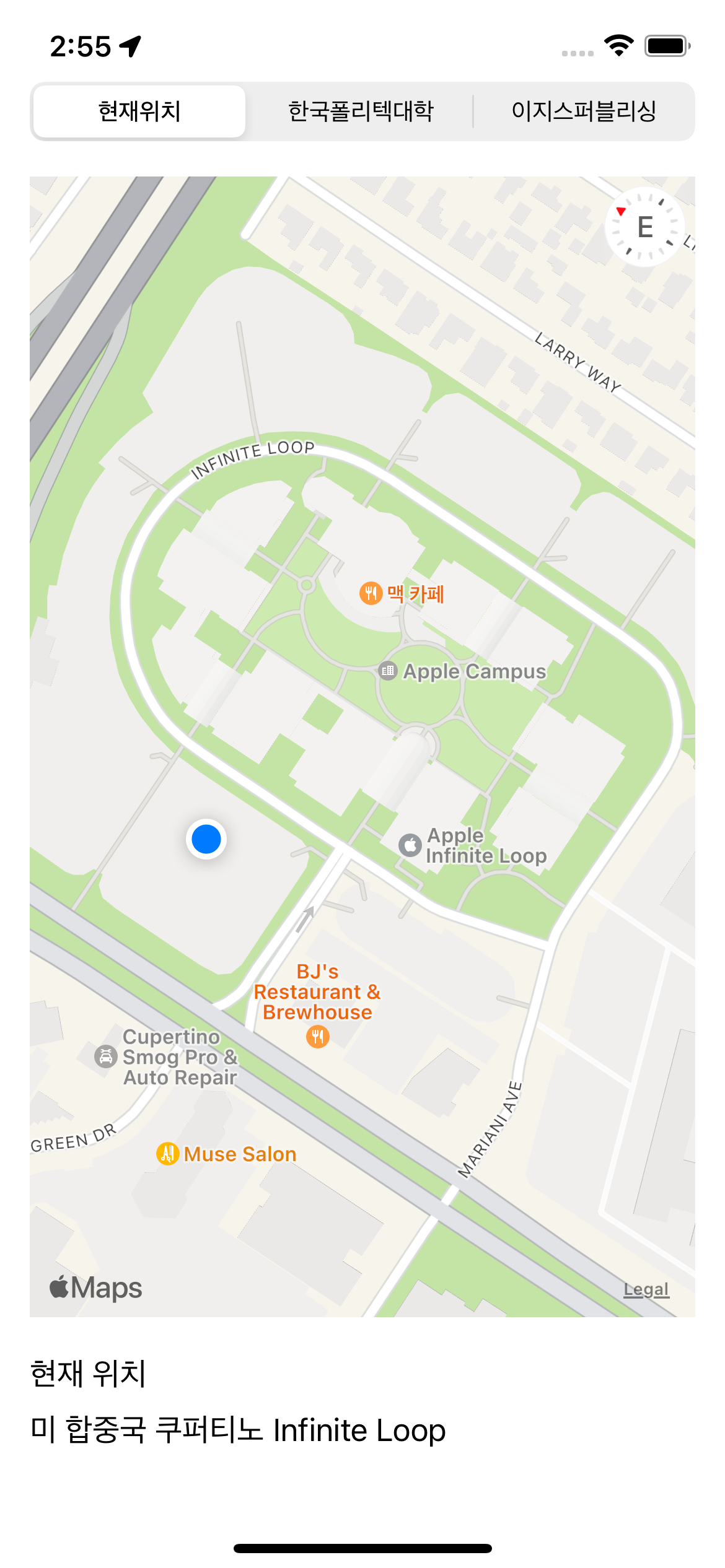
//
// ViewController.swift
// Map
//
// Created by BeomGeun Lee on 2021
//
import UIKit
import MapKit
class ViewController: UIViewController, CLLocationManagerDelegate {
@IBOutlet var myMap: MKMapView!
@IBOutlet var lblLocationInfo1: UILabel!
@IBOutlet var lblLocationInfo2: UILabel!
let locationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
lblLocationInfo1.text = ""
lblLocationInfo2.text = ""
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestWhenInUseAuthorization()
locationManager.startUpdatingLocation()
myMap.showsUserLocation = true
}
func goLocation(latitudeValue: CLLocationDegrees, longitudeValue : CLLocationDegrees, delta span :Double)-> CLLocationCoordinate2D {
let pLocation = CLLocationCoordinate2DMake(latitudeValue, longitudeValue)
let spanValue = MKCoordinateSpan(latitudeDelta: span, longitudeDelta: span)
let pRegion = MKCoordinateRegion(center: pLocation, span: spanValue)
myMap.setRegion(pRegion, animated: true)
return pLocation
}
func setAnnotation(latitudeValue: CLLocationDegrees, longitudeValue : CLLocationDegrees, delta span :Double, title strTitle: String, subtitle strSubtitle:String) {
let annotation = MKPointAnnotation()
annotation.coordinate = goLocation(latitudeValue: latitudeValue, longitudeValue: longitudeValue, delta: span)
annotation.title = strTitle
annotation.subtitle = strSubtitle
myMap.addAnnotation(annotation)
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
let pLocation = locations.last
_ = goLocation(latitudeValue: (pLocation?.coordinate.latitude)!, longitudeValue: (pLocation?.coordinate.longitude)!, delta: 0.01)
CLGeocoder().reverseGeocodeLocation(pLocation!, completionHandler: {
(placemarks, error) -> Void in
let pm = placemarks!.first
let country = pm!.country
var address:String = country!
if pm!.locality != nil {
address += " "
address += pm!.locality!
}
if pm!.thoroughfare != nil {
address += " "
address += pm!.thoroughfare!
}
self.lblLocationInfo1.text = "현재 위치"
self.lblLocationInfo2.text = address
})
locationManager.stopUpdatingLocation()
}
@IBAction func sgChangeLocation(_ sender: UISegmentedControl) {
if sender.selectedSegmentIndex == 0 {
self.lblLocationInfo1.text = ""
self.lblLocationInfo2.text = ""
locationManager.startUpdatingLocation()
} else if sender.selectedSegmentIndex == 1 {
setAnnotation(latitudeValue: 37.751853, longitudeValue: 128.87605740000004, delta: 1, title: "한국폴리텍대학 강릉캠퍼스", subtitle: "강원도 강릉시 남산초교길 121")
self.lblLocationInfo1.text = "보고 계신 위치"
self.lblLocationInfo2.text = "한국폴리텍대학 강릉캠퍼스"
} else if sender.selectedSegmentIndex == 2 {
setAnnotation(latitudeValue: 37.556876, longitudeValue: 126.914066, delta: 0.1, title: "이지스퍼블리싱", subtitle: "서울시 마포구 잔다리로 109 이지스 빌딩")
self.lblLocationInfo1.text = "보고 계신 위치"
self.lblLocationInfo2.text = "이지스퍼블리싱 출판사 "
}
}
}
연결 관계
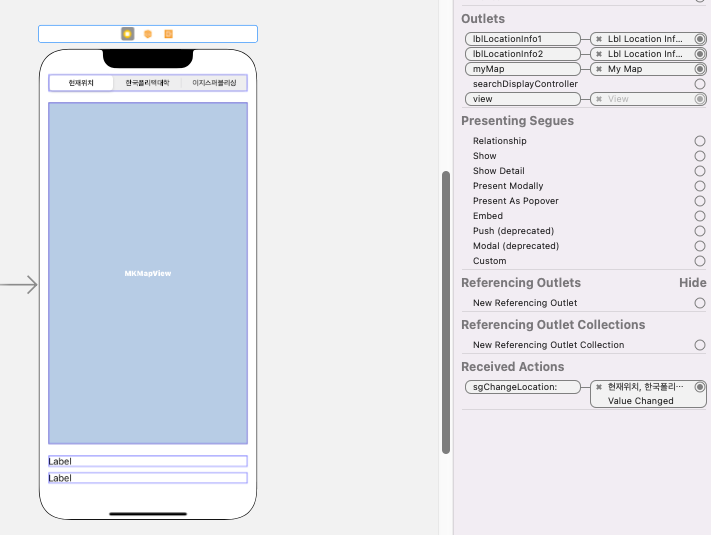

지도 이용시 이렇게 테스트 가능 , 시뮬레이터에서 art 누르면 확대 축소 가능
9강
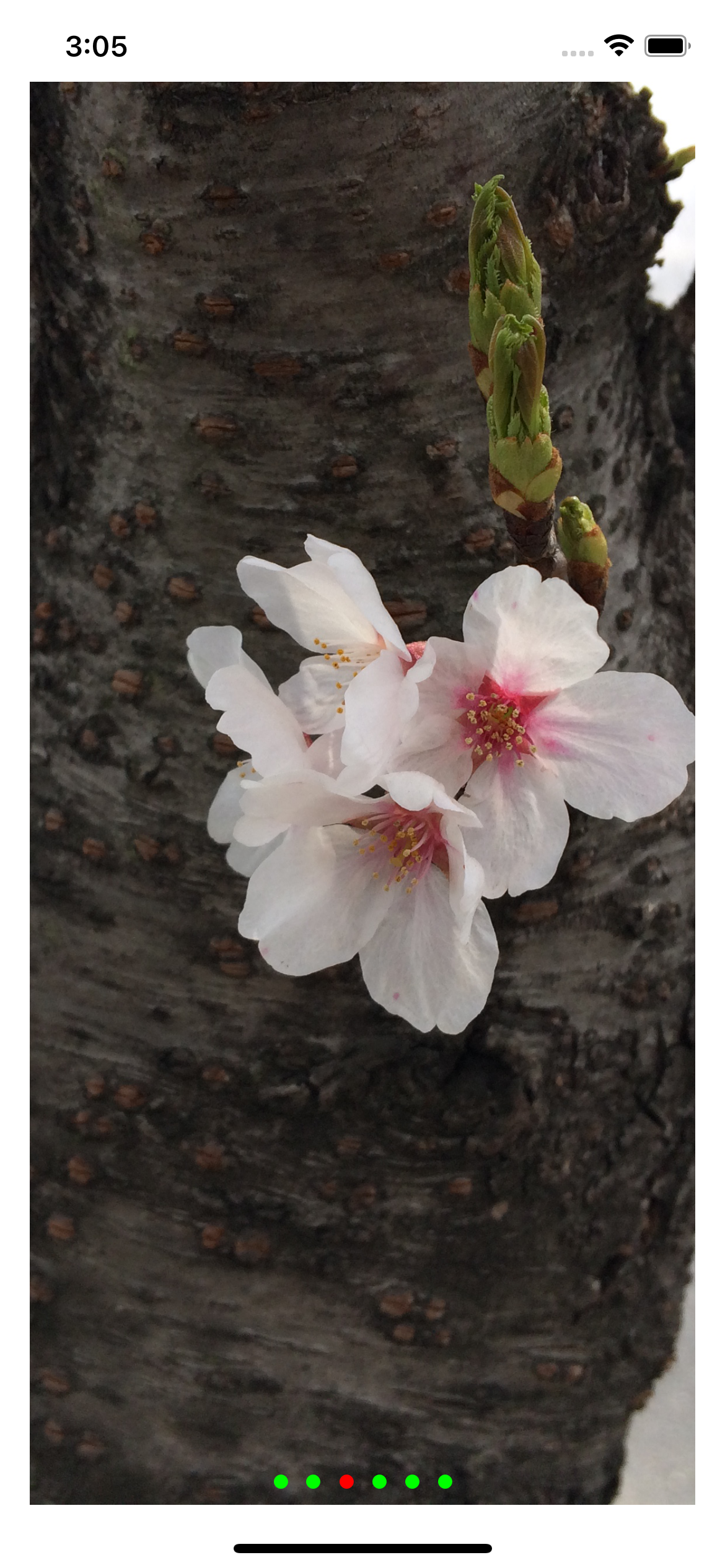
//
// ViewController.swift
// PageControl
//
// Created by Ho-Jeong Song on 2021/11/25.
//
import UIKit
var images = [ "01.png", "02.png", "03.png", "04.png", "05.png", "06.png" ]
class ViewController: UIViewController {
@IBOutlet var imgView: UIImageView!
@IBOutlet var pageControl: UIPageControl!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
pageControl.numberOfPages = images.count
pageControl.currentPage = 0
pageControl.pageIndicatorTintColor = UIColor.green
pageControl.currentPageIndicatorTintColor = UIColor.red
imgView.image = UIImage(named: images[0])
}
@IBAction func pageChange(_ sender: UIPageControl) {
imgView.image = UIImage(named: images[pageControl.currentPage])
}
}
연결관계
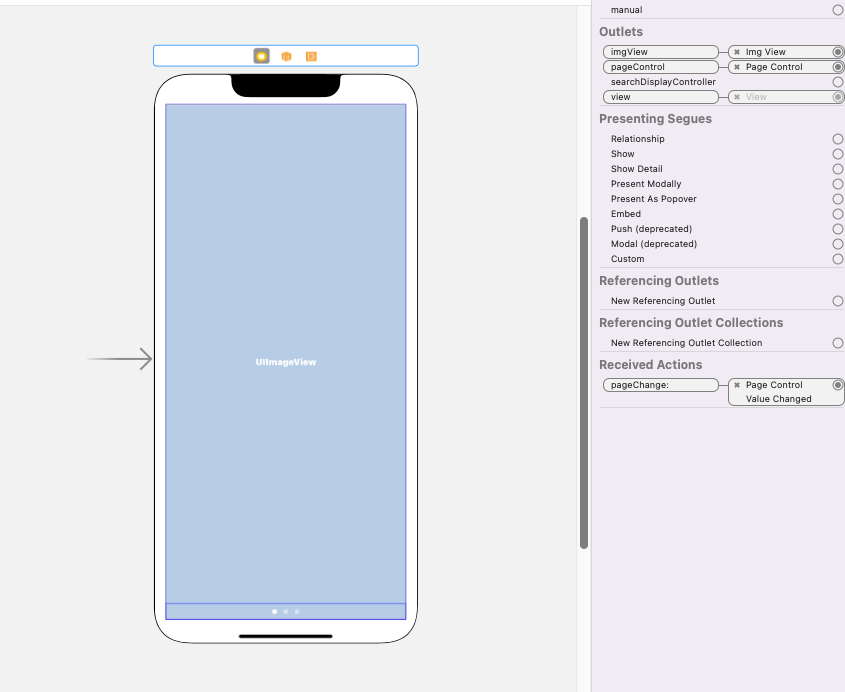
10강

//
// ViewController.swift
// Tab
//
// Created by BeomGeun Lee on 2021.
//
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnMoveImageView(_ sender: UIButton) {
tabBarController?.selectedIndex = 1
}
@IBAction func btnMoveDatePickerView(_ sender: UIButton) {
tabBarController?.selectedIndex = 2
}
}
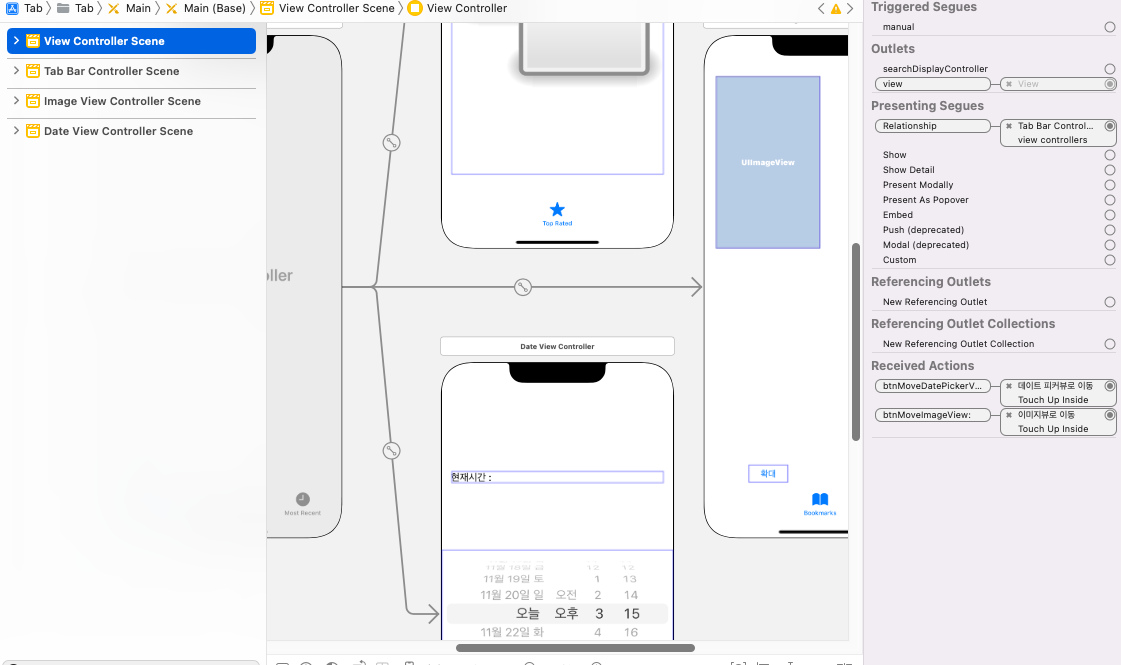
11강

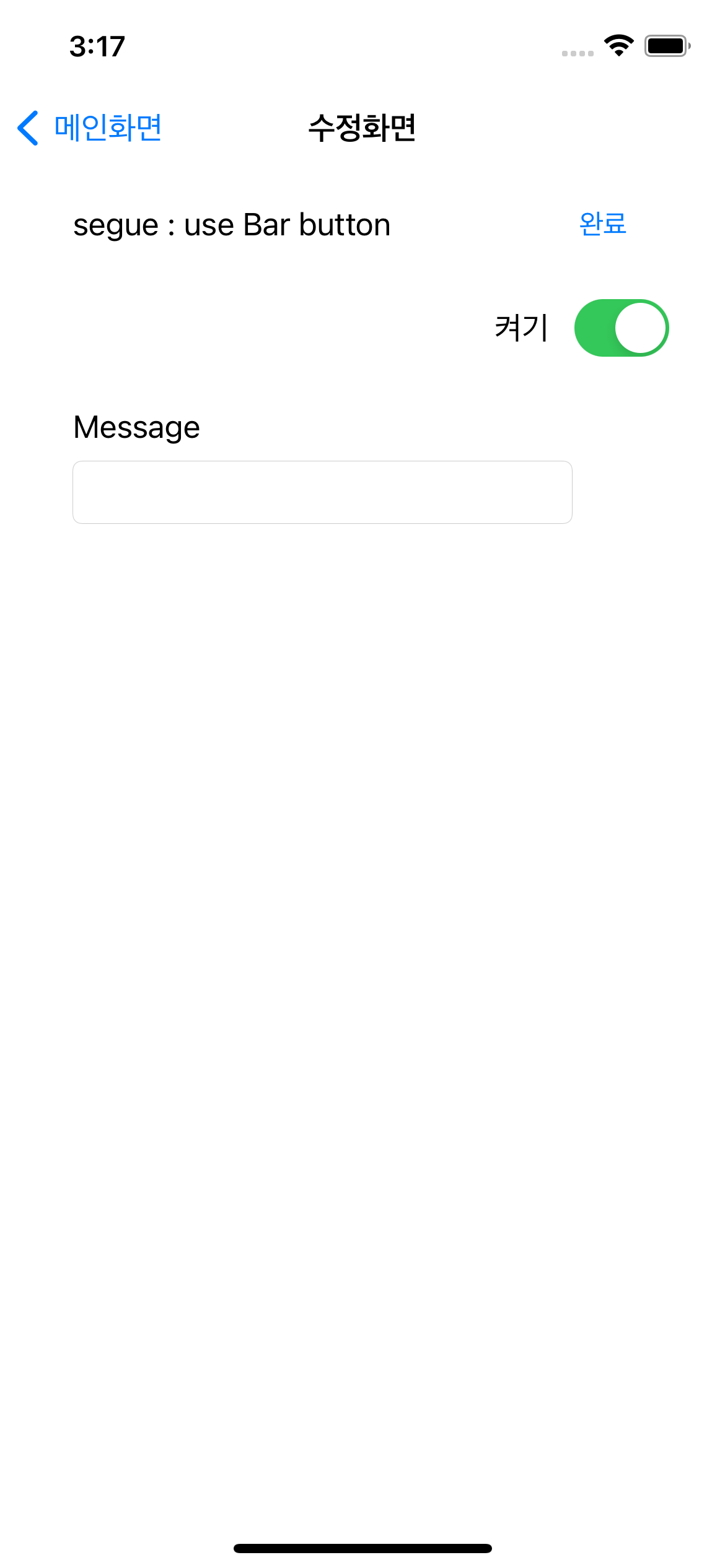
//
// ViewController.swift
// Navigation
//
// Created by BeomGeun Lee on 2021.
//
import UIKit
class ViewController: UIViewController, EditDelegate {
let imgOn = UIImage(named: "lamp_on.png")
let imgOff = UIImage(named: "lamp_off.png")
var isOn = true
@IBOutlet var txMessage: UITextField!
@IBOutlet var imgView: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
imgView.image = imgOn
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
let editViewController = segue.destination as! EditViewController
if segue.identifier == "editButton" {
editViewController.textWayValue = "segue : use button"
} else if segue.identifier == "editBarButton" {
editViewController.textWayValue = "segue : use Bar button"
}
editViewController.textMessage = txMessage.text!
editViewController.isOn = isOn
editViewController.delegate = self
}
func didMessageEditDone(_ controller: EditViewController, message: String) {
txMessage.text = message
}
func didImageOnOffDone(_ controller: EditViewController, isOn: Bool) {
if isOn {
imgView.image = imgOn
self.isOn = true
} else {
imgView.image = imgOff
self.isOn = false
}
}
}
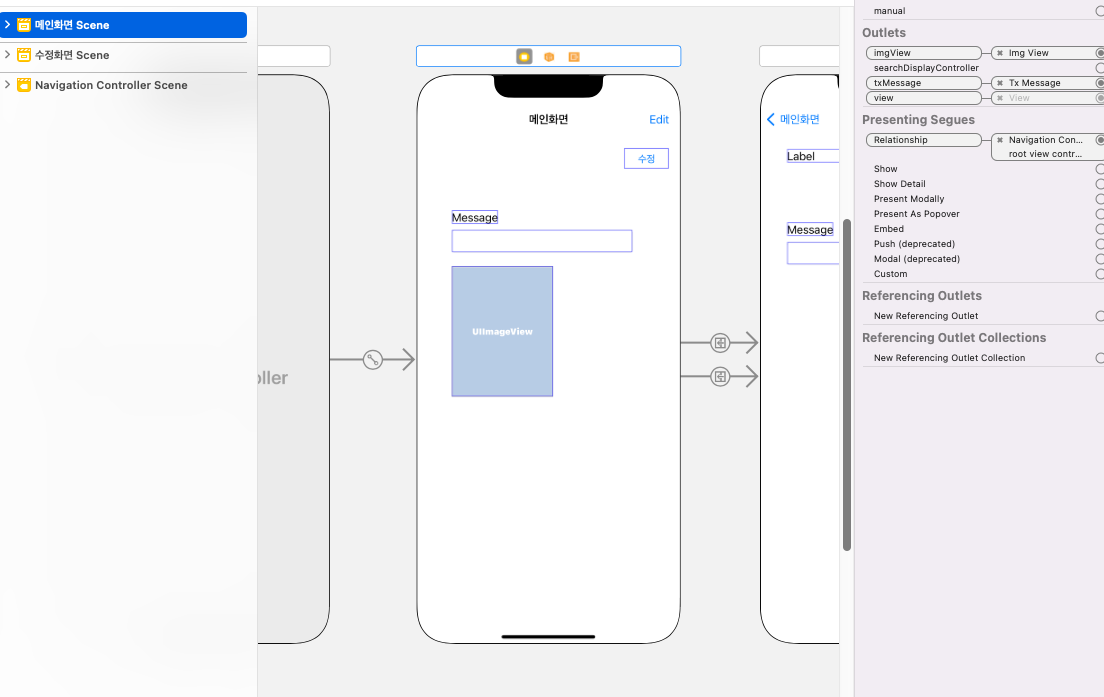
12강
(투두 리스트)테이블
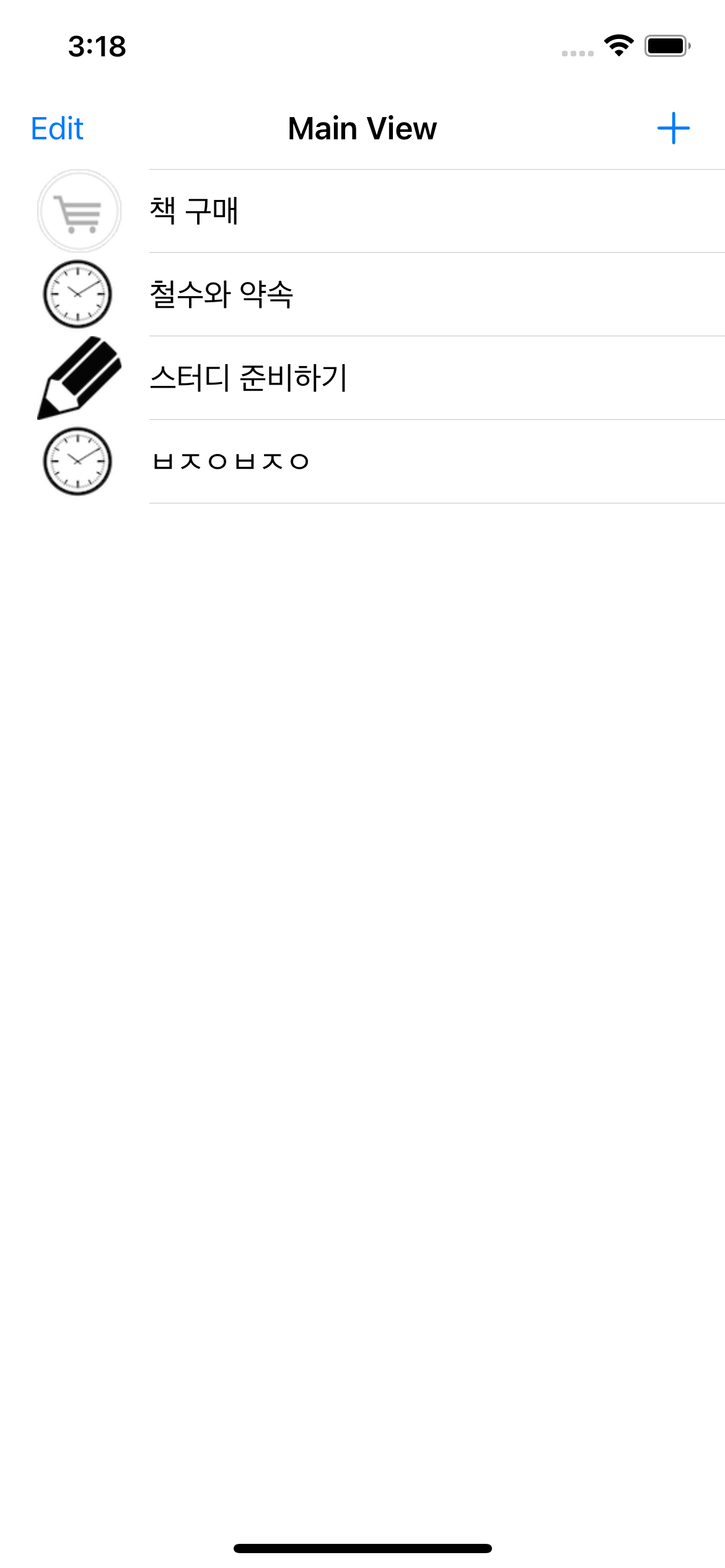
13강(오디오 출력)
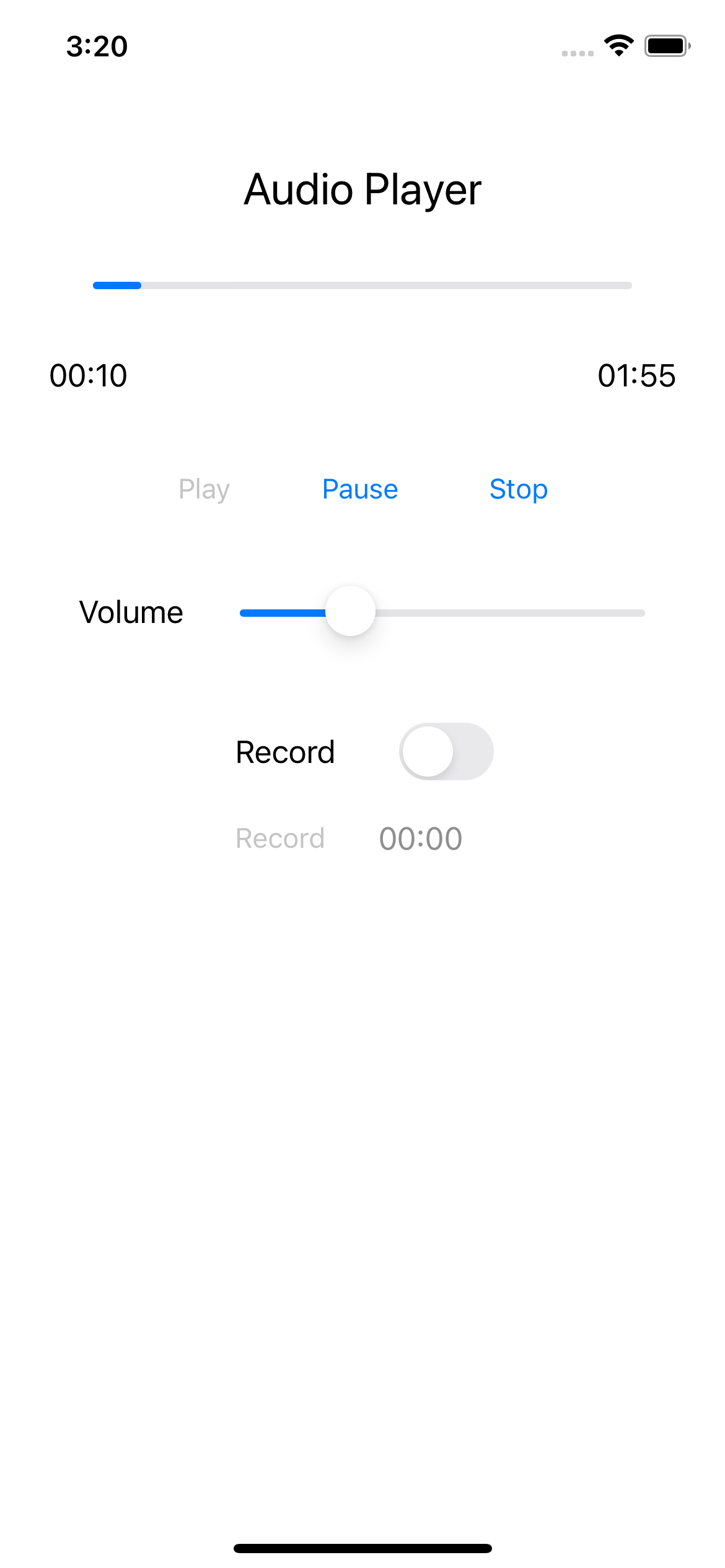
//
// ViewController.swift
// Audio
//
// Created by BeomGeun Lee on 2021.
//
import UIKit
import AVFoundation
class ViewController: UIViewController, AVAudioPlayerDelegate, AVAudioRecorderDelegate {
var audioPlayer : AVAudioPlayer!
var audioFile : URL!
let MAX_VOLUME : Float = 10.0
var progressTimer : Timer!
let timePlayerSelector:Selector = #selector(ViewController.updatePlayTime)
let timeRecordSelector:Selector = #selector(ViewController.updateRecordTime)
@IBOutlet var pvProgressPlay: UIProgressView!
@IBOutlet var lblCurrentTime: UILabel!
@IBOutlet var lblEndTime: UILabel!
@IBOutlet var btnPlay: UIButton!
@IBOutlet var btnPause: UIButton!
@IBOutlet var btnStop: UIButton!
@IBOutlet var slVolume: UISlider!
@IBOutlet var btnRecord: UIButton!
@IBOutlet var lblRecordTime: UILabel!
var audioRecorder : AVAudioRecorder!
var isRecordMode = false
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
selectAudioFile()
if !isRecordMode {
initPlay()
btnRecord.isEnabled = false
lblRecordTime.isEnabled = false
} else {
initRecord()
}
}
func selectAudioFile() {
if !isRecordMode {
audioFile = Bundle.main.url(forResource: "Sicilian_Breeze", withExtension: "mp3")
} else {
let documentDirectory = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)[0]
audioFile = documentDirectory.appendingPathComponent("recordFile.m4a")
}
}
func initRecord() {
let recordSettings = [
AVFormatIDKey : NSNumber(value: kAudioFormatAppleLossless as UInt32),
AVEncoderAudioQualityKey : AVAudioQuality.max.rawValue,
AVEncoderBitRateKey : 320000,
AVNumberOfChannelsKey : 2,
AVSampleRateKey : 44100.0] as [String : Any]
do {
audioRecorder = try AVAudioRecorder(url: audioFile, settings: recordSettings)
} catch let error as NSError {
print("Error-initRecord : \(error)")
}
audioRecorder.delegate = self
slVolume.value = 1.0
audioPlayer.volume = slVolume.value
lblEndTime.text = convertNSTimeInterval2String(0)
lblCurrentTime.text = convertNSTimeInterval2String(0)
setPlayButtons(false, pause: false, stop: false)
let session = AVAudioSession.sharedInstance()
do {
try AVAudioSession.sharedInstance().setCategory(.playAndRecord, mode: .default)
try AVAudioSession.sharedInstance().setActive(true)
} catch let error as NSError {
print(" Error-setCategory : \(error)")
}
do {
try session.setActive(true)
} catch let error as NSError {
print(" Error-setActive : \(error)")
}
}
func initPlay() {
do {
audioPlayer = try AVAudioPlayer(contentsOf: audioFile)
} catch let error as NSError {
print("Error-initPlay : \(error)")
}
slVolume.maximumValue = MAX_VOLUME
slVolume.value = 1.0
pvProgressPlay.progress = 0
audioPlayer.delegate = self
audioPlayer.prepareToPlay()
audioPlayer.volume = slVolume.value
lblEndTime.text = convertNSTimeInterval2String(audioPlayer.duration)
lblCurrentTime.text = convertNSTimeInterval2String(0)
setPlayButtons(true, pause: false, stop: false)
}
func setPlayButtons(_ play:Bool, pause:Bool, stop:Bool) {
btnPlay.isEnabled = play
btnPause.isEnabled = pause
btnStop.isEnabled = stop
}
func convertNSTimeInterval2String(_ time:TimeInterval) -> String {
let min = Int(time/60)
let sec = Int(time.truncatingRemainder(dividingBy: 60))
let strTime = String(format: "%02d:%02d", min, sec)
return strTime
}
@IBAction func btnPlayAudio(_ sender: UIButton) {
audioPlayer.play()
setPlayButtons(false, pause: true, stop: true)
progressTimer = Timer.scheduledTimer(timeInterval: 0.1, target: self, selector: timePlayerSelector, userInfo: nil, repeats: true)
}
@objc func updatePlayTime() {
lblCurrentTime.text = convertNSTimeInterval2String(audioPlayer.currentTime)
pvProgressPlay.progress = Float(audioPlayer.currentTime/audioPlayer.duration)
}
@IBAction func btnPauseAudio(_ sender: UIButton) {
audioPlayer.pause()
setPlayButtons(true, pause: false, stop: true)
}
@IBAction func btnStopAudio(_ sender: UIButton) {
audioPlayer.stop()
audioPlayer.currentTime = 0
lblCurrentTime.text = convertNSTimeInterval2String(0)
setPlayButtons(true, pause: false, stop: false)
progressTimer.invalidate()
}
@IBAction func slChangeVolume(_ sender: UISlider) {
audioPlayer.volume = slVolume.value
}
func audioPlayerDidFinishPlaying(_ player: AVAudioPlayer, successfully flag: Bool) {
progressTimer.invalidate()
setPlayButtons(true, pause: false, stop: false)
}
@IBAction func swRecordMode(_ sender: UISwitch) {
if sender.isOn {
audioPlayer.stop()
audioPlayer.currentTime=0
lblRecordTime!.text = convertNSTimeInterval2String(0)
isRecordMode = true
btnRecord.isEnabled = true
lblRecordTime.isEnabled = true
} else {
isRecordMode = false
btnRecord.isEnabled = false
lblRecordTime.isEnabled = false
lblRecordTime.text = convertNSTimeInterval2String(0)
}
selectAudioFile()
if !isRecordMode {
initPlay()
} else {
initRecord()
}
}
@IBAction func btnRecord(_ sender: UIButton) {
if (sender as AnyObject).titleLabel?.text == "Record" {
audioRecorder.record()
(sender as AnyObject).setTitle("Stop", for: UIControl.State())
progressTimer = Timer.scheduledTimer(timeInterval: 0.1, target: self, selector: timeRecordSelector, userInfo: nil, repeats: true)
} else {
audioRecorder.stop()
progressTimer.invalidate()
(sender as AnyObject).setTitle("Record", for: UIControl.State())
btnPlay.isEnabled = true
initPlay()
}
}
@objc func updateRecordTime() {
lblRecordTime.text = convertNSTimeInterval2String(audioRecorder.currentTime)
}
}
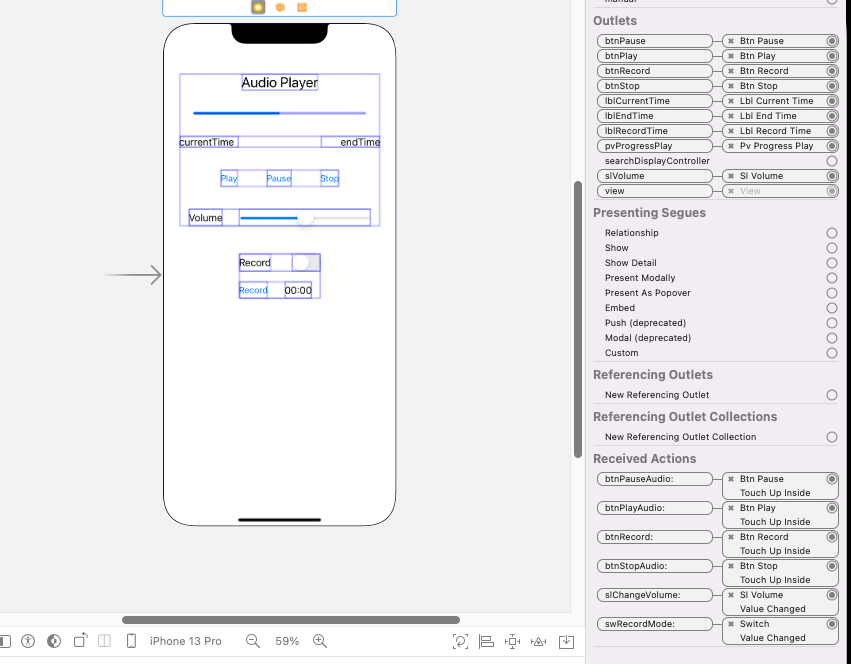
14강
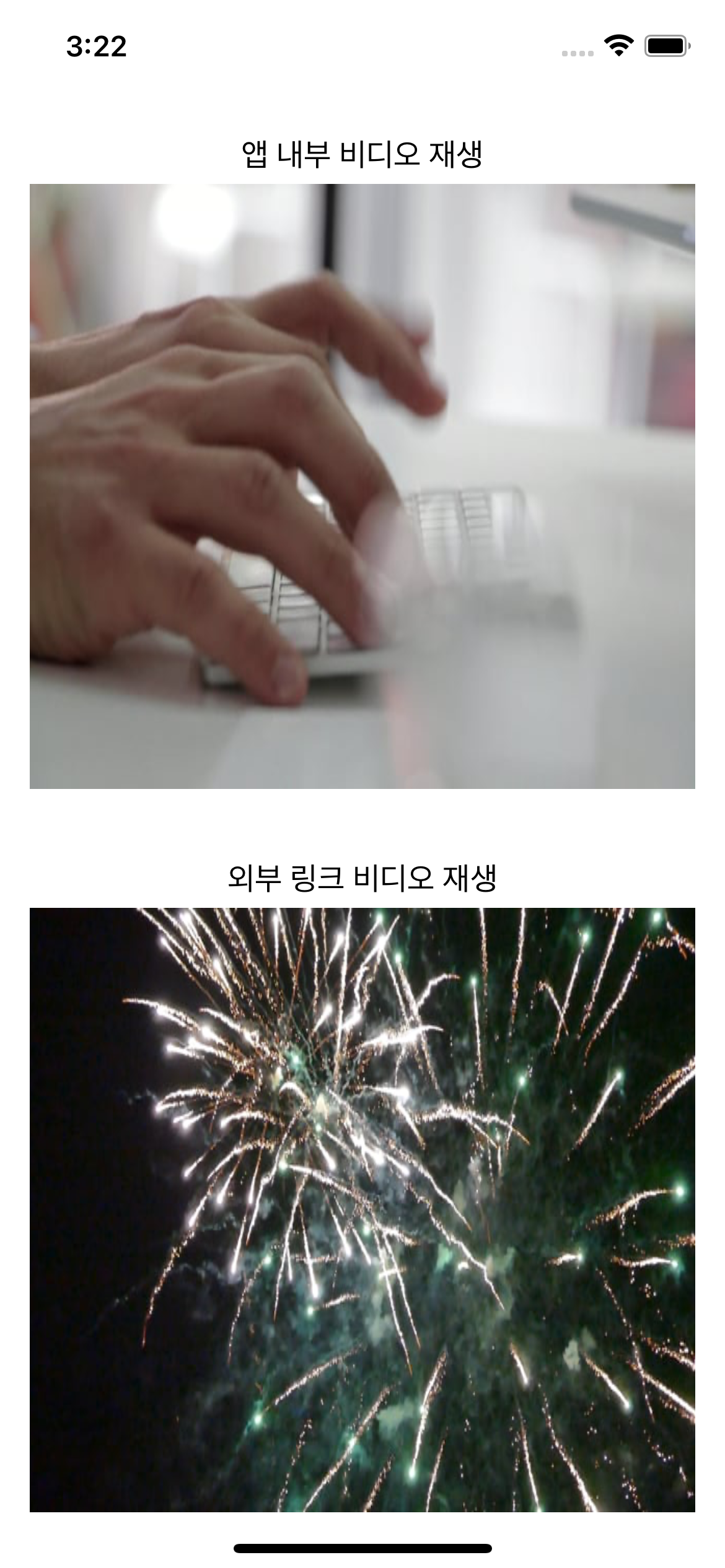
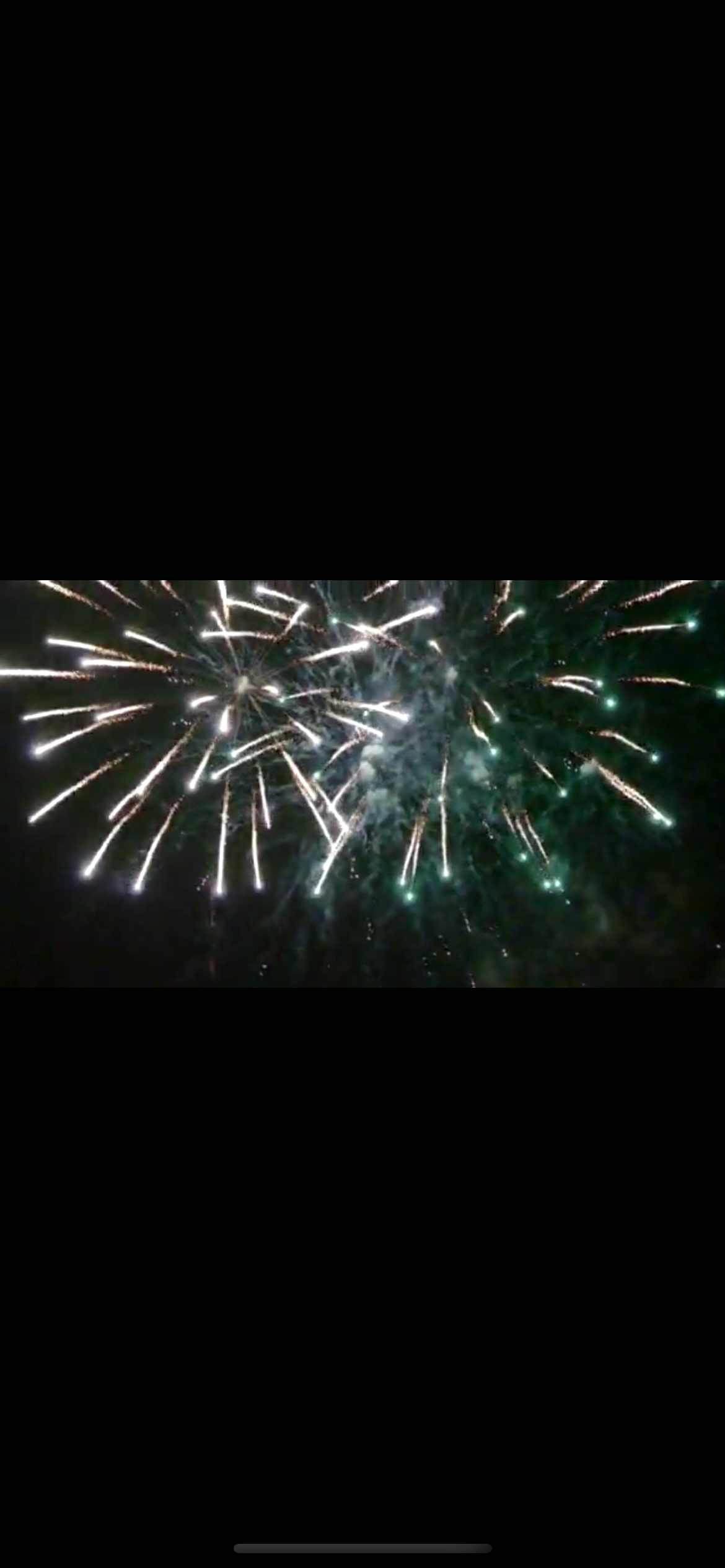
//
// ViewController.swift
// MoviePlayer
//
// Created by Ho-Jeong Song on 2021/11/26.
//
import UIKit
import AVKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnPlayInternalMovie(_ sender: UIButton) {
// 내부 파일 mp4
let filePath:String? = Bundle.main.path(forResource: "FastTyping", ofType: "mp4")
let url = NSURL(fileURLWithPath: filePath!)
playVideo(url: url)
}
@IBAction func btnPlayerExternalMovie(_ sender: UIButton) {
// 외부 파일 mp4
let url = NSURL(string: "https://dl.dropboxusercontent.com/s/e38auz050w2mvud/Fireworks.mp4")!
playVideo(url: url)
}
private func playVideo(url: NSURL) {
let playerController = AVPlayerViewController()
let player = AVPlayer(url: url as URL)
playerController.player = player
self.present(playerController, animated: true) {
player.play()
}
}
}
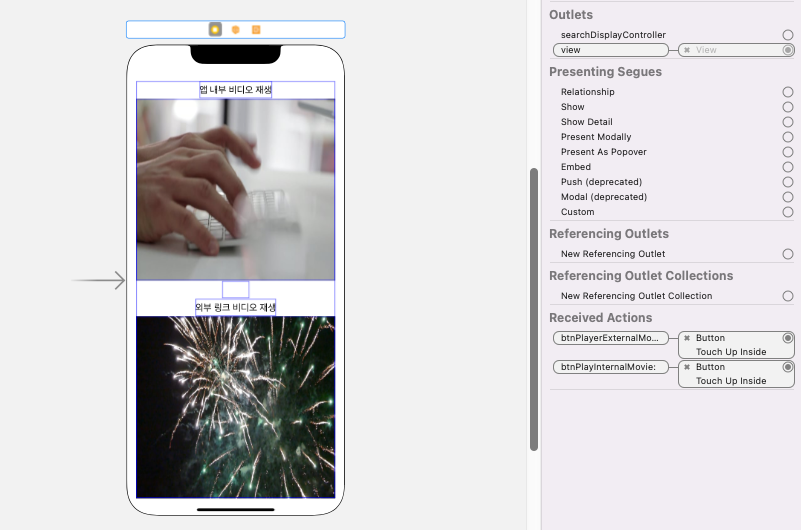
15강
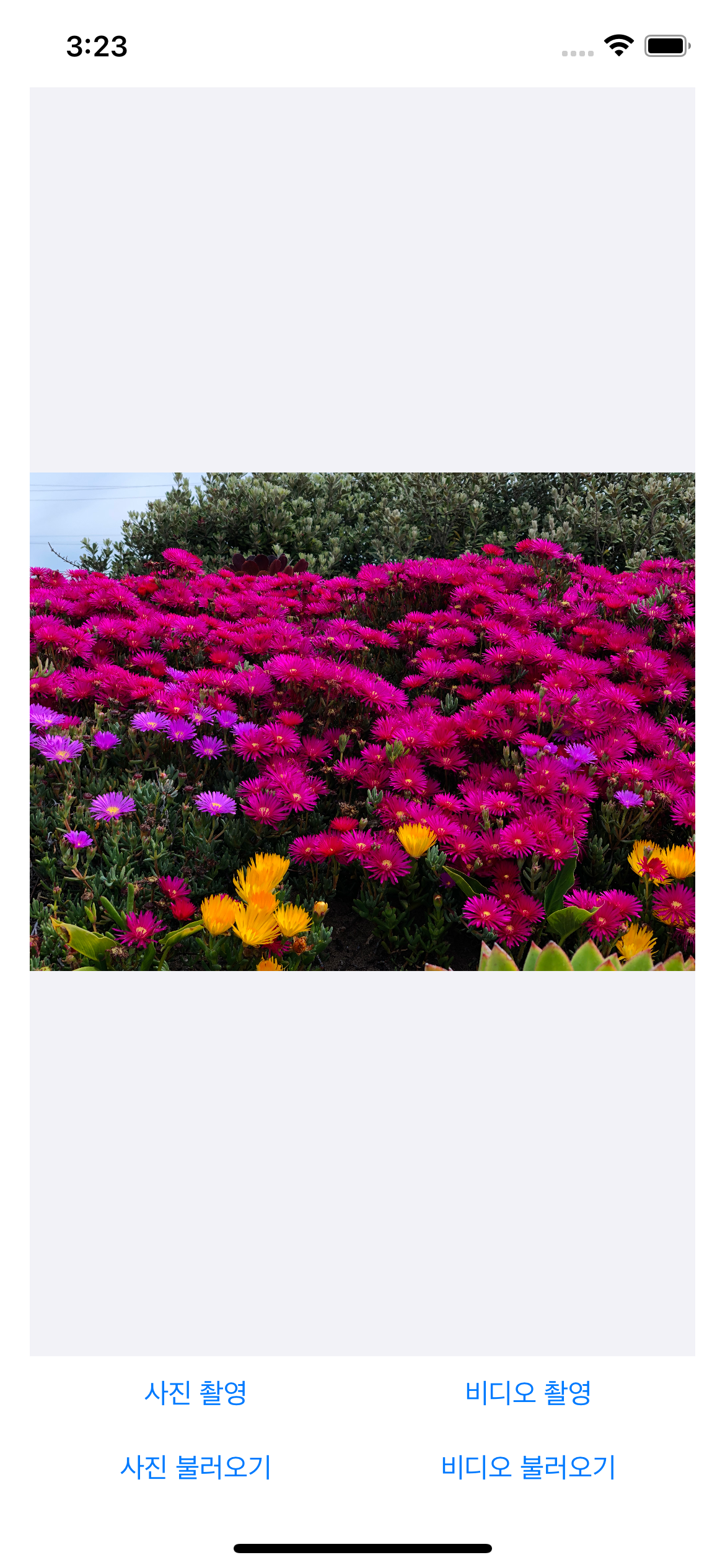
//
// ViewController.swift
// CameraPhotoLibrary
//
// Created by Ho-Jeong Song on 2021/11/27.
//
import UIKit
import MobileCoreServices
class ViewController: UIViewController, UINavigationControllerDelegate, UIImagePickerControllerDelegate {
@IBOutlet var imgView: UIImageView!
let imagePicker: UIImagePickerController! = UIImagePickerController()
var captureImage: UIImage!
var videoURL: URL!
var flagImageSave = false
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnCaptureImageFromCamera(_ sender: UIButton) {
if (UIImagePickerController.isSourceTypeAvailable(.camera)) {
flagImageSave = true
imagePicker.delegate = self
imagePicker.sourceType = .camera
imagePicker.mediaTypes = ["public.image"]
imagePicker.allowsEditing = false
present(imagePicker, animated: true, completion: nil)
}
else {
myAlert("Camera inaccessable", message: "Application cannot access the camera.")
}
}
@IBAction func btnLoadImageFromLibrary(_ sender: UIButton) {
if (UIImagePickerController.isSourceTypeAvailable(.photoLibrary)) {
flagImageSave = false
imagePicker.delegate = self
imagePicker.sourceType = .photoLibrary
imagePicker.mediaTypes = ["public.image"]
imagePicker.allowsEditing = true
present(imagePicker, animated: true, completion: nil)
}
else {
myAlert("Photo album inaccessable", message: "Application cannot access the photo album.")
}
}
@IBAction func btnRecordVideoFromCamera(_ sender: UIButton) {
if (UIImagePickerController.isSourceTypeAvailable(.camera)) {
flagImageSave = true
imagePicker.delegate = self
imagePicker.sourceType = .camera
imagePicker.mediaTypes = ["public.movie"]
imagePicker.allowsEditing = false
present(imagePicker, animated: true, completion: nil)
}
else {
myAlert("Camera inaccessable", message: "Application cannot access the camera.")
}
}
@IBAction func btnLoadVideoFromLibrary(_ sender: UIButton) {
if (UIImagePickerController.isSourceTypeAvailable(.photoLibrary)) {
flagImageSave = false
imagePicker.delegate = self
imagePicker.sourceType = .photoLibrary
imagePicker.mediaTypes = ["public.movie"]
imagePicker.allowsEditing = false
present(imagePicker, animated: true, completion: nil)
}
else {
myAlert("Photo album inaccessable", message: "Application cannot access the photo album.")
}
}
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [UIImagePickerController.InfoKey : Any]) {
let mediaType = info[UIImagePickerController.InfoKey.mediaType] as! NSString
if mediaType.isEqual(to: "public.image" as String) {
captureImage = info[UIImagePickerController.InfoKey.originalImage] as? UIImage
if flagImageSave {
UIImageWriteToSavedPhotosAlbum(captureImage, self, nil, nil)
}
imgView.image = captureImage
}
else if mediaType.isEqual(to: "public.movie" as String) {
if flagImageSave {
videoURL = (info[UIImagePickerController.InfoKey.mediaURL] as! URL)
UISaveVideoAtPathToSavedPhotosAlbum(videoURL.relativePath, self, nil, nil)
}
}
self.dismiss(animated: true, completion: nil)
}
func imagePickerControllerDidCancel(_ picker: UIImagePickerController) {
self.dismiss(animated: true, completion: nil)
}
func myAlert(_ title: String, message: String) {
let alert = UIAlertController(title: title, message: message, preferredStyle: UIAlertController.Style.alert)
let action = UIAlertAction(title: "Ok", style: UIAlertAction.Style.default, handler: nil)
alert.addAction(action)
self.present(alert, animated: true, completion: nil)
}
}

16강
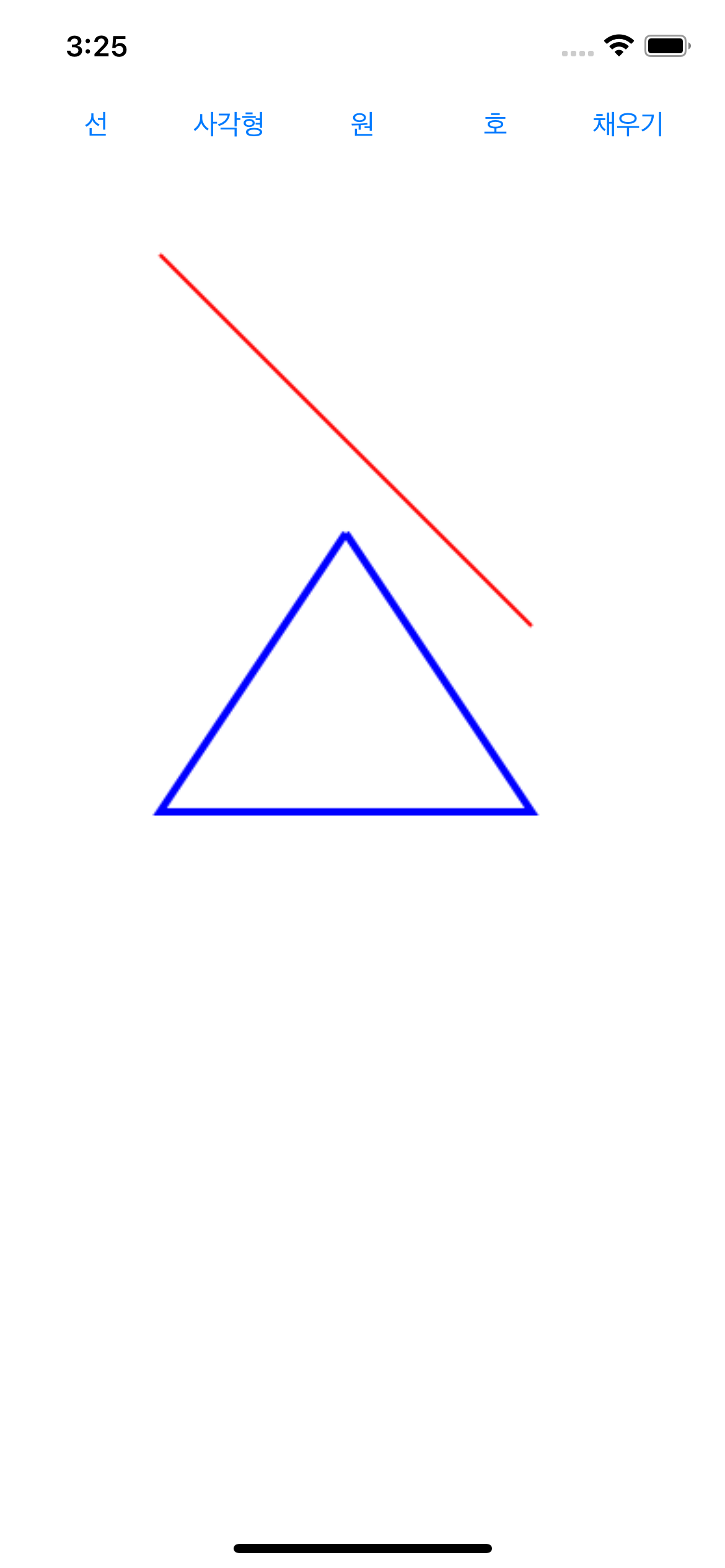
//
// ViewController.swift
// DrawGraphics
//
// Created by Ho-Jeong Song on 2021/12/01.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var imgView: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
@IBAction func btnDrawLine(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Line
context.setLineWidth(2.0)
context.setStrokeColor(UIColor.red.cgColor)
context.move(to: CGPoint(x: 70, y: 50))
context.addLine(to: CGPoint(x: 270, y: 250))
context.strokePath()
// Draw Triangle
context.setLineWidth(4.0)
context.setStrokeColor(UIColor.blue.cgColor)
context.move(to: CGPoint(x: 170, y: 200))
context.addLine(to: CGPoint(x: 270, y: 350))
context.addLine(to: CGPoint(x: 70, y: 350))
context.addLine(to: CGPoint(x: 170, y: 200))
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
@IBAction func btnDrawRectangle(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Rectangle
context.setLineWidth(2.0)
context.setStrokeColor(UIColor.red.cgColor)
context.addRect(CGRect(x: 70, y: 100, width: 200, height: 200))
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
@IBAction func btnDrawCircle(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Ellipse
context.setLineWidth(2.0)
context.setStrokeColor(UIColor.red.cgColor)
context.addEllipse(in: CGRect(x: 70, y: 50, width: 200, height: 100))
context.strokePath()
// Draw Circle
context.setLineWidth(5.0)
context.setStrokeColor(UIColor.green.cgColor)
context.addEllipse(in: CGRect(x: 70, y: 200, width: 200, height: 200))
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
@IBAction func btnDrawArc(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Arc
context.setLineWidth(5.0)
context.setStrokeColor(UIColor.red.cgColor)
context.move(to: CGPoint(x: 100, y: 50))
context.addArc(tangent1End: CGPoint(x: 250, y:50), tangent2End: CGPoint(x:250, y:200), radius: CGFloat(50))
context.addLine(to: CGPoint(x: 250, y: 200))
context.move(to: CGPoint(x: 100, y: 250))
context.addArc(tangent1End: CGPoint(x: 270, y:250), tangent2End: CGPoint(x:100, y:400), radius: CGFloat(20))
context.addLine(to: CGPoint(x: 100, y: 400))
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
@IBAction func btnDrawFill(_ sender: UIButton) {
UIGraphicsBeginImageContext(imgView.frame.size)
let context = UIGraphicsGetCurrentContext()!
// Draw Rectangle
context.setLineWidth(1.0)
context.setStrokeColor(UIColor.red.cgColor)
context.setFillColor(UIColor.red.cgColor)
let rectangel = CGRect(x: 70, y: 50, width: 200, height: 100)
context.addRect(rectangel)
context.fill(rectangel)
context.strokePath()
// Draw Circle
context.setLineWidth(1.0)
context.setStrokeColor(UIColor.blue.cgColor)
context.setFillColor(UIColor.blue.cgColor)
let circle = CGRect(x: 70, y: 200, width: 200, height: 100)
context.addEllipse(in: circle)
context.fillEllipse(in: circle)
context.strokePath()
// Draw Triangle
context.setLineWidth(1.0)
context.setStrokeColor(UIColor.green.cgColor)
context.setFillColor(UIColor.green.cgColor)
context.move(to: CGPoint(x: 170, y: 350))
context.addLine(to: CGPoint(x: 270, y: 450))
context.addLine(to: CGPoint(x: 70, y: 450))
context.addLine(to: CGPoint(x: 170, y: 350))
context.fillPath()
context.strokePath()
imgView.image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
}
}
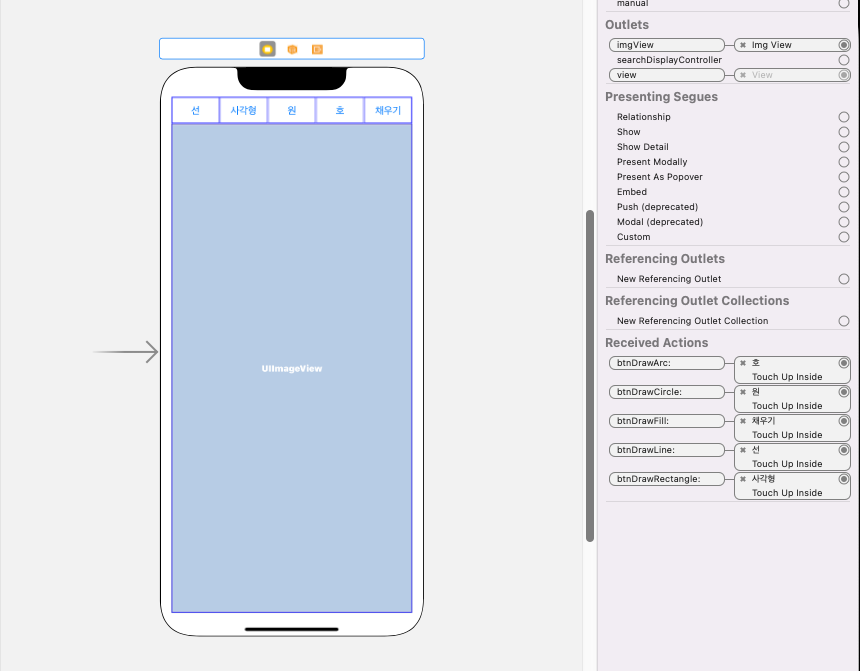
17강
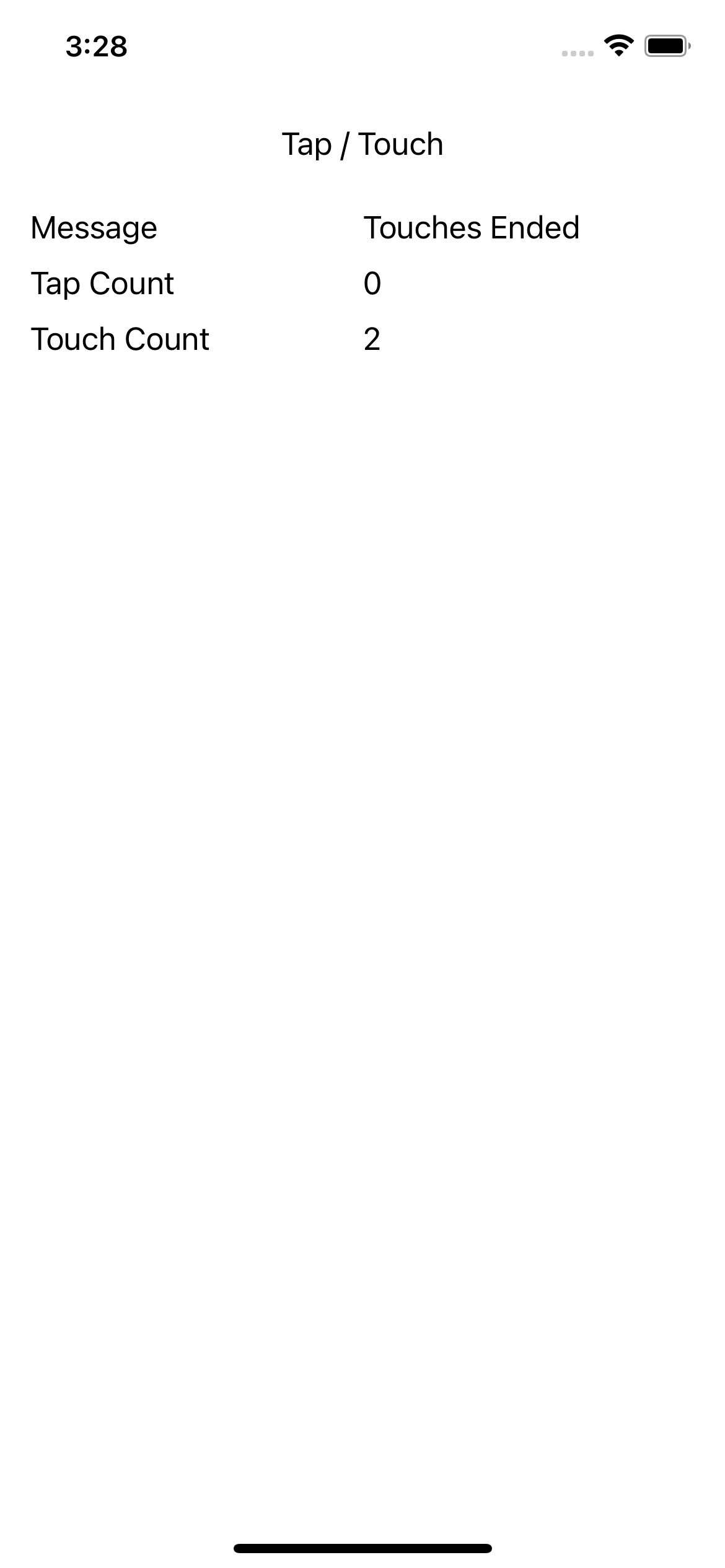
//
// ViewController.swift
// TapTouch
//
// Created by Ho-Jeong Song on 2021/12/01.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var txtMessage: UILabel!
@IBOutlet var txtTapCount: UILabel!
@IBOutlet var txtTouchCount: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
let touch = touches.first! as UITouch
txtMessage.text = "Touches Began"
txtTapCount.text = String(touch.tapCount)
txtTouchCount.text = String(touches.count)
}
override func touchesMoved(_ touches: Set<UITouch>, with event: UIEvent?) {
let touch = touches.first! as UITouch
txtMessage.text = "Touches Moved"
txtTapCount.text = String(touch.tapCount)
txtTouchCount.text = String(touches.count)
}
override func touchesEnded(_ touches: Set<UITouch>, with event: UIEvent?) {
let touch = touches.first! as UITouch
txtMessage.text = "Touches Ended"
txtTapCount.text = String(touch.tapCount)
txtTouchCount.text = String(touches.count)
}
}

18강
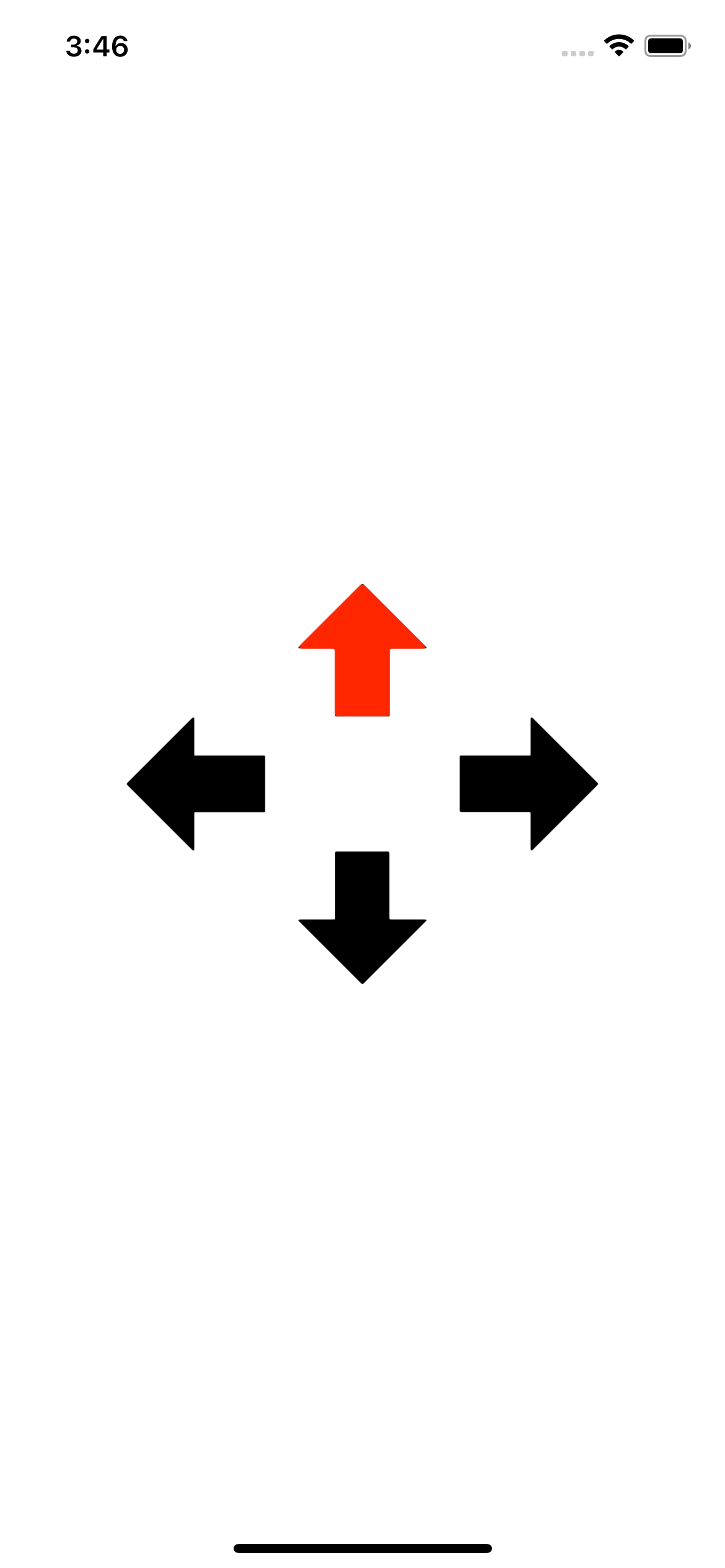
//
// ViewController.swift
// SwipeGesture
//
// Created by Ho-Jeong Song on 2021/12/02.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var imgViewUp: UIImageView!
@IBOutlet var imgViewDown: UIImageView!
@IBOutlet var imgViewLeft: UIImageView!
@IBOutlet var imgViewRight: UIImageView!
var imgLeft = [UIImage]()
var imgRight = [UIImage]()
var imgUp = [UIImage]()
var imgDown = [UIImage]()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
imgUp.append(UIImage(named: "arrow-up-black.png")!)
imgUp.append(UIImage(named: "arrow-up-red.png")!)
imgDown.append(UIImage(named: "arrow-down-black.png")!)
imgDown.append(UIImage(named: "arrow-down-red.png")!)
imgLeft.append(UIImage(named: "arrow-left-black.png")!)
imgLeft.append(UIImage(named: "arrow-left-red.png")!)
imgRight.append(UIImage(named: "arrow-right-black.png")!)
imgRight.append(UIImage(named: "arrow-right-red.png")!)
imgViewUp.image = imgUp[0]
imgViewDown.image = imgDown[0]
imgViewLeft.image = imgLeft[0]
imgViewRight.image = imgRight[0]
let swipeUp = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.respondToSwipeGesture(_:)))
swipeUp.direction = UISwipeGestureRecognizer.Direction.up
self.view.addGestureRecognizer(swipeUp)
let swipeDown = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.respondToSwipeGesture(_:)))
swipeDown.direction = UISwipeGestureRecognizer.Direction.down
self.view.addGestureRecognizer(swipeDown)
let swipeLeft = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.respondToSwipeGesture(_:)))
swipeLeft.direction = UISwipeGestureRecognizer.Direction.left
self.view.addGestureRecognizer(swipeLeft)
let swipeRight = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.respondToSwipeGesture(_:)))
swipeRight.direction = UISwipeGestureRecognizer.Direction.right
self.view.addGestureRecognizer(swipeRight)
}
@objc func respondToSwipeGesture(_ gesture: UIGestureRecognizer) {
if let swipeGesture = gesture as? UISwipeGestureRecognizer {
imgViewUp.image = imgUp[0]
imgViewDown.image = imgDown[0]
imgViewLeft.image = imgLeft[0]
imgViewRight.image = imgRight[0]
switch swipeGesture.direction {
case UISwipeGestureRecognizer.Direction.up:
imgViewUp.image = imgUp[1]
case UISwipeGestureRecognizer.Direction.down:
imgViewDown.image = imgDown[1]
case UISwipeGestureRecognizer.Direction.left:
imgViewLeft.image = imgLeft[1]
case UISwipeGestureRecognizer.Direction.right:
imgViewRight.image = imgRight[1]
default:
break
}
}
}
}

19강

//
// ViewController.swift
// PinchGesture
//
// Created by Ho-Jeong Song on 2021/12/02.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet var txtPinch: UILabel!
var initialFontSize:CGFloat!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
let pinch = UIPinchGestureRecognizer(target: self, action: #selector(ViewController.doPinch(_:)))
self.view.addGestureRecognizer(pinch)
}
@objc func doPinch(_ pinch: UIPinchGestureRecognizer) {
if (pinch.state == UIGestureRecognizer.State.began) {
initialFontSize = txtPinch.font.pointSize
} else {
txtPinch.font = txtPinch.font.withSize(initialFontSize * pinch.scale)
}
}
}
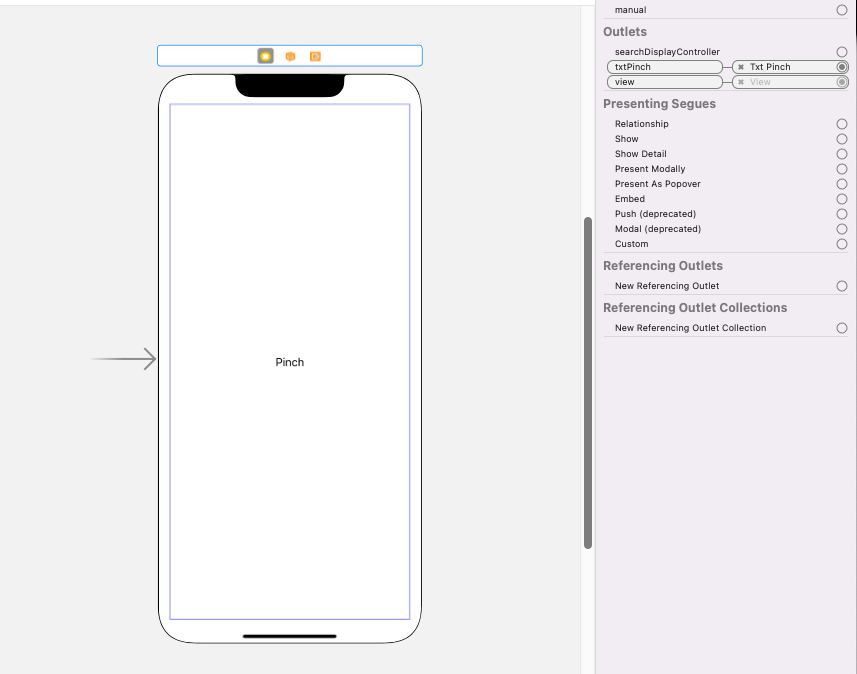
BMI 실습 소스
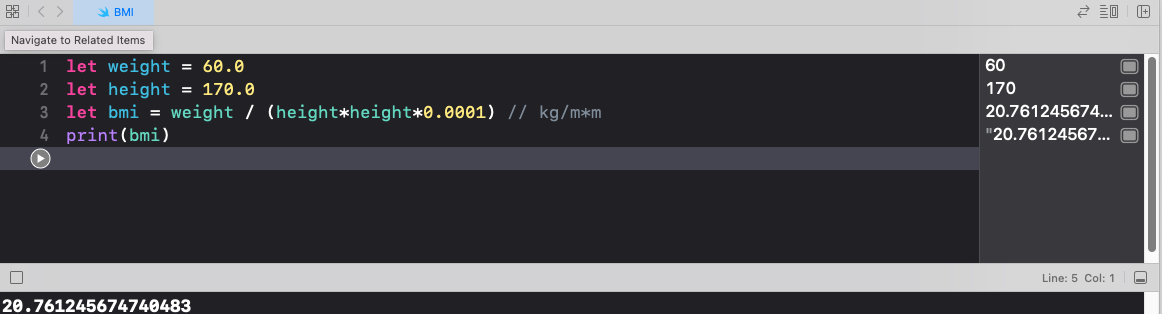
let weight = 60.0
let height = 170.0
let bmi = weight / (height*height*0.0001) // kg/m*m
print(bmi)
import Foundation
let weight = 60.0
let height = 170.0
let bmi = weight / (height*height*0.0001) // kg/m*m
let shortenedBmi = String(format: "%.2f", bmi) // 더블형을 문자열로 바꿔 저장
print(bmi, shortenedBmi)
//20.761245674740483 20.8
import Foundation
class BMI {
var weight : Double
var height : Double
init(weight:Double, height:Double){
self.height = height
self.weight = weight
}
func calcBMI() -> String {
let bmi=weight/(height*height*0.0001)// kg/m*m
let shortenedBmi = String(format: "%.1f", bmi)
var body = ""
if bmi >= 40{
body = "3단계 비만"
} else if bmi >= 30 && bmi < 40 {
body = "2단계 비만"
} else if bmi >= 25 && bmi < 30 {
body = "1단계 비만"
} else if bmi >= 18.5 && bmi < 25 {
body = "정상"
} else {
body = "저체중"
}
return "BMI:\(shortenedBmi), 판정:\(body)"
}
}
var han = BMI(weight:62.5, height:172.3)
print(han.calcBMI())
// BMI를 도출하는 클레스 생성자를 이용하요 값을 넣고 클레스 메소드를 이용하여 판정 결과 추출
//BMI:21.1, 판정:정상
728x90
'APP > iOS' 카테고리의 다른 글
MapKit with SwiftUI 기초부터 심화까지 1 : MapKit 다루기 기본 과정 (0) | 2024.01.08 |
---|---|
iOS23 SkillUpThon 2주차 정리 (0) | 2023.06.22 |
iOS23 SkillUpThon 1주차 정리 (0) | 2023.06.21 |
앱의 생명주기(Life Cycle) (0) | 2023.04.16 |
iOS 프로그래밍 : Event Driven Programming (0) | 2022.11.18 |